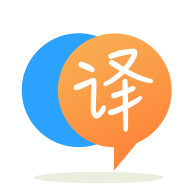
[英]How to set distance between two UITextFields in UIAlertController?
[英]How to set validation in UITextFields
我正在使用一些文本字段和文本字段驗證。 我在代碼下方顯示了此代碼中的單擊按鈕事件,並將所有文本字段文本保存在Web服務器上,在此代碼驗證中僅在空文本字段上運行。 但是我想更正電子郵件地址驗證並固定所有文本字段字符長度。我嘗試了很多次,但有些時候條件錯誤,有些時候沒有顯示警報視圖,有些時候沒有保存文本字段文本。 怎么可能請幫助,謝謝
- (IBAction)submit:(id)sender {
if(self.txname == nil || [self.txname.text isEqualToString:@""])
{
UIAlertView *ErrorAlert = [[UIAlertView alloc] initWithTitle:@"Name"message:@"All Fields are mandatory." delegate:nil cancelButtonTitle:@"OK"otherButtonTitles:nil, nil];
[ErrorAlert show];
}
else if(self.txemail == nil || [self.txemail.text isEqualToString:@""])
{
UIAlertView *ErrorAlert = [[UIAlertView alloc] initWithTitle:@"Email"message:@"All Fields are mandatory." delegate:nil cancelButtonTitle:@"OK"otherButtonTitles:nil, nil];
[ErrorAlert show];
}
else if(self.tx_phone == nil || [self.tx_phone.text isEqualToString:@""])
{
UIAlertView *ErrorAlert = [[UIAlertView alloc] initWithTitle:@"Phone"message:@"All Fields are mandatory." delegate:nil cancelButtonTitle:@"OK"otherButtonTitles:nil, nil];
[ErrorAlert show];
}
else if(self.txcomment == nil || [self.txcomment.text isEqualToString:@""])
{
UIAlertView *ErrorAlert = [[UIAlertView alloc] initWithTitle:@"Comment"message:@"All Fields are mandatory." delegate:nil cancelButtonTitle:@"OK"otherButtonTitles:nil, nil];
[ErrorAlert show];
}
else
{
//Here YOUR URL
NSMutableURLRequest *request = [[NSMutableURLRequest alloc] initWithURL:[NSURL URLWithString:@"MY URL"]];
//create the Method "GET" or "POST"
[request setHTTPMethod:@"POST"];
//Pass The String to server(YOU SHOULD GIVE YOUR PARAMETERS INSTEAD OF MY PARAMETERS)
NSString *userUpdate =[NSString stringWithFormat:@"name=%@&email=%@&phone=%@& comment=%@&",_txname.text,_txemail.text,_tx_phone.text,_txcomment.text,nil];
//Check The Value what we passed
NSLog(@"the data Details is =%@", userUpdate);
//Convert the String to Data
NSData *data1 = [userUpdate dataUsingEncoding:NSUTF8StringEncoding];
//Apply the data to the body
[request setHTTPBody:data1];
//Create the response and Error
NSError *err;
NSURLResponse *response;
NSData *responseData = [NSURLConnection sendSynchronousRequest:request returningResponse:&response error:&err];
NSString *resSrt = [[NSString alloc]initWithData:responseData encoding:NSASCIIStringEncoding];
//This is for Response
NSLog(@"got response==%@", resSrt);
if(resSrt)
{
NSLog(@"got response");
}
else
{
NSLog(@"faield to connect");
}
{
UIAlertView *ErrorAlert = [[UIAlertView alloc] initWithTitle:@"Success"message:@"All Fields are mandatory." delegate:nil cancelButtonTitle:@"OK"otherButtonTitles:nil, nil];
[ErrorAlert show];
}
[self.view endEditing:YES];
self.txname.text=@"";
self.txemail.text=@"";
self.tx_phone.text=@"";
self.txcomment.text=@"";
}
}
喜歡
- (IBAction)submit:(id)sender {
if (![txname hasText]) {
[self showAlertView:@"Alert" message:@"name is empty"];
}
else if (![txemail hasText])
{
[self showAlertView:@"Alert" message:@"email is empty"];
}
else if ([self isValidEmailAddress:txemail.text] == NO)
{
[self showAlertView:@"Alert" message:@"Invaildemail"];
}
else
{
// call webservice for succes
}
創建警報控制器
- (void)showAlertView:(NSString*)title message:(NSString*)message
{
UIAlertController* alertMessage = [UIAlertController
alertControllerWithTitle:title
message:message
preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction* yesButton = [UIAlertAction
actionWithTitle:@"OK"
style:UIAlertActionStyleDefault
handler:^(UIAlertAction* action){
}];
[alertMessage addAction:yesButton];
[self presentViewController:alertMessage animated:YES completion:nil];
}
用於電子郵件驗證
- (BOOL)isValidEmailAddress:(NSString *)emailAddress
{
//Create a regex string
NSString *stricterFilterString = @"[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,4}" ;
//Create predicate with format matching your regex string
NSPredicate *emailTest = [NSPredicatepredicateWithFormat:
@"SELF MATCHES %@", stricterFilterString];
//return true if email address is valid
return [emailTest evaluateWithObject:emailAddress];
}
更新
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string {
if (textField == self.txname)
{
// Prevent crashing undo bug – see note below.
if(range.length + range.location > textField.text.length)
{
return NO;
}
NSUInteger newLength = [textField.text length] + [string length] - range.length;
return newLength <= 25;
}
return YES;
}
我在代碼中做到了,喜歡下面的方式:
- (IBAction)submit:(id)sender {
if (![self isFormValid]) {
return;
}
NSError *error;
if (!error)
{
UIAlertView *signupalert = [[UIAlertView alloc]initWithTitle:@"Congratulations" message:@"Record Added Successfully" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil, nil];
[signupalert show];
}
}
-(BOOL)isFormValid
{
NSString *emailRegEx =@"[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,4}";
NSPredicate *emailTest =[NSPredicate predicateWithFormat:@"SELF MATCHES %@",emailRegEx];
if (txname.text && txname.text.length==0)
{
[self showErrorMessage:@"Please enter name"];
return NO;
}
else if (tx_phone.text && tx_phone.text.length!=10)
{
[self showErrorMessage:@"Please enter valid phone number"];
return NO;
}
else if([emailTest evaluateWithObject: txemail.text]==NO)
{
[self showErrorMessage:@"Please enter Valid Email_id"];
return NO;
}
else if (txcomment.text && txcomment.text.length==0)
{
[self showErrorMessage:@"Please enter comment"];
return NO;
}
return YES;
}
-(void)showErrorMessage:(NSString *)message
{
UIAlertView *alertmessage = [[UIAlertView alloc]initWithTitle:@"Error" message:message delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil, nil];
[alertmessage show];
}
-(BOOL) NSStringIsValidEmail:(NSString *)checkEmail
{
BOOL stricterFilter = NO;
NSString *filter = @"^[A-Z0-9a-z\\._%+-]+@([A-Za-z0-9-]+\\.)+[A-Za-z]{2,4}$";
NSString *lstring = @"^.+@([A-Za-z0-9-]+\\.)+[A-Za-z]{2}[A-Za-z]*$";
NSString *emailRegex = stricterFilter ? stricterFilterString : laxString;
NSPredicate *emailTest = [NSPredicate predicateWithFormat:@"SELF MATCHES %@", emailRegex];
return [emailTest evaluateWithObject:checkEmail];
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.