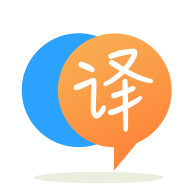
[英]accessing a 'typedef struct with char array' inside another structure
[英]Accessing a typedef structure inside another typedef struct
請參見下面給出的代碼段。 我們如何使用struct storage
訪問struct sample
的descrptn
?
typedef struct {
char hex;
char descrptn[50];
}TypeText;
typedef struct {
int val;
TypeText test[10];
}TypePara;
static const TypeText sample[]={
{0x00, "Low"},
{0x7F, "Mid"},
{0xFF, "HIgh"},
};
const TypePara storage[]= {
{0, sample},
{1, sample}
};
您的問題我們如何使用結構存儲訪問結構示例中的descrptn? 可以在下面的更正代碼的主要功能中解決該問題,但是由於對復合結構的形狀存在一些不正確的假設,因此可能看起來與您假定的情況不同。
首先,有一些語法錯誤和錯誤的假設需要解決。
1) -注意:由於要將常量表達式的元素初始化為0xFF,即使在初始化程序中將其對齊應將其標識為帶signed char
,具體取決於您的編譯器及其設置,它也可能會假定為unsigned char
並發出警告溢出。 (這正是在我的系統上發生的事情)。 因為該值實際上與帶signed char
對齊,所以在運行時不應發生溢出。
2) -您有一個struct
數組。 盡管可以使用變量來初始化簡單數組( 可變長度數組的概念自C99開始有效),但是只能使用常量值來初始化結構 。 因為您的代碼嘗試使用變量初始化結構( sample
),所以它應該無法編譯。
3) -結構體初始化程序的形狀必須與它正在初始化的結構體的形狀匹配,包括初始化程序的位置和類型。 由於TypePara是復合結構(包含結構成員的結構),因此其初始化程序必須考慮到這一點。 您的const TypePara storage[] ={...}
初始化程序的形狀不正確。
前兩個項目應在編譯時消息中為您明確標記。 確保已設置編譯器以查看它們。
不幸的是,第三項不會總是顯示為錯誤或警告。 C有時會讓您做不一定正確的事情。
以下分別以語法和注釋解決了所有這些問題。
使用struct
定義,並進行以下指示的更改,您可以像這樣訪問descrptn
:(以下內容是對原始文章的完整且可編譯的改編)
typedef struct { //Note:
char hex; //Initializer for TypeText shaped like this:
char descrptn[50]; //hex description
}TypeText; //{0x01, "one"};
typedef struct { //Note: TypePara is a struct containing an array of struct
int val; //Initializer for each instance of TypePara shaped like this:
TypeText test[10]; //val TypeText[0] TypeText[1] TypeText[9]
}TypePara; //{1, {0x01, "one"},{0x02, "two"}...{0x0A, "Ten"}};
static const TypeText sample[]={
{0x00, "Low"},
{0x49, "Mid"},
//{0xFF, "HIgh"} //commented and replaced to
{0x7F, "High"} //prevent possible overflow condition
};
//const TypePara storage[]= {
// {0, sample}, //error, "sample is not a compile time constant
// {1, sample}
//};
//Note: illustrates shape only. Values are otherwise meaningless
const TypePara storage[] = {
{ 0,{{0x00, "zero"},{0x01, "one"},{0x02, "two"},{0x03, "three"},{0x04, "four"},{0x05, "five"},{0x06, "six"},{0x07, "seven"},{0x08, "eight"},{0x09, "nine"}}},
{ 1,{{0x01, "zero"},{0x11, "one"},{0x12, "two"},{0x13, "three"},{0x14, "four"},{0x15, "five"},{0x16, "six"},{0x17, "seven"},{0x18, "eight"},{0x19, "nine"}}},
{ 2,{{0x02, "zero"},{0x21, "one"},{0x22, "two"},{0x23, "three"},{0x24, "four"},{0x25, "five"},{0x26, "six"},{0x27, "seven"},{0x28, "eight"},{0x29, "nine"}}},
{ 3,{{0x03, "zero"},{0x31, "one"},{0x32, "two"},{0x33, "three"},{0x34, "four"},{0x35, "five"},{0x36, "six"},{0x37, "seven"},{0x38, "eight"},{0x39, "nine"}}},
{ 4,{{0x04, "zero"},{0x41, "one"},{0x42, "two"},{0x43, "three"},{0x44, "four"},{0x45, "five"},{0x46, "six"},{0x47, "seven"},{0x48, "eight"},{0x49, "nine"}}}
};
int main(void)
{
//Accessing descrptn
printf( "descrptn is %s\n", storage[0].test[0].descrptn);
printf( "descrptn is %s\n", storage[0].test[1].descrptn);
printf( "descrptn is %s\n", storage[0].test[2].descrptn);
printf( "descrptn is %s\n", storage[0].test[3].descrptn);
//...
//This can continue as you have 5 storage elements
//defined, each of those containing 10 test elements.
return 0;
}
如果需要十六進制值,則應使用unsigned char
或uint_8
,而不要使用char(0xff為負數)。 此外,我想你只需要在每一個TypePara和的TypeText不是一個數組,但是這應該有助於解決您的代碼。
#include <stdio.h>
typedef struct {
unsigned char hex;
char descrptn[50];
} TypeText;
typedef struct {
int val;
TypeText *test;
} TypePara;
static TypeText sample[]={
{0x00, "Low"},
{0x7F, "Mid"},
{0xFF, "HIgh"}
};
TypePara storage[]= {
{0, &sample[0]},
{1, &sample[1]}
};
int main()
{
printf("%s\n", storage[0].test->descrptn);
return 0;
}
在storage
陣列的初始化中, sample
的類型是“指向const TypeText的指針” 。 因此,為了使初始化工作正常進行,您需要一個指向TypePara
結構中的const Typetext
的指針。 所以TypePara
結構的定義應該像這樣
typedef struct
{
int value;
const TypeText *array;
}
TypePara;
這是一個完整的示例:
#include <stdio.h>
typedef struct
{
unsigned char hex;
char description[50];
}
TypeText;
typedef struct
{
int value;
const TypeText *array;
}
TypePara;
static const TypeText sample[]=
{
{ 0x00, "Low" },
{ 0x7F, "Mid" },
{ 0xFF, "High" }
};
const TypePara storage[]=
{
{ 0, sample },
{ 1, sample }
};
int main( void )
{
for ( int i = 0; i < 2; i++ )
{
printf( "storage with value %d:\n", storage[i].value );
for ( int j = 0; j < 3; j++ )
printf( " hex=%02x description='%s'\n", storage[i].array[j].hex, storage[i].array[j].description );
printf( "\n" );
}
}
storage[index].test[index].descrptn
應該管用。
實際上,我以這種方式找到了解決方案:
typedef struct {
int age;
int RollNo;
int Rank;
char Name[10];
}TypeStudent;
typedef struct {
char class_name[20];
TypeStudent *Students;
}TypeClass;
int main()
{
const TypeStudent Stu_Details[] = {
{ 3, 1, 18, "Mahesh"},
{ 3, 1, 7, "Kumar"}
};
const TypeClass Class_Details[]= {
{ "Class 10", Stu_Details}, //two students details
{ "Class 8", 0} //no student details attached
};
printf("\r\nTest: %d",Class_Details[0].Students->Rank);
printf("\r\nTest: %d",(Class_Details[0].Students+1)->Rank);
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.