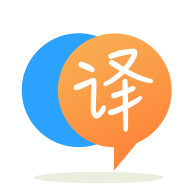
[英]Is there another better way to get file ext from a given path in python
[英]Good way to count number of functions of a python file, given path
我不想導入我的模塊。 我必須計算給定 .py 文件路徑的函數數量。 這樣做的最佳方法是什么?
我想到的一件事是計算代碼中“def”的數量,但這似乎不是解決此問題的最佳方法。 有沒有更好的方法來計算函數的數量?
您可以使用ast.NodeVisitor
:
import inspect
import importlib
import ast
class CountFunc(ast.NodeVisitor):
func_count = 0
def visit_FunctionDef(self, node):
self.func_count += 1
mod = "/path/to/some.py"
p = ast.parse(open(mod).read())
f = CountFunc()
f.visit(p)
print(f.func_count)
如果您想包含 lambda,您需要添加一個visit_Lambda
:
def visit_Lambda(self, node):
self.func_count += 1
這將找到所有定義,包括任何類的方法,我們可以添加更多限制來禁止:
class CountFunc(ast.NodeVisitor):
func_count = 0
def visit_ClassDef(self, node):
return
def visit_FunctionDef(self, node):
self.func_count += 1
def visit_Lambda(self, node):
self.func_count += 1
您可以根據自己的喜好定制代碼, greentreesnakes 文檔中描述了所有節點及其屬性
要計算頂級定義,請使用ast
模塊,如下所示:
import ast
with open(filename) as f:
tree = ast.parse(f.read())
sum(isinstance(exp, ast.FunctionDef) for exp in tree.body)
Padraic 擊敗了我,但這是我的代碼,它適用於 Python 2 和 Python 3。
from __future__ import print_function
import ast
class FunctionCounter(ast.NodeVisitor):
def __init__(self, filename):
self.function_count = 0
with open(filename) as f:
module = ast.parse(f.read())
self.visit(module)
def visit_FunctionDef(self, node):
print('function: {}'.format(node.name))
self.function_count += 1
# Uncomment this to disable counting methods, properties within a
# class
# def visit_ClassDef(self, node):
# pass
if __name__ == '__main__':
counter = FunctionCounter('simple.py')
print('Number of functions: {}'.format(counter.function_count))
visit_ClassDef
的 2 行您可以使用pyclbr
模塊來獲取模塊的結果,唯一的問題是您需要使用模塊的名稱,因為您將導入它而不是文件路徑,這也得益於from X import Y
識別 python 源基於模塊(不是像math
這樣的內置模塊)
from pyclbr import readmodule_ex, Function
#readmodule_ex is function 1
def test(): #2
pass
def other_func(): #3
pass
class Thing:
def method(self):
pass
result = readmodule_ex("test") #this would be it's own file if it is test.py
funcs = sum(isinstance(v,Function) for v in result.values())
print(funcs)
你可以使用
len(dir(module))
希望它有幫助
我的回答是對@ Naveen Kumar 的回答的編輯,因為我目前無法編輯他的回答。 您可以使用
import module
len(dir(module))
IE:
import math
print(len(dir(math)))
輸出:
63
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.