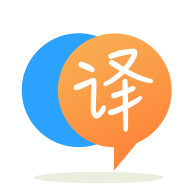
[英]How to create a function using javascript which will check a field value/user input and show alert if the input is correct?
[英]Javascript: How use a loop function to show all the user input in one single alert box?
我正在嘗試編寫一個函數,該函數將為用戶在表單中輸入的所有數據顯示一個警報框。 我必須只使用簡單的javascript(抱歉, 沒有jQuery )來做到這一點。 我的HTML如下:
<form method="POST">
<label class="form">Name: </label><input type="text" name="name" id="name"><br>
<label class="form">Address: </label><input type="text" name="address" id="address"><br>
<label class="form">Email: </label><input type="text" name="email" id="email"><br>
<button id="submit" type="submit" value="submit" onclick="showAlert()">
Submit
</button>
</form>
我的JavaScript:
function showAlert() {
var userInputs = document.getElementsByTagName("input");
for (var i=0; i < userInputs.length; i++) {
alert(userInputs.value + " ");
//Basically my idea would be to implement a loop for as many input fields,
//run through all of them, then display ONE SINGLE alert box containing all the
//data entered by the user. But I'm having trouble with how to implement the loop.
}
}
如何實現循環?
我編寫了另一個實現相同效果的函數,但它涉及為每個輸入字段編寫一長串乏味的變量列表,我不想這樣做,因為它很亂:
function alternateShowAlert() {
var name = document.getElementById('name').value;
var address = document.getElementById('address').value;
var email = document.getElementById('email'.value;
alert(name + " " + address + " " + email)
//This function, although it works fine, will be too long and tedious if I have
//more input fields such as age, city, state, country, gender, etc. I want to put
//it in a loop format but how do I do this?
}
您有邏輯:您需要循環來收集所有信息,但應該只顯示一個警報,因此,除了在循環內發出警報外,您還需要在執行以下操作:
function showAlert() {
var userInputs = document.getElementsByTagName("input");
var infos = ""; // Create a string for the content
for (var i=0; i < userInputs.length; i++) {
infos += userInputs[i].value + " "; // Add the info from user input
}
alert(infos); // Show one alert at the end
}
function showAlert() {
var userInputs = document.getElementsByTagName("input");
var alertBody = "";
for (var i=0; i < userInputs.length; i++) {
alertBody += userInputs[i].value + " ";
}
alert(alertBody);
}
document.getElementsByTagName()
返回一個數組,因此您需要使用它們的索引來訪問該數組的元素: userInputs[i]
。
或者,您可以使用Array.prototype.forEach
。 請參見下面的示例。
function showAlert() {
var userInputs = document.getElementsByTagName("input");
var alertBody = "";
Array.prototype.forEach.call(userInputs, function(element) {
alertBody += element.value + " ";
});
alert(alertBody);
}
您可以單行完成它,沒有任何循環:
function Show() { alert([].slice.call(document.querySelectorAll("input[type='text']")).map(function(x) { return x.id + ": " + x.value; }).join("\\n")); }
Name: <input type="text" id="name" /><br /> Address: <input type="text" id="address" /><br /> Email: <input type="text" id="email" /><br /> <button type="button" onclick="Show();">Show</button>
所有現代瀏覽器(IE 9+)都支持querySelectorAll
方法,它是與jQuery選擇器等效的普通JS。 但是,它返回一個節點列表,該列表應轉換為純數組 ,以便我們使用所需的.map()
方法輕松獲取所有值。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.