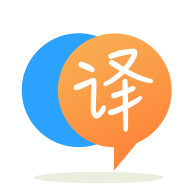
[英]How random.nextBytes(bytes) generates the bytes after we have seed
[英]How do I write bytes to a file based on a long with SecureRandom().nextBytes?
對於我的java類,我項目的一部分涉及從用戶那里獲取加密密鑰長度,並將其舍入到最接近的1024的倍數。該長度以長整數形式傳遞。 在我的方法中,我得到的很長,並且我得到了要寫入的文件路徑。 在示例中,我看到了此實現:
try (FileOutputStream out = new FileOutputStream(file)) {
byte[] bytes = new byte[1024];
new SecureRandom().nextBytes(bytes);
out.write(bytes);
}
但是,在哪里以及如何實現我的long變量? 我不能在字節[long]中加長。 我知道我需要根據教授使用SecureRandom()。nextBytes()。 任何幫助將不勝感激,因為這部分一直讓我發瘋。
這是我到目前為止所擁有的,但是我忍不住認為這不是我的教授想要完成的方式...
public void oneKeyGenerator(String keyPath, long keyLength) {
final long CONST_MULTIPLE = 1024;
try {
FileOutputStream out = new FileOutputStream(keyPath);
byte[] bytes = new byte[1024];
for(long x = 0; x < keyLength/CONST_MULTIPLE; x++) {
new SecureRandom().nextBytes(bytes);
out.write(bytes);
}
} catch(IOException e){
gui.fileException(e.getMessage());
}
}
您不必循環,只需分配所需大小的字節數組即可:
long roundNext(long v, long multiple) {
return ((v + multiple - 1) / multiple) * multiple;
}
public void oneKeyGenerator(String keyPath, long keyLength) {
final long CONST_MULTIPLE = 1024;
try {
FileOutputStream out = new FileOutputStream(keyPath);
byte[] bytes = new byte[(int) roundNext(keyLength, CONST_MULTIPLE)];
new SecureRandom().nextBytes(bytes);
out.write(bytes);
} catch(IOException e){
gui.fileException(e.getMessage());
}
}
希望能幫助到你。
您的要求似乎有些奇怪。 是否要求用戶輸入的密鑰長度必須存儲很長? 這將意味着用戶可以請求一個長度大於2,147,483,647的密鑰,因為這是int可以容納的最大值。 那將是巨大的,聽起來很荒謬。 您可能只能使用int而不是long。 20億字節大約需要2 GB的數據。
加密密鑰通常以位指定,但是仍然是260+ MB的數據。
您需要將其分解為單獨的問題來解決,並創建單獨的方法。
下面,我提出了一個解決方案,該解決方案實際上可以讓您編寫超級大密鑰,但是我認為您並不是真的想要這樣做,弄清楚它會更有趣。 您可能應該將keySize強制轉換為int,然后像在totoro的答案中一樣使用它。 這個答案有點瘋狂,但是它應該可以幫助您,甚至可以讓您重新思考自己在做什么。
static final long CONST_MULTIPLE = 1024;
private long getNearest1024Multiple(long value)
{
double divisor = value / (double)CONST_MULTIPLE;
int multiple = (int)Math.round(divisor);
if (multiple == 0)
{
multiple = 1;
}
return multiple * CONST_MULTIPLE;
}
private ByteArrayOutputStream generateLongEncryptionKey(long keySize) throws IOException
{
ByteArrayOutputStream baos = new ByteArrayOutputStream();
SecureRandom secureRandom = new SecureRandom();
while (keySize > 0)
{
if (keySize > Integer.MAX_VALUE)
{
// The keySize long actually has a super huge value in it, so grab a chunk at a time
byte[] randomBytes = new byte[Integer.MAX_VALUE];
secureRandom.nextBytes(randomBytes);
baos.write(randomBytes);
keySize -= Integer.MAX_VALUE;
}
else
{
// We grabbed the last chunk
byte[] randomBytes = new byte[(int)keySize];
secureRandom.nextBytes(randomBytes);
baos.write(randomBytes);
keySize -= keySize;
}
}
return baos;
}
private void generateAndSaveKey(String keyPath, long userInputKeyLength) throws IOException
{
long roundedKeyLength = getNearest1024Multiple(userInputKeyLength);
ByteArrayOutputStream baos = generateLongEncryptionKey(roundedKeyLength);
FileOutputStream fileOutputStream = new FileOutputStream(keyPath);
baos.writeTo(fileOutputStream);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.