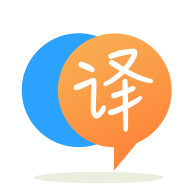
[英]What is the most efficient way to retrieve properties from a nested JavaScript object?
[英]What is the most efficient way to copy some properties from an object in JavaScript?
說,我有一個對象:
const user = {_id: 1234, firstName: 'John', lastName: 'Smith'}
我想創建另一個沒有_id
鍵的對象:
const newUser = {firstName: 'John', lastName: 'Smith'}
我正在使用這個:
const newUser = Object.assign({}, {firstName: user.firstName, lastName: user.lastName})
有沒有更好的方法來做到這一點?
您可以通過一種解構形式實現它:
const user = { _id: 1234, firstName: 'John', lastName: 'Smith' }; const { _id, ...newUser } = user; console.debug(newUser);
但是,在撰寫此答案時,spread ( ...
) 語法仍處於ECMAScript 提案階段(第 3 階段),因此在實踐中可能無法普遍使用。 您可以將它與“轉譯”層一起使用,例如 Babel。
使用Array#reduce
方法和Object.keys
方法來完成。
const user = { _id: 1234, fistName: 'John', lastName: 'Smith' }; var res = Object.keys(user).reduce(function(obj, k) { if (k != '_id') obj[k] = user[k]; return obj; }, {}); console.log(res);
您將進行兩次淺拷貝:一次使用對象文字,另一次使用Object.assign
。 所以只需使用兩者中的第一個:
const newUser = {firstName: user.firstName, lastName: user.lastName};
最有效的很可能是常規循環
const user = {_id: 1234, fistName: 'John', lastName: 'Smith'}; let obj = {}, key; for (key in user) { if ( key !== '_id' ) obj[key] = user[key]; } console.log(obj)
這個微小的功能將選擇特定的鍵來復制或從復制中排除。 排除優先:
function copy(obj, include=[], exclude=[]) { return Object.keys(obj).reduce((target, k) => { if (exclude.length) { if (exclude.indexOf(k) < 0) target[k] = obj[k]; } else if (include.indexOf(k) > -1) target[k] = obj[k]; return target; }, {}); } // let's test it const user = { _id: 1234, firstName: 'John', lastName: 'Smith' }; // both will return the same result but have different uses. console.log( 'include only firstName and lastName:\\n', copy(user, ['firstName', 'lastName']) ); console.log( 'exclude _id:\\n', copy(user, null, ['_id']) );
遍歷對象鍵,將所需的屬性鍵放入數組中,然后使用Array.prototype.includes()
僅將這些鍵復制到新對象中。
const account = { id: 123456, firstname: "John", lastname: "Doe", login: "john123", site_admin: false, blog: "https://opensource.dancingbear/", email: "john123@example.com", bio: "John ❤️ Open Source", created_at: "2001-01-01T01:30:18Z", updated_at: "2020-02-16T21:09:14Z" }; function selectSomeProperties(account) { return Object.keys(account).reduce(function(obj, k) { if (["id", "email", "created_at"].includes(k)) { obj[k] = account[k]; } return obj; }, {}); } const selectedProperties = selectSomeProperties(account); console.log(JSON.stringify(selectedProperties))
結果:
{"id":123456,"email":"john123@example.com","created_at":"2001-01-01T01:30:18Z"}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.