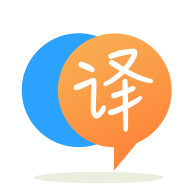
[英]What is the proper way to pass parameters between urls using react router?
[英]What's the proper way to pass dependencies between components in React?
想象一下,組件A創建了一個組件B需要顯示的項目列表。 從組件A到父組件將數據從組件A傳遞到組件B的正確方法是什么?
例如,假設組件A的構造函數創建了一個項目列表,並具有返回該列表的函數_getListItems()
。 我希望父母可以通過道具將該列表傳遞給其他組件。
我的幼稚(非工作)實現的父級試圖渲染這樣的組件:
render () {
return (
<div>
<h1>Data Test</h1>
<ComponentA ref='compa'/>
<ComponentB items={this.refs.compa._getListItems()}/>
</div>
);
}
....盡管上面的代碼不起作用,但我希望它能說明我正在嘗試做的事情。
PS。 nOOb會做出反應並使用JavaScript,因此如果我的問題的答案很明顯,請原諒我...
將您的組件分為兩個單獨的類別。
https://medium.com/@dan_abramov/smart-and-dumb-components-7ca2f9a7c7d0#.skmxo7vt4
因此,在您的情況下,數據應由ComponentA
和ComponentB
的父級創建,並通過prop將數據傳遞給ComponentA
和ComponentB
。
例:
render(){
let items = this._getListItems();
return (
<div>
<ComponentA items={items} />
<ComponentB items={items} />
</div>
);
}
在評論中重寫OP的方法:
class MyContainer extends React.Component {
constructor(props) {
super(props);
this.state = { stuff: [1,2,3] };
}
render() {
return (
<div>
<ComponentA items={this.state.stuff} />
<ComponentB items={this.state.stuff} />
</div>
);
}
}
按照上面接受的答案,我剛剛經歷了一個(相關的)EUREKA時刻,因此我將擴大答案。 當父母使用自己的狀態將道具傳遞給孩子時,只要父母的狀態發生變化,就會調用其render()函數,從而用更新后的狀態更新孩子。 因此,您可以執行以下操作:
class MyContainer extends React.Component {
constructor(props) {
super(props);
let sltd = this.props.selected
this.state = {
stuff: [1,2,3],
selected: sltd
};
}
_handleAStuff(value) {
this.setState(selected: value)
//do other stuff with selected...
}
_handleBStuff(value) {
this.setState(selected: value)
//do other stuff with selected...
}
render() {
return (
<div>
<ComponentA items={this.state.stuff} selected={this.state.selected} parentFunc={this._handleAStuff.bind(this)} />
<ComponentB items={this.state.stuff} selected={this.state.selected} parentFunc={this._handleBStuff.bind(this)} />
</div>
);
}
}
MyContainer.defaultProps = {
selected: 0
}
class ComponentA extends React.Component {
constructor(props) {
super(props)
}
_handleSelect(value) {
this.props.parentFunc(value.label)
}
render() {
const itm = this.props.items.map(function(values) {
return { value: values, label: values}
})
return (
<div>
<Select
options={itm}
value={this.props.selected}
onChange={this._handleSelect.bind(this)}
/>
</div>
);
}
}
// ComponentB...
上面的回調模式意味着ComponentA和ComponentB不需要維護狀態,它們只是“渲染內容”,這也很酷。 我開始看到REACT的力量...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.