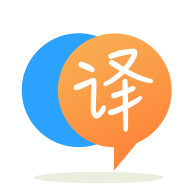
[英]Using jQuery to require user to press every letter and number on keyboard
[英]Regex in jQuery validation - require a number with 5 digits and 1 letter (letter is not case sensitive)
我正在嘗試使用一些驗證來制作表單。 我遇到的一個問題是注冊號驗證。 每個用戶已經屬於一個關聯,所以當他們聯系我們時,我們必須通過檢查數據庫中的數字來驗證它們。 我需要的幫助是注冊號上的正則表達式。
目標是讓他們提交一個5位數和1個字母的數字 。 這封信不區分大小寫。 到目前為止,我試過這個:
^(\d{5})([A-Z]{1})$
像12345A這樣的任何數字都是可以接受的。
這種驗證看起來有點過分,但我們的用戶傾向於經常插入錯誤的信息而不能立即回復電子郵件,因此他們可以提交正確的詳細信息,而無需我們追查他們的正確信息。
任何幫助或指出錯誤表示贊賞。
contact.js
$(function() { // Validate the contact form $('#contactform').validate({ // Specify what the errors should look like // when they are dynamically added to the form errorElement: "label", wrapper: "td", errorPlacement: function(error, element) { error.insertBefore(element.parent().parent()); error.wrap("<tr class='error'></tr>"); $("<td></td>").insertBefore(error); }, // Add requirements to each of the fields rules: { name: { required: true, minlength: 2 }, email: { required: true, email: true }, RegNo: { required: true, minlength: 6 }, tuname: { required: true, minlength: 1 }, dob: { required: true, minlength: 10 } }, // Specify what error messages to display // when the user does something horrid messages: { name: { required: "Please enter your name.", minlength: jQuery.format("At least {0} characters required.") }, email: { required: "Please enter your email.", email: "Please enter a valid email." }, RegNo: { required: "Please enter your Reg No. (eg. 12345A)", minlength: jQuery.format("At least {0} characters required. eg. 12345A") }, tuname: { required: "Please enter your Twitter Username.", minlength: jQuery.format("At least {0} characters required.") }, dob: { required: "Please enter a DOB.", dob: jQuery.format("At least {0} characters required.") } }, // Use Ajax to send everything to processForm.php submitHandler: function(form) { $("#send").attr("value", "Sending..."); $(form).ajaxSubmit({ success: function(responseText, statusText, xhr, $form) { $(form).slideUp("fast"); $("#response").html(responseText).hide().slideDown("fast"); } }); return false; } }); }); strong text
<?php // Clean up the input values foreach($_POST as $key => $value) { if(ini_get('magic_quotes_gpc')) $_POST[$key] = stripslashes($_POST[$key]); $_POST[$key] = htmlspecialchars(strip_tags($_POST[$key])); } // Assign the input values to variables for easy reference $name = $_POST["name"]; $email = $_POST["email"]; $RegNo = $_POST["RegNo"]; $tuname = $_POST["tuname"]; $dob = $_POST["DOB"]; // Test input values for errors $errors = array(); if(strlen($name) < 2) { if(!$name) { $errors[] = "You must enter a name."; } else { $errors[] = "Name must be at least 2 characters."; } } if(!$email) { $errors[] = "You must enter an email."; } else if(!validEmail($email)) { $errors[] = "You must enter a valid email."; } if(strlen($RegNo) < 6) { if(!$RegNo) { $errors[] = "You must enter a Reg Number. (eg 12345A)"; } else { $errors[] = "Name must be at least 6 characters. (eg 12345A)"; } } if(strlen($tuname) < 2) { if(!$tuname) { $errors[] = "You must enter your twitter username."; } else { $errors[] = "Username cannot be blank."; } } if(strlen($DOB) < 10) { if(!$DOB) { $errors[] = "You must enter a DOB"; } else { $errors[] = "DOB must be at least 10 characters."; } } if($errors) { // Output errors and die with a failure message $errortext = ""; foreach($errors as $error) { $errortext .= "<li>".$error."</li>"; } die("<span class='failure'>The following errors occured:<ul>". $errortext ."</ul></span>"); } // Send the email $to = "email@domain.com"; $subject = "email subject"; $message = "$name, $RegNo, $tuname, $dob"; $headers = "From: $email"; mail($to, $subject, $message, $headers); // Die with a success message die("<span class='success'>Success! Your message has been sent.</span>"); // A function that checks to see if // an email is valid function ValidatePPS($RegNo) { var formatRegex = /^(\\d{7})([AZ]{1,2})$/i; if (!formatRegex.test($RegNo)) { return "The format of the provided PPSN is invalid"; } } function validEmail($email) { $isValid = true; $atIndex = strrpos($email, "@"); if (is_bool($atIndex) && !$atIndex) { $isValid = false; } else { $domain = substr($email, $atIndex+1); $local = substr($email, 0, $atIndex); $localLen = strlen($local); $domainLen = strlen($domain); if ($localLen < 1 || $localLen > 64) { // local part length exceeded $isValid = false; } else if ($domainLen < 1 || $domainLen > 255) { // domain part length exceeded $isValid = false; } else if ($local[0] == '.' || $local[$localLen-1] == '.') { // local part starts or ends with '.' $isValid = false; } else if (preg_match('/\\\\.\\\\./', $local)) { // local part has two consecutive dots $isValid = false; } else if (!preg_match('/^[A-Za-z0-9\\\\-\\\\.]+$/', $domain)) { // character not valid in domain part $isValid = false; } else if (preg_match('/\\\\.\\\\./', $domain)) { // domain part has two consecutive dots $isValid = false; } else if(!preg_match('/^(\\\\\\\\.|[A-Za-z0-9!#%&`_=\\\\/$\\'*+?^{}|~.-])+$/', str_replace("\\\\\\\\","",$local))) { // character not valid in local part unless // local part is quoted if (!preg_match('/^"(\\\\\\\\"|[^"])+"$/', str_replace("\\\\\\\\","",$local))) { $isValid = false; } } if ($isValid && !(checkdnsrr($domain,"MX") || checkdnsrr($domain,"A"))) { // domain not found in DNS $isValid = false; } } return $isValid; } function validateDate($dob) { $d = DateTime::createFromFormat('dm-Y', $dob); return $d && $d->format('dm-Y') === $dob; } ?> **index.html**
<div id="form-group" align="center"> <form id="contactform" action="processForm.php" method="post"> <table> <br> <tr> <td> <p> <label for="name">Full Name:</label> </td> <td> <input type="text" id="name" name="name" class="form-control" /> </p> </td> </tr> <tr> <td> <p> <label for="email">Email Address:</label> </td> <td> <input type="email" id="email" name="email" class="form-control" /> </p> </td> </tr> <tr> <td> <p> <label for="RegNo">Garda Reg No:</label> </td> <td> <input type="text" id="RegNo" name="RegNo" class="form-control"></input> </p> </td> </tr> <tr> <td> <p> <label for="tuname">Twitter Username:</label> </td> <td> <input type="text" id="tuname" name="tuname" class="form-control"></input> </p> </td> </tr> <tr> <td> <p> <label for="dob">DOB:</label> </td> <td> <input type="date" id="dob" name="dob" class="form-control" placeholder="00/00/00"></input> </p> </td> </tr> <tr> <td></td> <td> <p> <input type="submit" value="Send!" id="send" type="button" class="btn btn-primary" /> </p> </td> </tr> </table> </form> <div id="response"></div> </div>
在你的正則表達式中, {1}
是不必要的,因為[AZ]
已經強制使用單個字符。 表達式可以重寫為^(\\d{5})([AZ])$
。
否則,您的表達式將匹配任何帶有5位數后跟單個字母的字符串。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.