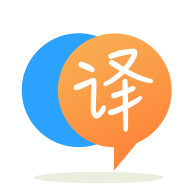
[英]AWS SES Email using boto3 through python lambda function
[英]Send formatted html email via AWS SES with Python and boto3
我正在嘗試設置電子郵件功能以發送帶有格式化數據的電子郵件。 我有以下內容可用於在電子郵件中發送pandas數據幀,但用於將pandas數據幀轉換為html的格式不會通過用戶定義的空格數來發送。
#install the AWS SES SDK for Python (boto3) from the command line
#pip install boto3
import boto3
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.randn(10, 4), columns=['A', 'B', 'C', 'D']) * 10000
def email(bodytext = 'No data...check your function arguments', dftoconvert = None):
region = 'us-west-2'
user = '' #insert your access key to use when creating the client
pw = '' #insert the secret key to use when creating the client
client = boto3.client(service_name = 'ses',
region_name = region,
aws_access_key_id = user,
aws_secret_access_key = pw)
me = 'me@example.com'
you = ['you@example.com']
subject = 'testSUBJECT'
COMMASPACE = ', '
you = COMMASPACE.join(you)
#Build email message parts
#Build and send email
destination = { 'ToAddresses' : [you],
'CcAddresses' : [],
'BccAddresses' : []}
try:
bodyhtml = dftoconvert.to_html(float_format = lambda x: '({:15,.2f})'.format(abs(x)) if x < 0 else '+{:15,.2f}+'.format(abs(x)))
message = {'Subject' : {'Data' : subject},
'Body': {'Html' : {'Data' : bodyhtml}}}
except NoneType: #If there is no data to convert to html
message = {'Subject' : {'Data' : subject},
'Body': {'Text' : {'Data' : bodytext}}}
except Exception as e:
print(e)
result = client.send_email(Source = me,
Destination = destination,
Message = message)
return result if 'ErrorResponse' in result else ''
print(df)
email(dftoconvert = df)
出於某種原因,空格被忽略。 我已經將有效數字標識符更改為非常小且非常大,並且html輸出看起來正確,並且Python中的浮點數具有正確的前導空格數。 見下文。
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.randn(10, 4), columns=['A', 'B', 'C', 'D']) * 10000
df
A B C D
0 1071.786803 -4354.776685 -3541.261466 2653.522461
1 489.865060 -12567.822512 13888.890274 14243.027303
2 471.995980 9473.174725 -1776.897694 5085.236174
3 5932.486256 -12558.720083 -17714.593696 -3503.574051
4 -8886.624311 -10286.622739 -4513.326771 2714.793954
5 5965.944055 10207.608141 19224.094501 4748.746867
6 14189.480430 -13826.251008 9847.711830 -1241.976560
7 -9044.406158 -14337.121541 19572.135090 -18146.356528
8 3057.233113 -14410.383480 -931.179975 -16273.711970
9 -14492.047676 -1150.506849 -1892.032700 -797.596310
df.to_html(float_format = lambda x: '({:15,.2f})'.format(abs(x)) if x < 0 else '+{:15,.2f}+'.format(abs(x)))
Out[3]: '<table border="1" class="dataframe">\\n <thead>\\n <tr style="text-align: right;">\\n <th></th>\\n <th>A</th>\\n <th>B</th>\\n <th>C</th>\\n <th>D</th>\\n </tr>\\n </thead>\\n <tbody>\\n <tr>\\n <th>0</th>\\n <td>+ 1,071.79+</td>\\n <td>( 4,354.78)</td>\\n <td>( 3,541.26)</td>\\n <td>+ 2,653.52+</td>\\n </tr>\\n <tr>\\n <th>1</th>\\n <td>+ 489.87+</td>\\n <td>( 12,567.82)</td>\\n <td>+ 13,888.89+</td>\\n <td>+ 14,243.03+</td>\\n </tr>\\n <tr>\\n <th>2</th>\\n <td>+ 472.00+</td>\\n <td>+ 9,473.17+</td>\\n <td>( 1,776.90)</td>\\n <td>+ 5,085.24+</td>\\n </tr>\\n <tr>\\n <th>3</th>\\n <td>+ 5,932.49+</td>\\n <td>( 12,558.72)</td>\\n <td>( 17,714.59)</td>\\n <td>( 3,503.57)</td>\\n </tr>\\n <tr>\\n <th>4</th>\\n <td>( 8,886.62)</td>\\n <td>( 10,286.62)</td>\\n <td>( 4,513.33)</td>\\n <td>+ 2,714.79+</td>\\n </tr>\\n <tr>\\n <th>5</th>\\n <td>+ 5,965.94+</td>\\n <td>+ 10,207.61+</td>\\n <td>+ 19,224.09+</td>\\n <td>+ 4,748.75+</td>\\n </tr>\\n <tr>\\n <th>6</th>\\n <td>+ 14,189.48+</td>\\n <td>( 13,826.25)</td>\\n <td>+ 9,847.71+</td>\\n <td>( 1,241.98)</td>\\n </tr>\\n <tr>\\n <th>7</th>\\n <td>( 9,044.41)</td>\\n <td>( 14,337.12)</td>\\n <td>+ 19,572.14+</td>\\n <td>( 18,146.36)</td>\\n </tr>\\n <tr>\\n <th>8</th>\\n <td>+ 3,057.23+</td>\\n <td>( 14,410.38)</td>\\n <td>( 931.18)</td>\\n <td>( 16,273.71)</td>\\n </tr>\\n <tr>\\n <th>9</th>\\n <td>( 14,492.05)</td>\\n <td>( 1,150.51)</td>\\n <td>( 1,892.03)</td>\\n <td>( 797.60)</td>\\n </tr>\\n </tbody>\\n</table>'
但是電子郵件似乎忽略了任何前導空格,並且無論前導空格有多少,都以相同的方式顯示html。 我無法弄清楚如何在電子郵件中顯示html格式,就像html出現在Python輸出中一樣(帶有前導空格)。 有沒有更好的辦法? 是否有比html更好的格式來通過電子郵件格式化數據幀?
我假設你想在表格單元格中使用前導空格來格式化電子郵件中的表格。 這將無效,因為空白將被合並。
你可以用不間斷的空間替換兩個空格,它會使郵件看起來有點不同,但不是很理想。
def email(bodytext = 'No data...check your function arguments', dftoconvert = None, replace=False):
region = 'us-west-2'
user = '' #insert your access key to use when creating the client
pw = '' #insert the secret key to use when creating the client
client = boto3.client(service_name = 'ses',
region_name = region,
aws_access_key_id = user,
aws_secret_access_key = pw)
me = 'me@example.com'
you = ['you@example.com']
subject = 'testSUBJECT'
COMMASPACE = ', '
you = COMMASPACE.join(you)
#Build email message parts
#Build and send email
destination = { 'ToAddresses' : [you],
'CcAddresses' : [],
'BccAddresses' : []}
try:
bodyhtml = dftoconvert.to_html(float_format = lambda x: '({:15,.2f})'.format(abs(x)) if x < 0 else '+{:15,.2f}+'.format(abs(x)))
# use no-break space instead of two spaces next to each other
if replace:
bodyhtml = bodyhtml.replace(' ', ' ')
message = {'Subject' : {'Data' : subject},
'Body': {'Html' : {'Data' : bodyhtml}}}
except NoneType: #If there is no data to convert to html
message = {'Subject' : {'Data' : subject},
'Body': {'Text' : {'Data' : bodytext}}}
except Exception as e:
print(e)
result = client.send_email(Source = me,
Destination = destination,
Message = message)
return result if 'ErrorResponse' in result else ''
email(dftoconvert=df)
email(dftoconvert=df, replace=True)
結果如下:
並替換空間:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.