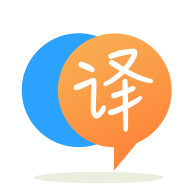
[英]Returned null values when parsing JSON data from URL using Jackson
[英]Parsing Json Data from Url- Null Values Return - Java
我正在使用Eclipse IDE從URl解析Json數據,但是每當我運行此代碼時,它都會返回空值。
這是來自URL的json數據
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
這是POJO類
package com.beto.test.json;
public class Data {
private String id;
private String body;
private String title;
private String userId;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
@Override
public String toString() {
return "ClassPojo [id = " + id + ", body = " + body + ", title = " + title + ", userId = " + userId + "]";
}
}
JsonParserUrl.java類
package com.beto.test.json;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.List;
import java.util.Objects;
/**
* Created by BeytullahC on 23.03.2015.
*/
public class JsonParserFromUrl {
public static void main(String[] args) {
DefaultHttpClient httpClient = null;
try {
httpClient = new DefaultHttpClient();
HttpResponse response = getResponse("http://jsonplaceholder.typicode.com/posts", httpClient);
String outPut = readData(response);
System.out.println(outPut);
Gson gson = new Gson();
List<Data> fromJson = gson.fromJson(outPut, new TypeToken<List<Data>>(){}.getType());
System.out.println("DATA SIZE : "+fromJson.size());
System.out.println("GET FIRST DATA : "+fromJson.get(0));
} catch (Exception e) {
e.printStackTrace();
} finally {
httpClient.getConnectionManager().shutdown();
}
}
public static HttpResponse getResponse(String url, DefaultHttpClient httpClient) throws IOException {
try {
HttpGet httpGet = new HttpGet(url);
httpGet.addHeader("accept", "application/json");
HttpResponse response = httpClient.execute(httpGet);
return response;
} catch (IOException e) {
throw e;
}
}
public static String readData(HttpResponse response) throws Exception {
BufferedReader reader = null;
try {
reader = new BufferedReader(new InputStreamReader(
response.getEntity().getContent()));
StringBuffer data = new StringBuffer();
char[] dataLength = new char[1024];
int read;
while (((read = reader.read(dataLength)) != -1)) {
data.append(dataLength, 0, read);
}
return data.toString();
} finally {
if (reader != null)
reader.close();
}
}
}
TestRunner.java類
package com.beto.test.json;
public class TestRunner {
public static void main(String[] args) {
// TODO Auto-generated method stub
try{
Data data = JsonParserfromUrl.getData("http://jsonplaceholder.typicode.com/posts/1");
System.out.println("result:\n" +data);
}
catch(Exception e)
{
System.out.println("error");
}
}}
自由主義者增加了:
- gson-2.3.1.jar
- httpclient-4.5.jar
- commons-logging-1.1.1.jar
- httpclient-cache-4.4.jar
- httpmime-4.0.jar
- commons-codec-1.1.jar
- httpcore-4.4.jar
輸出值
ClassPojo [id = " null ", body = " null ", title = " null ", userId ="null"]
請解決此問題,它不應返回空值。
首先,您應該獲取JSON字符串,然后將其解析為Data類。
String yourJsonString = readUrl("http://jsonplaceholder.typicode.com/posts/1");
Gson gson = new Gson();
Data data = gson.fromJson(yourJsonString, Data.class);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.