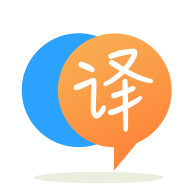
[英]Find smallest integer greater or equal than x (positive integer) multiple of z (positive integer, probably power of 2)
[英]How do I find the largest value smaller than or equal to X and the smallest value greater than or equal to X?
我正在嘗試使用C ++ algorithm
庫中的lower_bound
和upper_bound
函數來查找以下內容:
我寫了以下代碼:
#include <iostream>
#include <algorithm>
int main() {
using namespace std;
int numElems;
cin >> numElems;
int ar[numElems];
for (int i = 0; i < numElems; ++i)
cin >> ar[i];
stable_sort(ar,ar+numElems);
cout << "Input number X to find the largest number samller than or equal to X\n";
int X;
cin >> X;
int *iter = lower_bound(ar,ar+numElems,X);
if (iter == ar+numElems)
cout << "Sorry, no such number exists\n";
else if (*iter != X && iter != ar)
cout << *(iter-1) << endl;
else
cout << *iter << endl;
cout << "Input number X to find the smallest number greater than or equal to X\n";
cin >> X;
int *iter2 = lower_bound(ar,ar+numElems,X);
if (iter2 == ar+numElems)
cout << "Sorry, no such number exists\n";
else
cout << *iter2 << endl;
return 0;
}
但是對於一些隨機的測試案例,它給了我錯誤的答案。
誰能在我的程序中找到不正確的代碼?
讓我告訴您您在哪里出錯了:
int array[] = { 10, 15, 18 };
//Input number X to find the largest number samller than or equal to X
//X = 16
15 //Correct!
//X = 200
Sorry, no such number exists //Wrong! Should be 18
//X = 1
10 //Wrong! There is no such number
//Input number X to find the smallest number greater than or equal to X
//X = 16
18 //Correct!
//X = 200
Sorry, no such number exists //Correct!
//X = 1
10 //Correct!
如您所見,第一個測試用例是罪魁禍首,您錯誤地認為如果最后一個元素比最后一個元素晚一個,則它找不到該元素。 但這不是事實,因為最后一個元素將永遠是最小的元素! 您應該刪除條件。
接下來,對於第三個輸入,您永遠不會檢查iter == ar
,但是應該這樣做,因為找到第一個元素時iter == ar
,如果它不是X
,那么就沒有這樣的數字(它不能成為之前的那個,因為iter
已經是第一個!
對於“小於或等於”的情況,您的邏輯有些倒退。
對於lower_bound
的結果,需要考慮三種情況:它返回最后一個元素之后的位置,第一個元素的位置或之間的某個位置
如果iter == ar+numElems
,則您要查找的值是數組的最后一個元素(因為所有元素都小於X
)。
如果iter == ar
(第一個位置),則有兩種情況: *iter == X
和*iter != X
如果*iter == X
,則X
是您的結果,因為數組中沒有較小的值。
如果*iter != X
,則最小數組元素大於X
,則沒有結果。
否則(即iter != ar
),結果為ar[-1]
。
小於或等於數字X的最大值。
對我來說,排序數組最簡單的方法是:
auto ret = upper_bound(arr.rbegin(), arr.rend(), X,
[](int a, int b){return a>=b;});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.