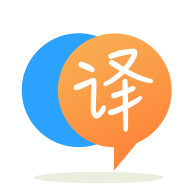
[英]How to find unique elements in a two-dimensional array of strings in java?
[英]how to find max value of the two-dimensional array in java using multithreading
我想找到二維數組的最大值。 我發現此值不使用多線程。 如何使用多線程查找二維數組的最大值? 我想比較以不同方式查找數組最大值的速度。
public class Search {
public int[][] fillMatrix(int matrix[][]) {
for (int i = 0; i < matrix.length; i++){
for (int j = 0; j < matrix[i].length; j++){
matrix[i][j] = (int)(Math.random() * 1000);
}
}
return matrix;
}
public int searchMaxValue(int[][] matrix, int row, int column) {
int max = matrix[0][0];
for (int a = 0; a < row; a++) {
for (int b = 0; b < column; b++) {
try {
Thread.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
if (matrix[a][b] > max) {
max = matrix[a][b];
}
}
}
return max;
}
public static void main(String[] args) {
Search search = new Search();
int[][] matrix = new int[4][100];
search.fillMatrix(matrix);
long start = System.currentTimeMillis();
int max = search.searchMaxValue(matrix, 4, 100);
long end = System.currentTimeMillis();
System.out.println("Max value is " + max);
System.out.println("Time for execution: " + (end - start));
}
}
最簡單的方法是啟動一個線程,以計算矩陣中四行中的每一行的最大值,讓主線程加入所有這些行線程,並計算四行最大值的最大值。 但是,您可能需要更大的數組才能看到時差。 不要忽略必要的同步。
如果我懷疑您正在尋找代碼,則應該嘗試解決方案並運行它,然后重新發布或詳細說明遇到的問題。
這是在Java 8中的操作方法:
int[][] values = fillMatrix(new int[1000][1000]);
OptionalInt max = Arrays.stream(values)
.parallel()
.flatMapToInt(Arrays::stream)
.parallel()
.max();
但是坦率地說,我不確定使用這么多個線程進行這種簡單的計算是否有意義,實際上創建和編排線程的代價在這種情況下似乎太高了。
回應更新
由於這是您的家庭作業,因此我提供了一個沒有任何評論的答案,目的是確保您至少考慮一下它的工作原理,但是主要思想是為每個線程提供數據塊,以避免接下來發生沖突:
int[][] matrix = fillMatrix(new int[100][100]);
int totalThreads = 10;
int[] results = new int[totalThreads];
int chunkSize = matrix.length / totalThreads;
CountDownLatch end = new CountDownLatch(totalThreads);
for (int i = 0; i < totalThreads; i++) {
int threadIndex = i;
new Thread(
() -> {
int max = -1;
int startIndex = threadIndex * chunkSize;
for (int j = startIndex; j < startIndex + chunkSize && j < matrix.length; j++) {
for (int k = 0; k < matrix[j].length; k++) {
if (max == -1 || max < matrix[j][k]) {
max = matrix[j][k];
}
}
}
results[threadIndex] = max;
end.countDown();
}
).start();
}
end.await();
int max = results[0];
for (int k = 1; k < results.length; k++) {
if (max < results[k]) {
max = results[k];
}
}
System.out.printf("Max found %d%n", max);
這是實現此目標的概述。 我不是故意提供代碼,因此您可以自己實現它。
創建一個從數組中查找findmax的方法,將其
findMax(int[] input)
對於2D數組中的每個子數組(可以使用
matrix[i]
進行訪問)
在線findMax(matrix[i])
一個線程以findMax(matrix[i])
(提示:使用ExecutorService
),一旦找到max,將其填充到該線程中名為results
的一維數組的第i
個位置,指示其完成(提示:使用CountDownLatch
)在主線程中,等待所有線程完成(提示:使用
CountDownLatch
),然后調用findMax(results)
,您便擁有了矩陣的findMax(results)
。
注意事項:我們是否需要派出與矩陣中的行一樣多的線程? 那么,我們是否使用具有行數的FixedThreadPool
?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.