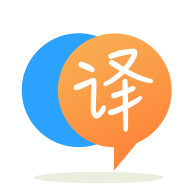
[英]How to iterate through an ArrayList of Objects of ArrayList of Objects?
[英]How to iterate through ArrayList of objects?
我有一個名為SparseMatrix的類。 它包含節點的ArrayList(也是類)。 我想知道如何遍歷數組並訪問Node中的值。 我嘗試過以下方法:
//Assume that the member variables in SparseMatrix and Node are fully defined.
class SparseMatrix {
ArrayList filled_data_ = new ArrayList();
//Constructor, setter (both work)
// The problem is that I seem to not be allowed to use the operator[] on
// this type of array.
int get (int row, int column) {
for (int i = 0; i < filled_data_.size(); i++){
if (row * max_row + column == filled_data[i].getLocation()) {
return filled_data[i].getSize();
}
}
return defualt_value_;
}
}
我可能會切換到靜態數組(並在每次添加對象時重新創建它)。 如果有人有解決方案,我非常感謝您與我分享。 另外,謝謝你提前幫助我。
如果您對此無法理解,請隨時提出問題。
假設filled_data_是一個包含名為Node的類的對象列表的列表。
List<Nodes> filled_data_ = new ArrayList<>();
for (Node data : filled_data_) {
data.getVariable1();
data.getVariable2();
}
更多信息http://crunchify.com/how-to-iterate-through-java-list-4-way-to-iterate-through-loop/
首先,您不應該使用原始類型。 有關詳細信息,請參閱此鏈接: 什么是原始類型,為什么我們不應該使用它?
修復是聲明數組列表所包含的對象類型。 將聲明更改為:
ArrayList<Node> filled_data_ = new ArrayList<>();
然后,您可以使用filled_data_.get(i)
訪問數組列表中的每個元素(而不是filled_data_[i]
,這將適用於常規數組)。
`filled_data_.get(i)`
以上將返回索引i
處的元素。 文檔: https : //docs.oracle.com/javase/7/docs/api/java/util/ArrayList.html#get(int)
如果您沒有使用泛型,那么您需要轉換對象
//Assume that the member variables in SparseMatrix and Node are fully defined.
class SparseMatrix {
ArrayList filled_data_ = new ArrayList();
//Constructor, setter (both work)
// The problem is that I seem to not be allowed to use the operator[] on
// this type of array.
int get (int row, int column) {
for (int i = 0; i < filled_data_.size(); i++){
Node node = (Node)filled_data.get(i);
if (row * max_row + column == node.getLocation()) {
return node.getSize();
}
}
return defualt_value_;
}
}
如果數組列表包含定義getLocation()
Nodes
,則可以使用:
((Nodes)filled_data_.get(i)).getLocation()
你也可以定義
ArrayList<Nodes> filled_data_ = new ArrayList<Nodes>();
創建ArrayList
對象時,應使用<>
括號指定包含元素的類型。 保持對List
接口的引用也很好 - 而不是ArrayList
類。 要遍歷這樣的集合,請使用foreach
循環:
以下是Node類的示例:
public class Node {
private int value;
public Node(int value) {
this.value = value;
}
public void setValue(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
以下是Main類的示例:
public class Main {
public static void main(String[] args) {
List<Node> filledData = new ArrayList<Node>();
filledData.add(new Node(1));
filledData.add(new Node(2));
filledData.add(new Node(3));
for (Node n : filledData) {
System.out.println(n.getValue());
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.