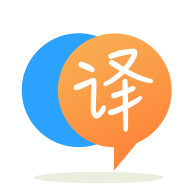
[英]How to wait for a thread to do something before continuing on another thread in Java?
[英]Trying to write a class that allows to do something before the Thread starts and after the Thread finishes
我正在嘗試編寫一個Utility類,該類有助於在單獨的線程上執行任務,提供在任務開始之前執行某些操作以及在任務結束之后執行某些操作的能力。
類似於android的AsyncTask
這是此類。
class MySync
{
public void preExecute() {}
public void executeInBackground() {}
public void postExecute() {}
public final void execute()
{
threadExecute.start();
}
private final Thread threadExecute = new Thread()
{
@Override
public void run()
{
try
{
MySync.this.preExecute();
MySync.this.executeInBackground();
MySync.this.postExecute();
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
};
}
這是應該使用的此類。 類的使用者將按要求覆蓋方法。
class RegisterStudent extends MySync
{
@Override
public void preExecute()
{
System.out.println("Validating Student details. Please wait...");
try
{
Thread.sleep(2000);
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
@Override
public void executeInBackground()
{
System.out.println("Storing student details into Database on Server. Please wait...");
try
{
Thread.sleep(4000);
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
@Override
public void postExecute()
{
System.out.println("Student details saved Successfully.");
}
}
最后開始任務:
public class AsyncDemo
{
public static void main(String... args)
{
new RegisterStudent().execute();
}
}
似乎工作正常。 我的問題是,這是否是實現標題中提到的目標的正確方法? 關於如何最好地實施的任何建議?
這樣做的壞處是您強迫用戶擴展您的課程。 在Java中,您只能擴展1個類。 因此,框架不應該把它剝奪。
而是使用一個接口:
public interface AsyncTask {
public default void preExecute() {}
public default void executeInBackground() {}
public default void postExecute() {}
}
並讓用戶將其傳遞給您的實用程序類:
class MySync
{
private AsyncTask task;
public MySync(AsyncTask task) {
this.task = task;
}
public final void execute()
{
threadExecute.start();
}
private final Thread threadExecute = new Thread()
{
@Override
public void run()
{
try
{
MySync.this.task.preExecute();
MySync.this.task.executeInBackground();
MySync.this.task.postExecute();
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
};
}
我不喜歡這種方法的事實是,您每次創建新的MySync
實例時MySync
創建一個新線程,如果您打算創建許多Object實例, MySync
該實例是不可伸縮的,而且創建一個新線程非常昂貴。線程,如果我是您,我將使用執行程序來限制分配給異步執行任務的線程總數,如果您只想使用一個線程,可以按以下方法執行:
ExecutorService executor = Executors.newFixedThreadPool(1);
我也將為這樣的代碼重新編寫代碼:
public abstract class MySync implements Runnable {
@Override
public final void run() {
try {
preExecute();
executeInBackground();
} finally {
postExecute();
}
}
protected abstract void preExecute();
protected abstract void executeInBackground();
protected abstract void postExecute();
}
這樣,您可以為所有實現定義整個邏輯。
然后,您可以像這樣提交任務:
executor.submit(new RegisterStudent());
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.