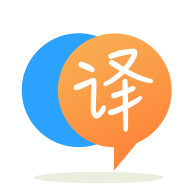
[英]How to test Webview is loaded or not without staticTexts in XCTest in swift
[英]How to test that staticTexts contains a string using XCTest
Xcode UI測試中,如何測試staticTexts是否包含字符串?
在調試器中,我可以運行這樣的程序來打印出 staticTexts 的所有內容: po app.staticTexts
。 但是我如何測試所有內容中是否存在字符串?
我可以檢查每個 staticText 是否存在,比如app.staticTexts["the content of the staticText"].exists
? 但我必須使用那個 staticText 的確切內容。 我怎樣才能只使用可能只是該內容一部分的字符串?
您可以使用NSPredicate
來過濾元素。
let searchText = "the content of the staticText"
let predicate = NSPredicate(format: "label CONTAINS[c] %@", searchText)
let elementQuery = app.staticTexts.containing(predicate)
if elementQuery.count > 0 {
// the element exists
}
使用CONTAINS[c]
指定搜索不區分大小寫。
看看蘋果謂詞編程指南
首先,您需要為要訪問的靜態文本對象設置可訪問性標識符。 這將允許您在不搜索它顯示的字符串的情況下找到它。
// Your app code
label.accessibilityIdentifier = "myLabel"
然后,你可以通過調用編寫一個測試斷言該字符串是否顯示的是你想要的字符串.label
上XCUIElement獲得所顯示的字符串的內容:
// Find the label
let myLabel = app.staticTexts["myLabel"]
// Check the string displayed on the label is correct
XCTAssertEqual("Expected string", myLabel.label)
要檢查它是否包含某個字符串,請使用range(of:)
,如果找不到您提供的字符串,它將返回nil
。
XCTAssertNotNil(myLabel.label.range(of:"expected part"))
我在構建 XCTest 時遇到了這個問題,我的文本塊中有一個動態字符串,我應該驗證。 我構建了這兩個函數來解決我的問題:
func waitElement(element: Any, timeout: TimeInterval = 100.0) {
let exists = NSPredicate(format: "exists == 1")
expectation(for: exists, evaluatedWith: element, handler: nil)
waitForExpectations(timeout: timeout, handler: nil)
}
func waitMessage(message: String) {
let predicate = NSPredicate(format: "label CONTAINS[c] %@", message)
let result = app.staticTexts.containing(predicate)
let element = XCUIApplication().staticTexts[result.element.label]
waitElement(element: element)
}
我知道這篇文章很舊,但我希望這可以幫助別人。
您可以創建擴展以簡單地在XCUIElement
使用它。
extension XCUIElement {
func assertContains(text: String) {
let predicate = NSPredicate(format: "label CONTAINS[c] %@", text)
let elementQuery = staticTexts.containing(predicate)
XCTAssertTrue(elementQuery.count > 0)
}
}
用法:
// Find the label
let yourLabel = app.staticTexts["AccessibilityIdentifierOfYourLabel"].firstMatch
// assert that contains value
yourLabel.assertContains(text: "a part of content of the staticText")
// Encapsulate your code
func yourElement() -> XCUIElement {
let string = "The European languages are members of the same family."
let predicate = NSPredicate(format: "label CONTAINS[c] '\(string)'")
return app.staticTexts.matching(predicate).firstMatch
}
// To use it
XCTAssert(yourPage.yourElement().waitForExistence(timeout: 20))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.