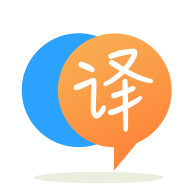
[英]Swift - How to make UIButton tap Interaction only on the image attached and ignore transparent parts
[英]Make only visible parts of png clickable UIbutton swift
嗨,我有一個文件,其中的視圖由10個分層圖像組成,這些圖像是具有大透明部分的png。 我希望只能在可見的部分上單擊它,但是我嘗試過的只是頂住點擊的頂部圖像/按鈕。 我已經嘗試過UIbuttons和可點擊UIImage視圖。 我在2.2版中工作。 有誰知道如何解決這個問題?
下面是一個如何工作的示例。 圖像和點擊手勢已在界面生成器中添加。 此示例只是將分接的視圖顯示在最前面,盡管可以根據您的要求輕松地對其進行修改。
class ClickViewController: UIViewController {
@IBOutlet var images: [UIImageView]!
@IBAction internal func tapGestureHandler(gesture: UITapGestureRecognizer) {
let sorted = images.sort() { a, b in
return a.layer.zPosition < b.layer.zPosition
}
for (i, image) in sorted.enumerate() {
let location = gesture.locationInView(image)
let alpha = image.alphaAtPoint(location)
if alpha > 50.0 {
print("selected image #\(i) \(image.layer.zPosition)")
view.addSubview(image)
return
}
}
}
}
這是一個如何設置情節提要的示例。 注意圖像視圖和輕擊手勢識別器。 也可以以編程方式添加視圖。
在“ 連接”檢查器中的“ 引用出口集合”下,將每個圖像鏈接到images
IBCollection。
這是確定輕擊位置上圖像中像素的alpha分量的代碼。 下面的代碼是對SO上另一個類似問題的解答 。
extension UIImageView {
//
// Return the alpha component of a pixel in the UIImageView.
//
// See: https://stackoverflow.com/questions/27923232/how-to-know-that-if-the-only-visible-area-of-a-png-is-touched-in-xcode-swift-o?rq=1
//
func alphaAtPoint(point: CGPoint) -> CGFloat {
var pixel: [UInt8] = [0, 0, 0, 0]
let colorSpace = CGColorSpaceCreateDeviceRGB();
let alphaInfo = CGImageAlphaInfo.PremultipliedLast.rawValue
guard let context = CGBitmapContextCreate(&pixel, 1, 1, 8, 4, colorSpace, alphaInfo) else {
return 0
}
CGContextTranslateCTM(context, -point.x, -point.y);
layer.renderInContext(context)
let floatAlpha = CGFloat(pixel[3])
return floatAlpha
}
}
@Luke Van謝謝你的回答,這對我有很大幫助。
這是Swift 3版本:(一些語法更改)
import UIKit
class ViewController: UIViewController {
@IBOutlet var images: [UIImageView]!
@IBAction internal func tapGestureHandler(gesture: UITapGestureRecognizer) {
let sorted = images.sorted() { a, b in
return a.layer.zPosition < b.layer.zPosition
}
for (i, image) in sorted.enumerated() {
let location = gesture.location(in: image)
let alpha = image.alphaAtPoint(point: location)
if alpha > 50.0 {
print("selected image #\(i) \(image.layer.zPosition)")
view.addSubview(image)
return
}
}
}
}
extension UIImageView {
func alphaAtPoint(point: CGPoint) -> CGFloat {
var pixel: [UInt8] = [0, 0, 0, 0]
let colorSpace = CGColorSpaceCreateDeviceRGB();
let alphaInfo = CGImageAlphaInfo.premultipliedLast.rawValue
guard let context = CGContext(data: &pixel, width: 1, height: 1, bitsPerComponent: 8, bytesPerRow: 4, space: colorSpace, bitmapInfo: alphaInfo) else {
return 0
}
context.translateBy(x: -point.x, y: -point.y);
layer.render(in: context)
let floatAlpha = CGFloat(pixel[3])
return floatAlpha
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.