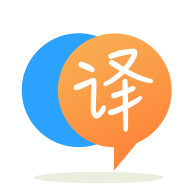
[英]How to use String.replace() to replace ALL occurrences of a pattern
[英]String.Replace only replaces first occurrence of matched string. How to replace *all* occurrences?
來自其他編程語言, String.replace()
通常替換所有匹配字符串。 但是, javascript/typescript並非如此。 我在網上找到了許多使用正則表達式的javascript解決方案。 由於特殊字符,我立即遇到了此解決方案的問題。 我懷疑有一種方法可以用正則表達式來糾正這個問題,但我不是正則表達式專家。 正如許多人在我之前所做的那樣,我創建了自己的方法。
也許有一些方法可以通過使用自定義StringBuilder()
類來提高性能。 我歡迎任何想法。
public static Replace = function (originalString: string, oldValue: string, newValue: string, ignoreCase: boolean = false) {
//
// if invalid data, return the original string
//
if ((originalString == null) || (oldValue == null) || (newValue == null) || (oldValue.length == 0) )
return (originalString);
//
// do text replacement
//
var dest = "";
var source: string = originalString;
if (ignoreCase)
{
source = source.toLocaleLowerCase();
oldValue = oldValue.toLowerCase();
}
//
// find first match
//
var StartPos = 0;
var EndPos = source.indexOf(oldValue, StartPos);
var Skip = (EndPos >= 0) ? EndPos - StartPos : source.length-StartPos;
//
// while we found a matched string
//
while (EndPos > -1) {
//
// copy original string skipped segment
//
if (Skip > 0) dest += originalString.substr(StartPos, Skip);
//
// copy new value
//
dest += newValue;
//
// skip over old value
//
StartPos = EndPos + oldValue.length;
//
// find next match
//
EndPos = source.indexOf(oldValue, StartPos);
Skip = (EndPos >= 0) ? EndPos - StartPos : source.length - StartPos;
}
//
// append the last skipped string segment from original string
//
if (Skip > 0) dest += originalString.substr(StartPos, Skip);
return dest;
}
為了向string
類添加對此方法的支持,我添加了以下代碼:
interface String { EZReplace(oldValue: string, newValue: string, ignorCase?: boolean): string; }
String.prototype.EZReplace = function (oldValue: string, newValue: string, ignorCase: boolean = false) {
return EZUtil.Replace(this, oldValue, newValue, ignorCase);}
....重新查看其他帖子后,我修改了代碼以使用正則表達式。 執行性能測試會很有趣。
public static Replace = function (originalString: string, oldValue: string, newValue: string, ignoreCase: boolean = false) {
//
// if invalid data, return the original string
//
if ((originalString == null) || (oldValue == null) || (newValue == null) || (oldValue.length == 0))
return (originalString);
//
// set search/replace flags
//
var Flags: string = (ignoreCase) ? "gi" : "g";
//
// apply regex escape sequence on pattern (oldValue)
//
var pattern = oldValue.replace(/[-\[\]\/\{\}\(\)\*\+\?\.\\\^\$\|]/g, "\\$&");
//
// replace oldValue with newValue
//
var str = originalString.replace(new RegExp(pattern, Flags), newValue);
return (str);
}
在打字稿中, String.Replace 只替換匹配字符串的第一次出現。 需要 String.replaceAll() 方法
TypeScript 在這里沒有什么特別之處( 畢竟 TypeScript 只是帶有類型注釋的 JavaScript )。 JavaScript string.replace
僅在給定字符串時替換第一個實例。 獲得全部替換的唯一方法是使用帶有/g
修飾符的regex
。
或者我只是這樣做:
somestring.split('oldString').join('newString');
就我而言,我在 TypeScript 中確實喜歡這樣做。
this.mystr= this.mystr.replace(new RegExp('class="dec-table"', 'g'), 'class="copydec-table"');
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.