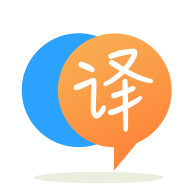
[英]How to mapping two table (one to many )using hibernate annotation + Spring MVC
[英]How to data bind to CheckBox using Many to many annotation in Spring MVC
我想這樣做;
我有一項工作可以改進CRUD應用程序,以學習Spring,Hibernate和JPA。 所以我想做很多對很多的例子,但是我請某人去做。
我想獲得員工的駕駛執照並更新信息。 我可以使用Spring forEach將員工的信息從數據庫中獲取到網頁中的CheckBox。 如果員工擁有哪個駕駛執照,則必須選中關系復選框。 我可以更新此信息。
例如,員工獲得了“ A1”和“ M”類的駕駛執照。 我可以獲取並綁定數據。
我創建了“員工”,“ driving_licenses”表。 我為商店員工的駕駛執照創建了“ employee_driving_licenses”表:
雇員:
id int
last_name nvarchar(20)
first_name nvarchar(10)
駕駛執照:
id int
code nvarchar(5)
employee_driving_licenses:
employee_id int
driving_license_id int
我創建了表的模型類,並添加了JPA批注。 我有Employee和DrivingLicense Entity Java類:
Employee.java
@Entity
@Table(name = "employees")
public class Employee {
private int id;
private String last_name;
private String first_name;
private java.util.Collection<DrivingLicense> driving_licenses = new HashSet<DrivingLicense>();
@GeneratedValue(strategy = GenerationType.AUTO)
@Id
@Column
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
@NotEmpty(message = "Please enter Last Name")
@Column
public String getLast_name() {
return last_name;
}
public void setLast_name(String last_name) {
this.last_name = last_name;
}
@NotEmpty(message = "Please enter First Name")
@Column
public String getFirst_name() {
return first_name;
}
@ManyToMany(fetch = FetchType.EAGER, mappedBy = "employees")
public java.util.Collection<DrivingLicense> getDriving_licenses() {
return driving_licenses;
}
public void setDriving_licenses(
java.util.Collection<DrivingLicense> driving_licenses) {this.driving_licenses = driving_licenses;
}
}
DrivingLicense.java
@Entity
@Table(name = "driving_licenses")
public class DrivingLicense {
private int id;
private String code;
private java.util.Collection<Employee> employees = new HashSet<Employee>();
@Override
public int hashCode() {
return new Long(id).hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (!(obj instanceof DrivingLicense)) {
return false;
}
return this.id == ((DrivingLicense) obj).getId();
}
@GeneratedValue(strategy = GenerationType.AUTO)
@Id
@Column
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
@Column
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
@ManyToMany(fetch = FetchType.EAGER, cascade = { CascadeType.PERSIST, CascadeType.MERGE })
@JoinTable(name = "employee_driving_licenses", joinColumns = { @JoinColumn(name = "driving_license_id", nullable = false, updatable = false) }, inverseJoinColumns = { @JoinColumn(name = "employee_id", nullable = false, updatable = false) })
public java.util.Collection<Employee> getEmployees() {
return employees;
}
public void setEmployees(java.util.Collection<Employee> employees) {
this.employees = employees;
}
}
我創建了應用程序DAO,服務,控制器和視圖類/頁面。
EmployeeController.java:
@RequestMapping(value = "index", method = RequestMethod.POST)
public String employee(@Valid @ModelAttribute("employee") Employee employee,
BindingResult result, @RequestParam String action,
java.util.Map<String, Object> map) {
boolean isValid = true;
if (result.hasErrors()) {
isValid = false;
}
Employee employeeResult = new Employee();
switch (action.toLowerCase()) {
case "add":
if (isValid)
employeeService.add(employee);
employeeResult = employee;
break;
case "edit":
if (isValid)
employeeService.edit(employee);
employeeResult = employee;
break;
}
map.put("drivingLicenseList", drivingLicenseService.findActiveDrivingLicense());
map.put("employee", employeeResult);
map.put("activeEmployeeList", employeeService.findActiveEmployees());
return "employee";
}
employee.jsp:
<c:forEach var="drive_licenses" items="${drivingLicenseList}">
<form:checkbox path="driving_licenses"
value="${drive_licenses }" label="${drive_licenses.code }" />
</c:forEach>
當我想更新員工的駕駛執照時,我收到如下錯誤代碼:
{employee=com.ay.model.Employee@573cb29c, org.springframework.validation.BindingResult.employee=org.springframework.validation.BeanPropertyBindingResult: 1 errors
Field error in object 'employee' on field 'driving_licenses': rejected value [com.ay.model.DrivingLicense@1,com.ay.model.DrivingLicense@7,com.ay.model.DrivingLicense@8,com.ay.model.Driving License@9]; codes [typeMismatch.employee.driving_licenses,typeMismatch.driving_licenses,typeMismatch.java.util.Collection,typeMis match]; arguments [org.springframework.context.support.DefaultMessageSourceResolvable: codes [employee.driving_licenses,driving_licenses]; arguments []; default message [driving_licenses]]; default message [Failed to convert property value of type 'java.lang.String[]' to required type 'java.util.Collection' for property 'driving_licenses'; nested exception is java.lang.IllegalStateException: Cannot convert value of type [java.lang.String] to required type [com.ay.model.DrivingLicense] for property 'driving_licenses[0]': no matching editors or conversion strategy found]}
如何獲取Collection類型的值? 請給我建議。
而且,如果我添加此代碼
value="${drive_licenses }"
在employee.jsp的網頁上,我可以正常在瀏覽器上看到對象值。 其實首先我添加了這段代碼
value="${drive_licenses.id }"
但是沒有在CheckBoxes上檢查員工的駕駛執照,並且每個CheckBoxes值都為空。 真正的語法是什么?
感謝您的支持。
這個部分太多了。 大致:
一 :創建包裝表格:
public class EmployeeForm{
private Employee employee;
//Driving licence needs a 'boolean' selected field
//Mark as @Transient so not persisted
//Otherwise create another wrapper/dto which has this boolean field
//Spring MVC can only bind to indexed fields e.g. List
private List<DrivingLicence> allAvailableLicences;
public EmployeeForm(Employee employee, Collecion<DrivingLicence> licenes){
//set instance vars
}
//getters
//setters
}
二 :將以下內容添加到您的控制器中:
//CALLED ON BOTH GET AND POST TO SET UP THE FORM
//FOR EDITING EXISTING EMPLOYEE REPLACE NEW EMPLOYEE WITH THE RELEVANT LOOKUP
@ModelAttribute("employeeForm")
public EmployeeForm getEmployeeForm(@RequestParam("employeeId", required = false) Long employeeId){
return new EmployeeForm(new Employee(), drivingLicenseService.findActiveDrivingLicense());
}
三 :
更改您的JSP表單以綁定到employeeForm:可以在路徑屬性中使用嵌套屬性,例如employee.name。 對於綁定許可證,您需要使用以下索引符號,即將列表中索引x處的許可證設置為選中。
<c:forEach var="licence" items="${employeeForm.allAvailableLicences}" varStatus="loop">
<form:checkbox path="licence[${loop.index]}.selected" label="${licence.code }" />
</c:forEach>
四:
更新您的表單提交處理程序:
@RequestMapping(value = "index", method = RequestMethod.POST)
public String employee(@Valid @ModelAttribute("employeeForm") EmployeeForm employeeForm,
BindingResult result, @RequestParam String action) {
//validation etc
Employee employee = employeeForm.getEmployee();
for(DrivingLicence licence : employeeForm.getAllAvailableLicences()){
//our indexed foem check box set the 'selected' field true
//if selected add to the employee
}
//save updated employee
return "nextPage";
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.