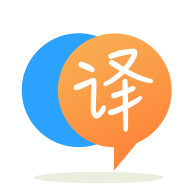
[英]spring-boot Maven: How to create executable jar with main class?
[英]Create a simple JAR instead of an executable JAR with Spring-Boot
我有一個多模塊 Spring-Boot 應用程序的問題。
我有一個用於服務的模塊,即核心模塊。 還有一個與視圖相關的類的模塊, web -Module。 它們都在父模塊中,我在其中添加了依賴項,例如“spring-boot-starter”,並且可以由兩個模塊使用。
現在問題來了:
我想使用嵌入式 Tomcat 運行web -Module,並將核心-Module 作為web -module 中的依賴項。
在其他 Spring 項目中,我只會包含maven-jar-plugin並創建一個核心模塊的 jar。
這個 Spring-Boot 項目的問題是maven-jar-plugin已經在“spring-boot-starter”中配置好了。 它需要一個 mainClass,只有web模塊才有。
摘自“spring-boot-starter”-POM
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<archive>
<manifest>
<mainClass>${start-class}</mainClass>
<addDefaultImplementationEntries>true</addDefaultImplementationEntries>
</manifest>
</archive>
</configuration>
</plugin>
有沒有辦法將核心-Module 打包為 JAR 而不需要該模塊中的“起始類”?
似乎您可以通過配置spring-boot-maven-plugin
來禁用 fat-JAR 替換原始文件並安裝到 repo 中。
默認情況下,重新打包目標將用可執行文件替換原始工件。 如果您只需要部署原始 jar 並且能夠使用常規文件名運行您的應用程序,請按如下方式配置插件:
<project>
...
<build>
...
<plugins>
...
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>1.5.1.RELEASE</version>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
<configuration>
<attach>false</attach>
</configuration>
</execution>
</executions>
...
</plugin>
...
</plugins>
...
</build>
...
</project>
此配置將生成兩個工件:原始工件和由重新打包目標生成的可執行計數器部分。 只會安裝/部署原始的。
您可以通過這種方式禁用 spring-boot-maven 插件。
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<executions>
<execution>
<configuration>
<skip>true</skip>
</configuration>
</execution>
</executions>
</plugin>
創建簡單的 jar 更新
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
至
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<classifier>exec</classifier>
</configuration>
</plugin>
有關更多詳細信息,請訪問以下 Spring URL:- https://docs.spring.io/spring-boot/docs/1.1.2.RELEASE/reference/html/howto-build.html
我找到了答案。 我像@judgingnotjudging 解釋的那樣配置了我的應用程序。 不同之處在於我把它放在了父級-POM 中:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
它阻止了子模塊中 JAR 的默認創建。 我可以通過只在web -Module 中包含它來解決這個問題。
這樣 Spring-Boot 從web -Module 構建一個 fat-JAR,從核心-Module 構建一個簡單的 JAR。
你可以簡單地改變
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
到
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
我不知道它是否仍然相關,但是,需要為 spring-boot-maven-plugin 配置一個簡單的分類器 -
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<classifier>exec</classifier>
</configuration>
</plugin>
</plugins>
</build>
這將生成兩個 jar - 一個是可以作為依賴項包含的普通 jar,另一個作為 exe jar,后綴為“exec”字樣 - 如 test-0.0.1-SNAPSHOT-exec.jar 和 test-0.0 .1-快照.jar
如果您使用嵌入式 tomcat 來運行您的應用程序,您不只是想要一個標准的 Spring Boot fat jar 用於您的 Web 應用程序嗎? 如果是這樣,只需在 pom 中將您的 web 模塊標記為依賴於您的核心模塊,然后構建您的項目。
是否有不同的用例需要將罐子分開?
您是將模塊構建為完全獨立的模塊還是作為單個多模塊項目的一部分? 區別在於后者,您將在根目錄下有一個 pom 指定模塊。 我特別忘記了 Maven 語法,所以我的例子是 Gradle(多模塊構建的 Maven 文檔在這里)。 對此感到抱歉。
baseProject
|----web-module
|----core-module
baseProject 的 build.gradle:
project(':web-module') {
dependencies {
compile project(':core-module')
}
evaluationDependsOn(':core-module')
}
Maven 具有類似的結構。 您應該查看文檔,但我相信您需要做的就是在您的父 pom 中正確指定模塊順序,如下所示,並在您的 web 模塊 pom.xml 中包含依賴項。
父pom:
...
<modules>
<module>core-module</module>
<module>web-module</module>
</modules>
網絡pom:
<project ...>
<parent>
<groupId>com.example</groupId>
<artifactId>simple-parent</artifactId>
<version>1.0</version>
</parent>
<artifactId>web-module</artifactId>
<packaging>war</packaging>
<name>My web-module</name>
<dependencies>
...
<dependency>
<groupId>com.example</groupId>
<artifactId>core-module</artifactId>
<version>1.0</version>
</dependency>
</dependencies>
...
核心 pom 只需要像上面的 web 模塊一樣包含parent
部分,否則是相同的。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.