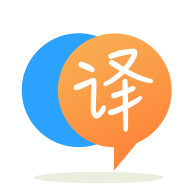
[英]JQuery - How do I get the value that was set in an input field's value attribute?
[英]How do I get the value of a JQuery plugin attribute so I can set it again?
我玩弄所謂的“水泡泡”提供一個簡單的jQuery插件這里 。 我使用如下調用創建了它:
$('#demo-1').waterbubble({
waterColor: 'orange',
textColor: '#fff',
txt: 'OJ',
font: '40px Segoe UI, serif',
wave: false,
animation: true,
radius: 150,
data: 0.2,
});
現在,當我單擊渲染的插件(在這種情況下為畫布)時,我希望值(0.2)增至0.3。 因此,我為所有畫布創建了一個單擊處理程序。
$( "canvas" ).click(function() {
var temp1 = $(this.id); // Gives me the correct ID
var temp2 = $(this.id).waterbubble.data; // Doesn't work
});
如何獲取“數據”字段的值,以便可以再次將其設置為0.3、0.4等? 如果動畫持續存在,那只是最上面的櫻桃。
您可以使用更新的data
值再次調用.waterbubble()
。
運行下面的代碼,單擊以增加水位
jQuery(function($) { var setting = { waterColor: 'orange', textColor: '#fff', txt: 'OJ', font: '40px Segoe UI, serif', wave: false, animation: true, radius: 150, data: 0.1, }; $('#demo-1') .data('waterbubble.setting', setting) .waterbubble(setting) .on('click', function() { var setting = $(this).data('waterbubble.setting'); setting.data = Math.min(1, setting.data + 0.1); $(this).waterbubble(setting); }); });
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <script src="http://www.jqueryscript.net/demo/Customizable-Liquid-Bubble-Chart-With-jQuery-Canvas/waterbubble.js"></script> <p>Click to increase water level</p> <canvas id="demo-1"></canvas>
我不知道它是否是您的理想解決方案,但我嘗試在插件中進行編輯並將新的原型屬性getConf
添加到Waterbubble
。 檢查您的控制台以查看data
。
(function($) {
$.fn.waterbubble = function(options) {
var config = $.extend({
radius: 100,
lineWidth: undefined,
data: 0.5,
waterColor: 'rgba(25, 139, 201, 1)',
textColor: 'rgba(06, 85, 128, 0.8)',
font: '',
wave: true,
txt: undefined,
animation: true
}, options);
var canvas = this[0];
config.lineWidth = config.lineWidth ? config.lineWidth : config.radius/24;
var waterbubble = new Waterbubble(canvas, config);
return this;
}
function Waterbubble (canvas, config) {
this.refresh(canvas, config);
this.getConf(config);
}
Waterbubble.prototype = {
getConf: function (config) {
var data = config.data;
console.log(data);
},
refresh: function (canvas, config) {
canvas.getContext('2d').clearRect(0, 0, canvas.width, canvas.height)
this._init(canvas, config)
},
_init: function (canvas, config){
var radius = config.radius;
var lineWidth = config.lineWidth;
canvas.width = radius*2 + lineWidth;
canvas.height = radius*2 + lineWidth;
this._buildShape(canvas, config);
},
_buildShape: function (canvas, config) {
var ctx = canvas.getContext('2d');
var gap = config.lineWidth*2;
//raidus of water
var r = config.radius - gap;
var data = config.data;
var lineWidth = config.lineWidth
var waterColor = config.waterColor;
var textColor = config.textColor;
var font = config.font;
var wave = config.wave
// //the center of circle
var x = config.radius + lineWidth/2;
var y = config.radius + lineWidth/2;
ctx.beginPath();
ctx.arc(x, y, config.radius, 0, Math.PI*2);
ctx.lineWidth = lineWidth;
ctx.strokeStyle = waterColor;
ctx.stroke();
//if config animation true
if (config.animation) {
this._animate(ctx, r, data, lineWidth, waterColor, x, y, wave)
} else {
this._fillWater(ctx, r, data, lineWidth, waterColor, x, y, wave);
}
if (typeof config.txt == 'string'){
this._drawText(ctx, textColor, font, config.radius, data, x, y, config.txt);
}
return;
},
_fillWater: function (ctx, r, data, lineWidth, waterColor, x, y, wave) {
ctx.beginPath();
ctx.globalCompositeOperation = 'destination-over';
//start co-ordinates
var sy = r*2*(1 - data) + (y - r);
var sx = x - Math.sqrt((r)*(r) - (y - sy)*(y - sy));
//middle co-ordinates
var mx = x;
var my = sy;
//end co-ordinates
var ex = 2*mx - sx;
var ey = sy;
var extent; //extent
if (data > 0.9 || data < 0.1 || !wave) {
extent = sy
} else{
extent = sy - (mx -sx)/4
}
ctx.beginPath();
ctx.moveTo(sx, sy)
ctx.quadraticCurveTo((sx + mx)/2, extent, mx, my);
ctx.quadraticCurveTo((mx + ex)/2, 2*sy - extent, ex, ey);
var startAngle = -Math.asin((x - sy)/r)
var endAngle = Math.PI - startAngle;
ctx.arc(x, y, r, startAngle, endAngle, false)
ctx.fillStyle = waterColor;
ctx.fill()
},
_drawText: function (ctx, textColor, font, radius, data, x, y, txt) {
ctx.globalCompositeOperation = 'source-over';
var size = font ? font.replace( /\D+/g, '') : 0.4*radius;
ctx.font = font ? font : 'bold ' + size + 'px Microsoft Yahei';
txt = txt.length ? txt : data*100 + '%'
var sy = y + size/2;
var sx = x - ctx.measureText(txt).width/2
ctx.fillStyle = textColor;
ctx.fillText(txt, sx, sy)
},
_animate: function (ctx, r, data, lineWidth, waterColor, x, y, wave) {
var datanow = {
value: 0
};
var requestAnimationFrame = window.requestAnimationFrame || window.msRequestAnimationFrame || window.mozRequestAnimationFrame || window.webkitRequestAnimationFrame || function (func) {
setTimeout(func, 16);
};
var self = this;
var update = function () {
if (datanow.value < data) {
datanow.value += (data - datanow.value)/15
self._runing = true;
} else {
self._runing = false;
}
}
var step = function () {
self._fillWater(ctx, r, datanow.value, lineWidth, waterColor, x, y, wave);
update();
if (self._runing) {
requestAnimationFrame(step);
}
}
step(ctx, r, datanow, lineWidth, waterColor, x, y, wave)
}
}
}(jQuery));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.