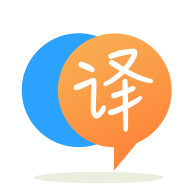
[英]Parsing internal JSON file in JSONArray in Java using org.json
[英]Parsing json file in java without using org.json
我正在嘗試從以下json數據中提取key中的第一個元素和值。 但是,我見過的大多數示例都在使用似乎已經過時的org.json? 使用以下json文件執行此操作的最佳方法是什么?
"data": [
{
"key": [
"01",
"2015"
],
"values": [
"2231439"
]
},
{
"key": [
"03",
"2015"
],
"values": [
"354164"
]
},
{
"key": [
"04",
"2015"
],
"values": [
"283712"
]
}
]
}
這就是我獲取json響應並將其存儲在String中的方式,該String從上面給出了json數據。
HttpURLConnection httpConnection = (HttpURLConnection) url.openConnection();
httpConnection.setRequestMethod("POST");
httpConnection.setDoOutput(true);
OutputStream os = httpConnection.getOutputStream();
os.write(jsonText.getBytes());
os.flush();
os.close();
int responseCode = httpConnection.getResponseCode();
System.out.println(responseCode);
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader br = new BufferedReader(new InputStreamReader(httpConnection.getInputStream()));
String input;
StringBuffer response = new StringBuffer();
while ((input = br.readLine()) != null) {
response.append(input);
}
br.close();
String responseJson = response.toString();
如果您想要替代方案,可以嘗試使用Google的gson嗎? https://github.com/google/gson
好吧,我嘗試了以下使用Jackson API的方法。 基本上,我創建了一個類,它將是整個JSON數據的Java表示形式
public class MyData {
private List<Map<String, List<String>>> data;
public List<Map<String, List<String>>> getData() {
return data;
}
public void setData(List<Map<String, List<String>>> data) {
this.data = data;
}
}
使用Jackson的API編寫了下面的解析器,但是您所描述的JSON中提到的“鍵”的值列表為String。
例如, 01和2015都是該“鍵”的列表中的項目。
請注意,我已將您的JSON數據轉儲到文件中,並且正在從其中讀取JSON。
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
try {
MyData myData = mapper.readValue(new File("data"), MyData.class);
for (Map<String, List<String>> map : myData.getData()) {
// To retrieve the first element from the list //
// map.get("key") would return a list
// in order to retrieve the first element
// wrote the below
System.out.println(map.get("key").get(0));
}
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
注意:由於您已經檢索了字符串中的JSON,請利用以下代碼
MyData myData = mapper.readValue(responseJson, MyData.class);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.