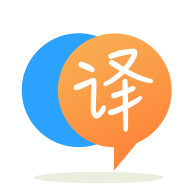
[英]Json parse is not rendering data in the front end using Node.js & hbs
[英]Using AJAX (as RESTful API) in Node.js to GET a JSON file and parse it based on which button in the front end is clicked
我在理解如何在server.js(Node.js)和前端javascript文件之間進行通信時遇到了很多麻煩。 我需要在服務器中使用AJAX作為RESTful API來獲取JSON文件,並根據前端html文件中的某些按鈕對其進行解析。 然后我需要將解析后的JSON發送回我的前端javascript文件進行風格化並放入html元素中進行顯示。
我理解如何在服務器中獲取JSON文件,但我很困惑如何判斷單擊了哪個按鈕(我讀過我不能使用$(“按鈕”)。單擊服務器文件中)如何將解析后的JSON發送回我的前端javascript文件,以便風格化為html元素。
我的代碼:
現在我在前端javascript文件中有RESTful API的東西,這不是它應該的地方..我還沒有改變它,因為我不知道如何在這兩個文件之間進行通信,正如我所說的以上並且不想在沒有如何做到這一點的情況下改變任何東西。
服務器端代碼:
var http = require('http');
var fs = require('fs');
var path = require('path');
var types = {
'.js' : 'text/javascript',
'.html' : 'text/html',
'.css' : 'text/css'
};
var server = http.createServer(function(req, res) {
var lookup = path.basename(decodeURI(req.url)) || 'a3.html';
var f = 'content/' + lookup;
fs.exists(f, function(exists) {
if (exists) {
fs.readFile(f, function(err, data) {
if (err) {
res.writeHead(500);
res.end('Error');
return;
}
var headers = {'Content-type': types[path.extname(lookup)]};
var s = fs.createReadStream(f).once('open', function() {
res.writeHead(200, headers);
this.pipe(res);
});
});
return;
}
res.writeHead(404);
res.end();
});
});
server.listen(8080);
console.log('Server running at http://127.0.0.1:8080/');
客戶端javascript(只有前兩個按鈕才能得到一個想法):
$(document).ready(function()
{
$("button#arts").click(function() {
$.ajax(
{
url: "./nytimes.json",
method: "GET",
dataType: "json"
}).done(function(json) {
for (var i = 0; i < json.num_results; i++) {
var $text = $("<p></p>").text(json.results[i].published_date +
"<br>" + json.results[i].title +
//"<br>" + obj.results[i].abstract +
"<br>" + json.results[i].short_url + "<br>";
$("section").append($text);
}
}).fail(function(jqXHR, textStatus) {
alert("Request failed: " + textStatus);
});
});
$("button#aut").click(function() {
$.ajax(
{
url: "./nytimes.json",
method: "GET",
dataType: "json"
}).done(function(json) {
for (var i = 0; i < json.num_results; i++) {
var authors = JSON.stringify(json.results[i].byline);
var names = authors.slice(3);
var singlename = authors.split("and");
var $list = $("<ul></ul>");
$("section").append($list);
if (singlename.length == 1) {
var $text = $("<li></li>").text(singlename);
$("ul").append($text);
} else if (singlename.length == 2) {
var $text = $("<li></li>").text(singlename[0]);
var $texttwo = $("<li></li>").text(singlename[1]);
$("ul").append($text);
$("ul").append($texttwo);
}
}
}).fail(function(jqXHR, textStatus) {
alert("Request failed: " + textStatus);
});
});
});
和客戶端html文件:
<!DOCTYPE html>
<html>
<head>
<title></title>
<link rel="stylesheet" type="text/css" href="content/style.css">
<script type="text/javascript" src="content/jquery-2.2.4.min.js" defer></script>
<script type="text/javascript" src="content/script.js" defer></script>
</head>
<body>
<ul>
<li>Click the "View Articles" button to view the basic information on all articles.</li>
<li>Click the "View Authors" button to view all known authors.</li>
<li>Click the "View URLs" button to view short URLs for all articles.</li>
<li>Click the "View Tags" button to view all tags connected to articles; tags
are sized from largest to smallest based on tags with the most and least
articles, respectively.
</li>
<li>To view a specific article, enter the article number (beginning with 0) in
the "Article Number" field and click the "Find" button.
</li>
<li>Click the "View Media" button to view all articles as hyperlinked images or video</li>
</ul>
<button id="arts">View Articles</button>
<button id="aut">View Authors</button>
<button id="url">View URLs</button>
<button id="tag">View Tags</button>
<form method="post" action="/">
Article Number: <input id="entry" type="text" name="ArtNum" value="">
<input id="art" type="submit" value="Find">
</form>
<button id="hyper">View Media</button>
<section id="data"></section>
</body>
</html>
$(document).on("click", ".arts", function() {
var cursor = $(this);
$.ajax({
url: "./nytimes.json",
type: "POST",
dataType: "json",
data: {
buttonClicked:'arts' // send info to server
},
cache: false,
timeout: 5000,
complete: function() {
console.log('complete');
},
success: function(data) {
// data received from the server, use it
},
error: function() {
console.log(err);
}
});
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.