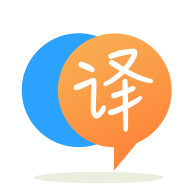
[英]Read user input “string” and “int” in one line separated by space (C)
[英]C program to convert input string of space separated ints into an int array
題:
我想創建一個C程序,它將一串空格分隔的int作為輸入(正數和負數,可變數字位數)並將字符串轉換為int數組。
從Stack Overflow上的字符串輸入讀取整數還有另一個問題,但它不適用於數字長度大於1或負數的數字。
嘗試:
#include <stdio.h>
int main () {
int arr[1000], length = 0, c;
while ((c = getchar()) != '\n') {
if (c != ' ') {
arr[length++] = c - '0';
}
}
printf("[");
for ( int i = 0; i < length-1; i++ ) {
printf("%d,", arr[i]);
}
printf("%d]\n", arr[length-1]);
}
如果我在終端輸入以下內容:
$ echo "21 7" | ./run
$ [2,1,7]
這是我得到的數組:[2,1,7]而不是[21,7]
如果我輸入以下內容:
$ echo "-21 7" | ./run
$ [-3,2,1,7]
我得到:[ - 3,2,1,7]而不是[-21,7]這沒有任何意義。
但是,如果我輸入:
$ echo "1 2 3 4 5 6 7" | ./run
$ [1,2,3,4,5,6,7]
注意:我假設輸入它總是一串空格分隔的整數。
這是一個准系統版本(沒有錯誤檢查,數字后應留下尾隨空格),我相信你可以從這里拿起:
int main(void)
{
int c;
int i, num = 0, neg = 0;
while ((c = getchar()) != EOF) {
if (c != ' ') {
if (c == '-') {
neg = 1;
} else {
i = c - '0';
num = num * 10 + i;
}
} else {
(neg == 1) ? num *= -1 : num;
printf("%d\n", num + 2); // this is just to show that you indeed get an integer and addition works
num = 0;
neg = 0;
}
}
}
完整的程序(改編自@onemasse的答案 )(不再需要無效輸入來停止讀取輸入):
#include <stdio.h>
#include <stdlib.h>
int main () {
int arr[1000], length = 0, c, bytesread;
char input[1000];
fgets(input, sizeof(input), stdin);
char* input1 = input;
while (sscanf(input1, "%d%n", &c, &bytesread) > 0) {
arr[length++] = c;
input1 += bytesread;
}
printf("[");
for ( int i = 0; i < length-1; i++ ) {
printf("%d,", arr[i]);
}
printf("%d]\n", arr[length-1]);
return 0;
}
從scanf
/ sscanf
手冊頁:
這些函數返回分配的輸入項數。 在匹配失敗的情況下,這可能比提供的數量少,甚至為零。
因此,如果返回值為0,則表示無法再進行轉換。
樣本I / O:
$ ./parse
1 2 3 10 11 12 -2 -3 -12 -124
[1,2,3,10,11,12,-2,-3,-12,-124]
注意 :我目前不確定這是如何工作的。 我會仔細看看的。 但是,如果有人理解,請編輯此帖或發表評論。
我做的不多,但這是我的去:)
#include"stdio.h"
#include"string.h"
#include"stdlib.h"
#define CHUNK 1000000
#define INT_COUNT 10000
int main(void) {
/*get all of the input*/
char temp_str[CHUNK] = "";
char* full_string = malloc(CHUNK * sizeof(char));
if (full_string == 0) {
printf("Memory Error\n");
exit(1);
}
int count = 2;
do {
fgets(temp_str, CHUNK, stdin);
strcat(full_string, temp_str);
full_string = realloc(full_string, count * CHUNK * sizeof(char));
if (full_string == 0) {
printf("Memory Error\n");
exit(1);
}
count++;
} while (strlen(temp_str) == CHUNK - 1 && temp_str[CHUNK - 2] != '\n');
//parse the input
char* token = strtok(full_string, " ");
int* arr = malloc(INT_COUNT * sizeof(int)), length = 0;
if (arr == 0) {
printf("Memory Error\n");
exit(1);
}
count = 1;
while (token != 0) {
arr[length] = atoi(token);
token = strtok(0, " ");
length++;
if (length == count * INT_COUNT) {
count++;
arr = realloc(arr, count * INT_COUNT);
if(arr == 0) {
printf("Memory Error\n");
exit(1);
}
}
}
free(full_string);
//print the integers
for (int i = 0; i < length; i++) {
printf("%d ", arr[i]);
if (i % 20 == 0 && i != 0) {
printf("\n");
}
}
free(arr);
return 0;
}
編輯了一下。 但是還有減號問題。 我稍后回顧一下。 如果你稍微調整一下,我猜,它可能會奏效。 你嘗試自己的方式。 我會試試我的。 但后來。
#include <stdio.h>
int main () {
int arr[1000]={0}, length = 0, c, i;
while (1) {
c = getchar();
if(c=='-')
{
//minus sign has problem yet. I ll come back once I have better soln.
}
else if(c==' ' || c=='\n')
{
length-=2;
arr[length]= arr[length]*10 + arr[length+1];
length++;
if(c=='\n')
{
break;
}
}
else if (c != ' ') {
arr[length++] = c - '0';
}
}
printf("[");
for (i = 0; i < length-1; i++ ) {
printf("%d,", arr[i]);
}
printf("%d]\n", arr[length-1]);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.