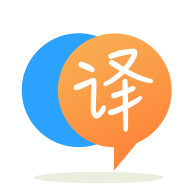
[英]How to delete a section and a row from a TableView with Realm and Swift?
[英]How do select a row from each section of the tableview in swift?
我想從同一個表視圖的不同部分中選擇一行。 我正在獲取許多行正在選擇的輸出,但我只想從每個部分中選擇一行。
這是我的陣列:
var filterFeedUnderAll = ["Complex","NotComplex","Easy"]
var filterFeedUnderAllStocks = ["AllStocks","Portfolio","Watchlist","Sector","Ticker"]
var filterFeedUnderByDate = ["ByDate","ByComments","ByLikes"]
我用過的方法:
func numberOfSectionsInTableView(tableView: UITableView) -> Int
{
return 3
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
var count:Int?
if section == 0
{
count = filterFeedUnderAll.count
}
else if section == 1
{
count = filterFeedUnderAllStocks.count
}
else if section == 2
{
count = filterFeedUnderByDate.count
}
return count!
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cell = self.m_HomeFeedFilterBaseTableView.dequeueReusableCellWithIdentifier("cell1", forIndexPath: indexPath) as! HomeFeedFIlterBaseTableViewCell
switch (indexPath.section)
{
case 0:
cell.m_TableItemsLabel.text = filterFeedUnderAll[indexPath.row]
case 1:
cell.m_TableItemsLabel.text = self.filterFeedUnderAllStocks[indexPath.row]
case 2:
cell.m_TableItemsLabel.text = filterFeedUnderByDate[indexPath.row]
default:
cell.m_TableItemsLabel.text = "Other"
}
return cell
}
func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath)
{
let cell = m_HomeFeedFilterBaseTableView.cellForRowAtIndexPath(indexPath) as! HomeFeedFIlterBaseTableViewCell
for selectedIndexPath: NSIndexPath in tableView.indexPathsForSelectedRows!
{
if selectedIndexPath.section == indexPath.section
{
cell.m_TableItemsLabel.textColor = selectedTextColor
tableView.deselectRowAtIndexPath(indexPath, animated: true)
}
}
}
我想從每個部分中選擇一行。 幫助我完成這項任務。
首先你需要在tableView
啟用多個選擇,然后這是我以前做過的代碼,請注意我使用格式為[String:NSIndexPath]
名為selectedRows
的Dictionary
,其中我按部分存儲一個indexPath我這樣做在addSelectedCellWithSection
最后一次快速更新
import UIKit
class ViewController: UIViewController,UITableViewDataSource,UITableViewDelegate,UITextFieldDelegate {
@IBOutlet weak var tableView: UITableView!
var filterFeedUnderAll = ["Complex","NotComplex","Easy"]
var filterFeedUnderAllStocks = ["AllStocks","Portfolio","Watchlist","Sector","Ticker","bla bla bla1","bla bla bla2","bla bla bla3","bla bla bla1","bla bla bla2","bla bla bla3","bla bla bla1","bla bla bla2","bla bla bla3"]
var filterFeedUnderByDate = ["ByDate","ByComments","ByLikes"]
var selectedRows = [String:IndexPath]()
var alert : UIAlertController?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func numberOfSections(in tableView: UITableView) -> Int
{
return 3
}
func tableView(_ tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return 50;
}
func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
let headerCell = tableView.dequeueReusableCell(withIdentifier: "headerCell") as! mycustomHeader
let layer = CAShapeLayer()
let corners = UIRectCorner.topLeft.union(UIRectCorner.topRight)
layer.path = UIBezierPath(roundedRect: CGRect(x: 0, y: 0, width: headerCell.frame.width, height: headerCell.frame.height), byRoundingCorners: corners, cornerRadii:CGSize(width: 20.0, height: 20.0)).cgPath
headerCell.layer.mask = layer
return headerCell
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
var count:Int?
if section == 0
{
count = filterFeedUnderAll.count
}
else if section == 1
{
count = filterFeedUnderAllStocks.count
}
else if section == 2
{
count = filterFeedUnderByDate.count
}
return count!
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
{
let cell = self.tableView.dequeueReusableCell(withIdentifier: "cell1", for: indexPath) as! testCell
switch (indexPath.section)
{
case 0:
cell.lblName.text = filterFeedUnderAll[indexPath.row]
case 1:
cell.lblName.text = self.filterFeedUnderAllStocks[indexPath.row]
case 2:
cell.lblName.text = filterFeedUnderByDate[indexPath.row]
default:
cell.lblName.text = "Other"
}
cell.lblName.textColor = UIColor.black
if(self.indexPathIsSelected(indexPath)) {
cell.lblName.textColor = UIColor.red
}
return cell
}
func addSelectedCellWithSection(_ indexPath:IndexPath) ->IndexPath?
{
let existingIndexPath = selectedRows["\(indexPath.section)"]
selectedRows["\(indexPath.section)"]=indexPath;
return existingIndexPath
}
func indexPathIsSelected(_ indexPath:IndexPath) ->Bool {
if let selectedIndexPathInSection = selectedRows["\(indexPath.section)"] {
if(selectedIndexPathInSection.row == indexPath.row) { return true }
}
return false
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let cell = self.tableView.cellForRow(at: indexPath) as! testCell
let previusSelectedCellIndexPath = self.addSelectedCellWithSection(indexPath);
if(previusSelectedCellIndexPath != nil)
{
let previusSelectedCell = self.tableView.cellForRow(at: previusSelectedCellIndexPath!) as! testCell
previusSelectedCell.lblName.textColor = UIColor.black
cell.lblName.textColor = UIColor.red
tableView.deselectRow(at: previusSelectedCellIndexPath!, animated: true)
}
else
{
cell.lblName.textColor = UIColor.red
}
for selectedIndexPath: IndexPath in tableView.indexPathsForSelectedRows!
{
if selectedIndexPath.section == indexPath.section
{
cell.lblName.textColor = UIColor.red
tableView.deselectRow(at: indexPath, animated: true)
}
}
}
}
希望這對你有所幫助,對我來說是完美的
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.