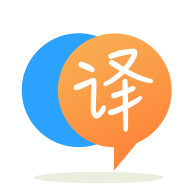
[英]Getting an error, 'Can't convert from BSON type objectId to String' when using MongoDB aggregation
[英]pass the mongodb bson.Objectid from JavaScript(fronend) to Go(backend)
我想通過檢查對象ID來更新MongoDB對象屬性值。 那種類型是bson.Objectid。 我正在使用mgo.v2
MongoDB Golang驅動程序。 但在這種情況下,我將PUT
請求發送到更新API。 我將object id
HEX值作為字符串發送到Golang API。 但是在將HEX值解碼為bson.Object
類型變量時,我的Golang方面發生了錯誤。
我該如何正確地做到這一點。
前端 :
HEXVALUE = "57f54ef4d6e0ac55f6c7ff24"
var widget = {id: HEXVALUE, position: 2, type: 2, class: "normal"};
$.ajax({
url: 'api/widget',
type: 'PUT',
contentType: "application/json",
data: JSON.stringify(widget),
success: function (data) {
console.log(data);
},
error: function (e) {
console.log(e);
}
});
走邊(服務器端):
type Widget struct {
Id bson.ObjectId `json:"id" bson:"_id"`
Position int `json:"position" bson:"position"`
Type int `json:"type" bson:"type"`
Class string `json:"class" bson:"class"`
}
func UpdateWidget(w http.ResponseWriter, r *http.Request) (error) {
decoder := json.NewDecoder(r.Body)
var widget models.DashboardWidget
err := decoder.Decode(&widget)
if err != nil {
log.Error("There is error happening decoding widget: %v", err);
return err;
}
reurn nil
};
產量
log error : There is error happening decoding widget
如何將十六進制值解碼為bson.ObjectId
類型。
1使用以下方法將十六進制值解碼為bson.ObjectId
類型:
bson.ObjectIdHex("57f54ef4d6e0ac55f6c7ff24")
2-要通過檢查對象ID來更新MongoDB對象屬性值,您可以使用
c.Update(bson.M{"_id": widget.Id}, widget)
這是工作代碼:
package main
import (
"encoding/json"
"fmt"
"log"
"net/http"
"strings"
"gopkg.in/mgo.v2"
"gopkg.in/mgo.v2/bson"
)
func main() {
session, err := mgo.Dial("localhost")
if err != nil {
panic(err)
}
defer session.Close()
session.SetMode(mgo.Monotonic, true) // Optional. Switch the session to a monotonic behavior.
c := session.DB("test").C("Widget")
c.DropCollection()
err = c.Insert(
&Widget{bson.NewObjectId(), 1, 2, "c1"},
&Widget{bson.NewObjectId(), 20, 2, "normal"},
&Widget{bson.ObjectIdHex("57f54ef4d6e0ac55f6c7ff24"), 2, 2, "normal"})
if err != nil {
log.Fatal(err)
}
result := []Widget{}
err = c.Find(bson.M{}).All(&result)
if err != nil {
log.Fatal(err)
}
fmt.Println(result)
s := `{"id":"57f54ef4d6e0ac55f6c7ff24","position":2,"type":2,"class":"normal"}`
decoder := json.NewDecoder(strings.NewReader(s))
var widget Widget
err = decoder.Decode(&widget)
if err != nil {
panic(err)
}
fmt.Println(widget)
c.Update(bson.M{"_id": widget.Id}, widget)
result2 := []Widget{}
err = c.Find(bson.M{}).All(&result2)
if err != nil {
log.Fatal(err)
}
fmt.Println(result2)
//http.HandleFunc("/", UpdateWidget)
//http.ListenAndServe(":80", nil)
}
func UpdateWidget(w http.ResponseWriter, r *http.Request) {
decoder := json.NewDecoder(r.Body)
var widget Widget //models.DashboardWidget
err := decoder.Decode(&widget)
if err != nil {
log.Fatal(err)
}
}
type Widget struct {
Id bson.ObjectId `json:"id" bson:"_id"`
Position int `json:"position" bson:"position"`
Type int `json:"type" bson:"type"`
Class string `json:"class" bson:"class"`
}
輸出:
[{ObjectIdHex("57f62973c3176903402adb5e") 1 2 c1} {ObjectIdHex("57f62973c3176903402adb5f") 20 2 normal} {ObjectIdHex("57f54ef4d6e0ac55f6c7ff24") 2 2 normal}]
{ObjectIdHex("57f54ef4d6e0ac55f6c7ff24") 2 2 normal}
[{ObjectIdHex("57f62973c3176903402adb5e") 1 2 c1} {ObjectIdHex("57f62973c3176903402adb5f") 20 2 normal} {ObjectIdHex("57f54ef4d6e0ac55f6c7ff24") 2 2 normal}]
3-您的代碼有一些拼寫錯誤:
日志錯誤:解碼小部件發生錯誤
這個錯誤屬於
err := decoder.Decode(&widget)
把它改成這個:
err := decoder.Decode(&widget)
if err != nil {
log.Fatal(err)
}
看到確切的錯誤。
更改
var widget models.DashboardWidget
至
var widget Widget
像這樣:
func UpdateWidget(w http.ResponseWriter, r *http.Request) {
decoder := json.NewDecoder(r.Body)
var widget Widget //models.DashboardWidget
err := decoder.Decode(&widget)
if err != nil {
log.Fatal(err)
}
}
和
JSON.stringify(widget)
產生:
`{"id":"57f54ef4d6e0ac55f6c7ff24","position":2,"type":2,"class":"normal"}`
這很好用:
package main
import (
"encoding/json"
"fmt"
"strings"
"gopkg.in/mgo.v2/bson"
)
func main() {
s := `{"id":"57f54ef4d6e0ac55f6c7ff24","position":2,"type":2,"class":"normal"}`
decoder := json.NewDecoder(strings.NewReader(s))
var widget Widget
err := decoder.Decode(&widget)
if err != nil {
panic(err)
}
fmt.Println(widget)
}
type Widget struct {
Id bson.ObjectId `json:"id" bson:"_id"`
Position int `json:"position" bson:"position"`
Type int `json:"type" bson:"type"`
Class string `json:"class" bson:"class"`
}
輸出:
{ObjectIdHex("57f54ef4d6e0ac55f6c7ff24") 2 2 normal}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.