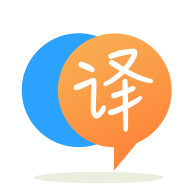
[英]If I perform an unwind segue (show), how can the mother view controller get the child's view controller?
[英]How to show a segue inside a child view controller?
我有一個主視圖控制器,在這個視圖控制器中,我將另一個視圖控制器顯示為它的子視圖控制器。 顯示子視圖控制器的代碼如下所示。 self.currentController 是位於主控制器內的子控制器。
self.addChildViewController(self.currentController!)
self.currentController?.view.frame = self.operationView.bounds
self.currentController?.view.layoutIfNeeded()
self.operationView.addSubview((self.currentController?.view!)!)
self.setNeedsStatusBarAppearanceUpdate()
現在我想使用下面的代碼在子視圖控制器內執行一個顯示轉場(另一個控制器,我們稱之為ThirdController):
performSegueWithIdentifier("ShowSegue", sender: nil)
這樣做時,ThirdController 將填滿全屏。 我想要做的是在子控制器位置顯示第三個控制器。 我怎樣才能做到這一點?
好的,對不起,我不知道如何用 Swift 編寫答案。 因此,我將向您展示我的解決方案是如何在 Objective-C 中完成的。
加載第一個子視圖的代碼:
- (void)loadASubview
{
subview = [self.storyboard instantiateViewControllerWithIdentifier:@"FirstView"];
[self addChildViewController:subview];
[self.view addSubview:subview.view];
[subview didMoveToParentViewController:self];
[subview.view setFrame:self.view.bounds];
}
卸載子視圖的代碼:
- (void)unloadASubview
{
[subview willMoveToParentViewController:nil];
[subview.view removeFromSuperview];
[subview removeFromParentViewController];
}
最初,當我需要加載子視圖 A 時,我將簡單地調用loadASubview 。 之后,如果我需要加載另一個子視圖,我會在加載新的子視圖之前通過調用 unloadASubview 來卸載我之前加載的子視圖。
請注意,函數內部的“subview”變量是在外部聲明的。
我希望這能幫到您。
斯威夫特 4
func loadASubView(){
subview = self.storyboard?.instantiateViewController(withIdentifier: "childviewstoryboardid")
self.addChildViewController(subview!)
self.containerView.addSubview(subview!.view)
subview?.didMove(toParentViewController: self)
subview?.view.frame = self.containerView.frame
}
func unloadASubview(){
subview?.willMove(toParentViewController: nil)
subview?.view.removeFromSuperview()
subview?.removeFromParentViewController()
}
這就是我對 autoLayout 的決心。 這是最好的方法,無需任何額外修改。
在 Children View Controller 中,您通常可以使用“show(vc: UIViewController, sender: Any?)”這個函數來顯示一個視圖控制器(如果有導航控制器,它會推送一個新的控制器,也不展示它)
斯威夫特 4.2
func setChild() {
let vc = UIStoryboard(name: "YOUR_STORYBOARD_NAME", bundle: nil).instantiateViewController(withIdentifier: "YOUR_CHILD_ID")
self.addChild(vc)
// need to set translatesAutoresizingMaskIntoConstraints false to enable auto layout
vc.view.translatesAutoresizingMaskIntoConstraints = false
/// add subview to parent
view.addSubview(vc.view)
/// send subview to back for show other view in parent
view.sendSubviewToBack(vc.view)
/// call did move to parent to show current children
vc.didMove(toParent: self)
/// <---create auto layout with VFL--->
let horiConstraints = NSLayoutConstraint.constraints(withVisualFormat: "H:|-0-[view]-0-|",
options: .alignAllCenterY,
metrics: nil,
views: ["view" : vc.view])
let verticalConstraints = NSLayoutConstraint.constraints(withVisualFormat: "V:|-0-[view]-0-|",
options: .alignAllCenterX,
metrics: nil,
views: ["view" : vc.view])
view.addConstraints(horiConstraints)
view.addConstraints(verticalConstraints)
view.layoutIfNeeded()
}
之后你可以刪除第一個孩子
func removeFirstChild() {
children.first?.willMove(toParent: nil)
children.first?.view.removeFromSuperview()
children.first?.didMove(toParent: nil)
}
或從父級中刪除所有子級
func removeAllChild() {
for child in children {
child.willMove(toParent: nil)
child.view.removeFromSuperview()
child.didMove(toParent: nil)
}
}
對象-C
- (void)setChild {
UIViewController *vc = [[UIStoryboard storyboardWithName:@"YOUR_STORYBOARD_NAME" bundle:nil] instantiateViewControllerWithIdentifier:@"YOUR_CHILD_ID"];
[self addChildViewController:vc];
// need to set translatesAutoresizingMaskIntoConstraints false to enable auto layout
vc.view.translatesAutoresizingMaskIntoConstraints = NO;
/// add subview to parent
[self.view addSubview:vc.view];
/// send subview to back for show other view in parent
[self.view sendSubviewToBack:vc.view];
/// call did move to parent to show current children
[vc didMoveToParentViewController:self];
/// <---create auto layout with VFL--->
NSArray<__kindof NSLayoutConstraint *> *horiConstraints;
horiConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"H:|-0-[view]-0-|"options:NSLayoutAttributeCenterY
metrics:nil
views:@{@"view": vc.view}];
NSArray<__kindof NSLayoutConstraint *> *verticalConstraints;
verticalConstraints = [NSLayoutConstraint constraintsWithVisualFormat:@"V:|-0-[view]-0-|"
options:NSLayoutAttributeCenterX
metrics:nil
views:@{@"view": vc.view}];
[self.view addConstraints:horiConstraints];
[self.view addConstraints:verticalConstraints];
[self.view layoutIfNeeded];
}
之后你可以刪除第一個孩子
- (void)removeFirstChild {
UIViewController *child = self.childViewControllers.firstObject;
[child willMoveToParentViewController:nil];
[child.view removeFromSuperview];
[child didMoveToParentViewController:nil];
}
或從父級中刪除所有子級
- (void)removeAllChild {
for (UIViewController* child in self.childViewControllers) {
[child willMoveToParentViewController:nil];
[child.view removeFromSuperview];
[child didMoveToParentViewController:nil];
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.