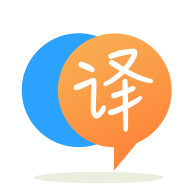
[英]Could you please verify if my code is correct for saving string to struct?
[英]How to use Mmap to share memory. Please correct My code
這里的問題是我希望我們的put為93。我希望所有線程共享該變量。 就像靜態變量是所有對象通用的一樣,我想要一個所有線程都通用的變量。
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <unistd.h>
static int *glob_var;
int main(void)
{
glob_var = (int*)mmap(NULL, sizeof *glob_var, PROT_READ | PROT_WRITE,
MAP_SHARED | MAP_ANONYMOUS, -1, 0);
*glob_var = 1;
int ppid =fork();
if (ppid == 0) {
*glob_var = 92; printf("%d\n", *glob_var);
} else if(ppid!=0){
(*glob_var)++; /////I want a 93 over here???
printf("%d\n", *glob_var); /////I want a 93 over here??? print
munmap(glob_var, sizeof *glob_var);
}
return 0;
}
這兩個過程都更新glob_var
。 您需要協調對該共享內存的訪問。 必須保證正確的數據修改順序,即應首先分配值92
。
在以下情況下, 信號燈通常用於同步操作:
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#include <unistd.h>
// Binary semaphore implementation. Initial state 0
union semun
{
int val;
struct semid_ds *buf;
unsigned short int *array;
struct seminfo *__buf;
};
int binary_semaphore_allocation (key_t key, int sem_flags)
{
return semget (key, 1, sem_flags);
}
int binary_semaphore_deallocate (int semid)
{
union semun ignored_argument;
return semctl (semid, 1, IPC_RMID, ignored_argument);
}
int binary_semaphore_initialize (int semid)
{
union semun argument;
unsigned short values[1];
values[0] = 0;
argument.array = values;
return semctl (semid, 0, SETALL, argument);
}
int binary_semaphore_wait (int semid)
{
struct sembuf operations[1];
operations[0].sem_num = 0;
/* Decrement by 1. */
operations[0].sem_op = -1;
operations[0].sem_flg = SEM_UNDO;
return semop (semid, operations, 1);
}
int binary_semaphore_post (int semid)
{
struct sembuf operations[1];
operations[0].sem_num = 0;
/* Increment by 1. */
operations[0].sem_op = 1;
operations[0].sem_flg = SEM_UNDO;
return semop (semid, operations, 1);
}
int main(void)
{
key_t ipc_key;
ipc_key = ftok(".", 'S');
int sem_id;
glob_var = (int*)mmap(NULL, sizeof *glob_var, PROT_READ | PROT_WRITE,
MAP_SHARED | MAP_ANONYMOUS, -1, 0);
*glob_var = 1;
if ((sem_id=binary_semaphore_allocation(ipc_key, IPC_CREAT|IPC_EXCL)) != -1)
{
if (binary_semaphore_initialize(sem_id) == -1)
{
printf("Semaphore initialization failed");
return 2;
}
}
int ppid = fork();
if (ppid == 0)
{
if ((sem_id = binary_semaphore_allocation(ipc_key, 0)) == -1)
{
printf("Child process failed to open semaphore");
return 3;
}
}
else
{
// Wait in parent process until child update glob_var
binary_semaphore_wait(sem_id);
}
if (ppid == 0)
{
*glob_var = 92;
printf("%d\n", *glob_var);
binary_semaphore_post(sem_id);
}
else if(ppid!=0)
{
(*glob_var)++;
printf("%d\n", *glob_var);
munmap(glob_var, sizeof *glob_var);
binary_semaphore_deallocate(sem_id);
}
return 0;
}
輸出:
92
93
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.