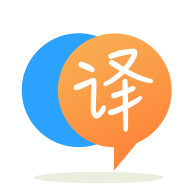
[英]How to get a HashMap result from Spring Data Repository using JPQL?
[英]Get data from a repository using Spring
好的,所以我是春天的新手,並不真正知道這是如何工作的。 我一直在嘗試一些事情,並認為它接近完成但沒有從服務器獲取任何數據並給我這個錯誤
Unsatisfied dependency expressed through constructor argument with index 4 of type [jp.co.fusionsystems.dimare.crm.service.impl.MyDataDefaultService]: : Error creating bean with name 'MyDataDefaultService' defined in file
我的終點
//mobile data endpoint
@RequestMapping(
value = API_PREFIX + ENDPOINT_MyData + "/getMyData",
method = RequestMethod.GET)
public MyData getMyData() {
return MyDataDefaultService.getData();
}
我的對象
public class MyData {
public MyData(final Builder builder) {
videoLink = builder.videoLink;
}
private String videoLink;
public String getVideoLink()
{
return videoLink;
}
public static class Builder
{
private String videoLink = "";
public Builder setVideo(String videoLink)
{
this.videoLink = videoLink;
return this;
}
public MyData build()
{
return new MyData(this);
}
}
@Override
public boolean equals(final Object other) {
return ObjectUtils.equals(this, other);
}
@Override
public int hashCode() {
return ObjectUtils.hashCode(this);
}
@Override
public String toString() {
return ObjectUtils.toString(this);
}
}
存儲庫
public classMyServerMyDataRepository implements MyDataRepository{
private finalMyServerMyDataJpaRepository jpaRepository;
private final MyDataConverter MyDataConverter = new MyDataConverter();
@Autowired
publicMyServerMyDataRepository(finalMyServerMyDataJpaRepository jpaRepository) {
this.jpaRepository = Validate.notNull(jpaRepository);
}
@Override
public MyData getData() {
MyDataEntity entity = jpaRepository.findOne((long) 0);
MyData.Builder builder = new MyData.Builder()
.setVideo(entity.getVideoLink());
return builder.build();
}
端點調用的 DefaultService
public class MyDataDefaultService {
private static final Logger logger = LoggerFactory.getLogger(NotificationDefaultService.class);
private finalMyServerMyDataRepository repository;
@Autowired
public MyDataDefaultService(MyServerMyDataRepository repository) {
this.repository = Validate.notNull(repository);
}
//Get the data from the server
public MobileData getData()
{
logger.info("Get Mobile Data from the server");
//Get the data from the repository
MobileData mobileData = repository.getData();
return mobileData;
}
}
轉換器
public class MyDataConverter extends AbstractConverter<MyDataEntity, MyData>
{
@Override
public MyData convert(MyDataEntity entity) {
MyData.Builder builder = new MyData.Builder()
.setVideo(entity.getVideoLink());
return builder.build();
}
}
我的實體
@Entity
@Table(name = “myServer”)
public class MyDataEntity extends AbstractEntity{
@Column(name = "video_link", nullable = true)
private String videoLink;
public String getVideoLink() {
return videoLink;
}
public void setVideoLink(final String videoLink) {
this.videoLink = videoLink;
}
}
感謝您對此的任何幫助
Hibernate 實體應該定義默認構造函數並實現Serializable
接口,假設AbstractEntity
符合要求。 Hibernate 不會接受沒有主鍵的實體,因此您也必須定義一個:
@Entity
@Table(name = “myServer”)
public class MyDataEntity implements Serializable {
@Id
@GeneratedValue
private Long id;
@Column(name = "video_link", nullable = true)
private String videoLink;
public MyDataEntity() {
}
...setters&getters
}
MyData
對象表示 JSON 服務器響應,您可以使用Jackson
注釋來控制結果 JSON 屬性:
public class MyDataResponse {
@JsonProperty("video_link")
private String videoLink;
public MyDataResponse() {
}
public MyDataResponse(String videoLink) {
this.videoLink = videoLink;
}
...setters&getters
}
Spring 有一個很棒的項目,稱為Spring Data ,它提供了 JPA 存儲庫,因此甚至不需要@Repository
注釋:
public class MyDataRepository extends CrudRepository<MyDataEntity, Long> {
}
Builder
類代表服務層:
@Service
public class MyDataService {
@Autowired
private MyDataRepository myDataRepository;
public MyDataResponse getMyData(Long id) {
MyDataEntity entity = myDataRepository.findOne(id);
...rest logic, copy necessary data to MyDataResponse
}
}
那么控制器是:
@RestController // @ResponseBody not needed when using like this
public MyDataController {
@Autowired
private MyDataService myDataService;
@RequestMapping("/getMyData") // no need to specify method for GET
public MyDataResponse getMyData(@RequestParam("ID") Long myDataId) {
... validation logic
return myDataService.getMyData(myDataId); // return response
}
}
現在它應該可以工作了,不要忘記將所需的依賴項添加到您的類路徑中。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.