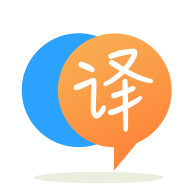
[英]note: expected 'char *' but argument is of type 'int *'
[英]note: expected ‘int * (*)()’ but argument is of type ‘int’
不寫任何代碼對我來說是不是太長了。 我嘗試用 C 編寫一個簡單的演示,但在下面遇到,
1 main.c|87 col 21 warning| passing argument 2 of ‘Logger’ makes pointer from integer without a cast [-Wint-conversion]
2 logger.h|24 col 6 error| note: expected ‘int * (*)()’ but argument is of type ‘int’
我定義了一個函數
void Logger(int priority, int errno, const char* fmt, ...);
Logger 的第二個參數是 int 類型。 但是,當我想在我的主進程中使用它時,
Logger(LOG_ERR, errno, "ERROR: select failed");
它告訴我第二個參數的預期類型是 'int * (*)()' 。 在我對該函數的調用中,第二個實際參數 errno 來自 errno.h
代碼如下 1.logger.c
// logger.c
#include <stdlib.h>
#include <string.h>
#include <stdarg.h>
#include <syslog.h>
#include <error.h>
#include "logger.h"
char LogLastMsg[128]; // all info of the last log, all the info to log last time
int Log2Stderr = LOG_INFO; //control Loging to stderr
void init_logger(char* logfile_path, FILE* logfile)
{
logfile = fopen(logfile_path, "w+");
if ( NULL == logfile )
{
fprintf(stderr, "Failed to init logger");
exit(-1);
}
}
void end_logger(FILE *logfile)
{
fclose(logfile);
}
/**
* @Synopsis a log func demo
* demo for how user defined module log info
*
* @Param priority: level of log, LOG_ERR, LOG_DEBUG etc.
* @Param errno: errno
* @Param fmt: format of message to log
* @Param ...: args follow by fmt
*/
void Logger(int priority, int errno, char* fmt, ...)
{
char priVc[][8] = {"Emerg", "Alert", "Crit", "Error", "Warning", "Notice", "Info", "Debug"};
char* priPt = priority < 0 || priority >= sizeof(priVc)/sizeof(priVc[0]) ?
"Unknow priority!" : priVc[priority];
char *errMsg = errno <= 0 ? NULL : strerror(errno);
{
va_list argPt;
unsigned Ln;
va_start(argPt, fmt); //now argPt is point to mylog's param:...
Ln = snprintf(LogLastMsg, sizeof(LogLastMsg), "[mylog...][%s]: ", priPt);
Ln += vsnprintf(LogLastMsg + Ln, sizeof(LogLastMsg) - Ln, fmt, argPt);
if (NULL != errMsg)
{
Ln += snprintf(LogLastMsg + Ln, sizeof(LogLastMsg) - Ln, "%d:%s", errno, errMsg);
}
va_end(argPt);
}
//choose the log which should be show on stderr
if (priority < LOG_ERR || priority <= Log2Stderr)
{
fprintf(stderr, "%s", LogLastMsg);
}
//always to syslog
syslog(priority, "%s", LogLastMsg);
if (priority <= LOG_ERR)
{
exit(-1);
}
return ;
}
/**
* @file main.c
* @synopsis
* @author Windeal, lipeilun4it@outlook.com
* @version 0.1
* @date 2016-09-12
*/
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <ctype.h>
#include <getopt.h>
#include <syslog.h>
#include <errno.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include "http_proxy.h"
#include "logger.h"
#include "socketapi.h"
#define LOG_FILE "http_proxy.log"
typedef struct Server{
struct sockaddr_in addr;
short port;
} Server;
typedef struct Client{
struct sockaddr_in addr;
int len;
} Client;
static int max_int(int a, int b)
{
int ret;
ret = a;
if ( ret < 0 )
{
ret = b;
}
return ret;
}
static int get_global_option(int argc, char **argv);
static int init_httpd_socket(int *sockfd);
int main(int argc, char **argv)
{
FILE *logfile;
int sockfd, clientfd;
Server server;
Client client;
fd_set rfds;
int max_fd;
struct timeval tv;
int retval = -1;
init_logger(LOG_FILE, logfile);
Logger(LOG_INFO, 0, "httpd start...\n");
init_httpd_socket(&sockfd);
while (1)
{
client.len = sizeof (struct sockaddr);
clientfd = Accept(sockfd, (struct sockaddr *)&client.addr, &client.len);
Logger(LOG_INFO, 0, "A new connection accept!");
while (1)
{
FD_ZERO(&rfds);
FD_SET(clientfd, &rfds);
max_fd = max_int(0, clientfd);
tv.tv_sec = 1;
tv.tv_usec = 0;
retval = select(max_fd+1, &rfds, NULL, NULL, &tv);
if ( retval == -1 )
{
Logger(LOG_ERR, 3, "ERROR: select failed");
break;
}
else if ( retval == 0 )
{
// printf("continue waiting!\n");
continue;
}
}
}
}
/**
* @synopsis init_httpd_socket, create a new socket for server
* @param sockfd
* @returns a new socket for server
*/
static int init_httpd_socket(int *sockfd)
{
struct sockaddr_in serv_addr;
short port;
Logger(LOG_INFO, 0, "init_http_socket...");
port = htons(SERV_PORT);
*sockfd = Socket(AF_INET, SOCK_STREAM, 0);
memset(&serv_addr, 0, sizeof (serv_addr));
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = port;
serv_addr.sin_addr.s_addr = htonl(INADDR_ANY);
Bind(*sockfd, (struct sockaddr*)&serv_addr, sizeof (serv_addr));
Listen(*sockfd, 6);
return *sockfd;
}
現在,我知道答案了。 錯誤應該在 Logger 的聲明中。
void Logger(int priority, int errno, char* fmt, ...);
字符串 'errno' 似乎不能是形式參數。 我用'Errno'來代替它,然后它就可以正常工作了。
我認為字符串 'errno' 可能是 errno.h 中定義的宏。 輸入“man errno”可能會得到更多信息。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.