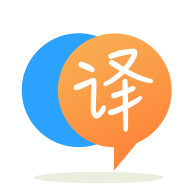
[英]How to randomly pick four elements from an array without repeating in Java?
[英]How to display randomly selected strings from an array in java without repetitions
我正在嘗試使用NetBeans IDE 6.9.1創建Bingo游戲(我是編碼方面的菜鳥)。 現在,我被困在嘗試創建賓果卡。 對於5x5卡的盒子,我使用了jButton。 我無法在“ B”列中隨機分配“ B”賓果球。 我有一個“工作”的代碼,用於隨機化哪個“ B”球進入哪個“ B” jButton,但是我的方法不適用於我用來輸出隨機繪制的賓果球的jLabel。 這是我的“隨機B球代碼”:
String[] Bball1 = {"B5", "B6", "B11"};
String Brandom1 = (Bball1[new Random().nextInt(Bball1.length)]);
String[] Bball2 = {"B1", "B8", "B15"};
String Brandom2 = (Bball2[new Random().nextInt(Bball2.length)]);
String[] Bball3 = {"B3", "B10", "B13"};
String Brandom3 = (Bball3[new Random().nextInt(Bball3.length)]);
String[] Bball4 = {"B2", "B9", "B14"};
String Brandom4 = (Bball4[new Random().nextInt(Bball4.length)]);
String[] Bball5 = {"B4", "B7", "B12"};
String Brandom5 = (Bball5[new Random().nextInt(Bball5.length)]);
以下是用戶選擇賓果圖案時單擊提交按鈕時的代碼,該卡片將生成(不完整):
btnSubmit.setEnabled(false);
cboPattern.setEnabled(false);
btn1B.setText(Brandom1);
btn2B.setText(Brandom2);
btn3B.setText(Brandom3);
btn4B.setText(Brandom4);
btn5B.setText(Brandom5);
是的,這是重復性的,並且不是隨機的,但是我對數組做了一些研究,因為我在計算機科學課上還沒有學到它們,並且得到了:
public static void main(String[] Bballs) {
String[] Bball;
Bball = new String[2];
Bball[0] = "B1";
Bball[1] = "B2";
Bball[2] = "B3";
int num = (int) (Math.random() * 2);
System.out.println(Bball[num]);
}
這只是一個測試代碼,如您所見,我仍然可以多次獲得B1,這不是我想要的賓果卡和隨機挑選的賓果球(我還沒有開始)的意思。 另外,每當我運行程序時,它都不會打印出最后一行。 我需要在星期三結束前完成此操作:/(這是ISU項目的一個較晚項目,不,我沒有晚開始)。 謝謝你的時間。
我希望這並不太遠,但是從一包N
物品(球)中取出M
元素而不重復的最簡單方法是簡單地將所有元素添加到List
並shuffle()
,然后使用subList()
獲取前M
值。
這是執行此操作的通用方法:
private static List<String> draw(String ballPrefix, int noOfBalls, int noToDraw) {
List<String> balls = new ArrayList<>();
for (int ballNo = 1; ballNo <= noOfBalls; ballNo++)
balls.add(ballPrefix + ballNo);
Collections.shuffle(balls);
return balls.subList(0, noToDraw);
}
測試
System.out.println(draw("B", 15, 5));
樣品輸出
[B9, B5, B3, B2, B15]
更新
好的,為了便於教學, List
有點類似於數組,但是功能更強大。 它包含一個值序列(如數組),並且值從0
開始索引,也像數組一樣。
與數組不同,在數組中您使用arr.length
獲得長度,對於列表,您調用list.size()
。 而不是使用arr[2]
檢索值,而是調用list.get(2)
。
可以使用list.set(2, 7)
而不是使用arr[2] = 7
分配值list.set(2, 7)
但這並不常見。 這是因為與使用new String[5]
分配的數組分配5個值的固定大小的數組不同,使用new ArrayList()
分配了列表,並且在調用add()
new ArrayList()
,列表的大小將根據需要add()
,就像上面的方法一樣。
在簡短介紹之后,下面是您使用上述方法的方式:
List<String> balls = draw("B", 15, 5);
btn1B.setText(balls.get(0));
btn2B.setText(balls.get(1));
btn3B.setText(balls.get(2));
btn4B.setText(balls.get(3));
btn5B.setText(balls.get(4));
如果要獲取隨機數,可以使用Math.random()
或Random
,這與您的問題無關。 您的問題就是您要顯示的內容。 一種方法是從特殊的最大數中獲得隨機數,並且最大數不變。 我想這就是您現在使用的。 另一種方法,當您從特殊的最大數中獲得一個隨機數,然后刪除該隨機數時,請確保將使用每個數字(從最小到最大)。 Java有一個Collections.java
工具,該工具具有shuffle
功能,可以使整個列表隨機化。 以下是適合您的示例測試代碼。 如果您希望每個數字(從最小到最大)均等地出現,則可以使用averageRandom_1
或averageRandom_2
。
import java.util.*;
public class Main {
public static void main(String[] args) {
System.out.println("Hello World!");
String[] src = new String[]{"B1", "B2", "B3", "B4", "B5"};
List<String> srcList = Arrays.asList(src);
/* for(int i = 0; i < srcList.size(); i++){
System.out.print(" random = " + getRandom_2(0, srcList.size()));
}*/
averageRandom_2(srcList);
}
/**
* @param min
* @param max
* @return [min, max), note it can't return max
*/
public static int getRandom_1(int min, int max){
return (int)(min + Math.random()*(max-min));
}
/**
* @param min
* @param max
* @return [min, max), note it can't return max
*/
public static int getRandom_2(int min, int max){
Random random = new Random();
return random.nextInt(max-min) + min;
}
public static void averageRandom_1(List<String> data){
List<String> dataCopy = new ArrayList<>();
dataCopy.addAll(data);
Collections.shuffle(dataCopy);
for(String item : dataCopy){
System.out.println("--" + item + "--");
}
}
public static void averageRandom_2(List<String> data){
List<String> dataCopy = new ArrayList<String>(data);
while(dataCopy.size() > 0) {
int random = getRandom_2(0, dataCopy.size());
System.out.println("random = " + random + "--" + dataCopy.get(random) + "--");
dataCopy.remove(random);
}
}
}
請記住random.nextInt(value)
返回0到(value-1)。 希望對您有所幫助。
您必須知道Math.random()給出一個介於0和1之間的數字。
它永遠不會給出1。
0 < Math.random() < 1
0 < Math.random() * 2 < 2
0 <= (int)(Math.random() * 2) <= 1
請注意下限邏輯,它使您永遠不會獲得索引2。
不要嘗試使用Math.random,您將不會獲得均勻的分布。 使用List和Random.nextInt(int),您將獲得一個非常簡單的解決方案,如下所示。
Random rand = new Random();
List<String> inRandomOrder = new ArrayList<>();
List<String> items = new ArrayList<>();
items.add("blah1");
items.add("blah2");
items.add("blah3");
while (!items.isEmpty()) {
String removed = items.remove(rand.nextInt(items.size()));
inRandomOrder.add(removed)
}
然后,您將以隨機順序獲得商品清單。 如果您需要真正的隨機性,那么必須使用SecureRandom並不是真正的隨機性,只是偽隨機性。 我確信這可能是使用Java 8流功能上實現此目的的一種更簡單的方法,但是我還沒有太多時間來弄亂它們,因此,如果答案是老式的,對不起。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.