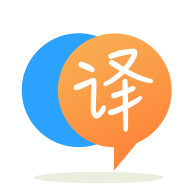
[英]Why the Media Player play media multiple times, when I switch back to app from notification?
[英]Playing media multiple times when play button clicks in notification bar
我創建了一個在服務中播放音樂的應用程序。服務運行得很好,音樂可以按預期播放。 我面臨的問題是,當我在音頻停止播放之前再次在通知中單擊“播放”按鈕時,音樂又在后台播放,這反過來又使音頻並行播放。 如果mediaplayer.isPlaying
條件似乎不起作用! 我的代碼是
public class MediaPlayerService extends Service implements MediaPlayer.OnCompletionListener {
Notification status;
private final String LOG_TAG = "NotificationService";
private MediaPlayer mediaPlayer;;
private MediaSessionManager mManager;
private MediaSession mSession;
private MediaController mController;
/** indicates how to behave if the service is killed */
int mStartMode;
/** interface for clients that bind */
IBinder mBinder;
/** indicates whether onRebind should be used */
boolean mAllowRebind;
/** Called when the service is being created. */
@Override
public void onCreate() {
mediaPlayer = new MediaPlayer();
mediaPlayer.reset();
mediaPlayer.setOnCompletionListener(this);
}
@Nullable
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
AssetFileDescriptor afd = null;
try {
afd = getAssets().openFd("mySound.mp3");
mediaPlayer.setDataSource(afd.getFileDescriptor(),afd.getStartOffset(),afd.getLength());
mediaPlayer.prepare();
if(!mediaPlayer.isPlaying()) {
Toast.makeText(this, "Not Playing", Toast.LENGTH_SHORT).show();
mediaPlayer.start();
}
else{
Toast.makeText(this, "Playing", Toast.LENGTH_SHORT).show();
mediaPlayer.pause();
}
} catch (IOException e) {
e.printStackTrace();
}
if (intent.getAction().equals(Constants.ACTION.STARTFOREGROUND_ACTION)) {
showNotification();
Toast.makeText(this, "Service Started", Toast.LENGTH_SHORT).show();
} else if (intent.getAction().equals(Constants.ACTION.PREV_ACTION)) {
Toast.makeText(this, "Clicked Previous", Toast.LENGTH_SHORT).show();
Log.i(LOG_TAG, "Clicked Previous");
} else if (intent.getAction().equals(Constants.ACTION.PLAY_ACTION)) {
if(mediaPlayer.isPlaying()) {
Toast.makeText(this, "Playing", Toast.LENGTH_SHORT).show();
}
else{
Toast.makeText(this, "Not Playing", Toast.LENGTH_SHORT).show();
}
Log.i(LOG_TAG, "Clicked Play");
} else if (intent.getAction().equals(Constants.ACTION.NEXT_ACTION)) {
Toast.makeText(this, "Clicked Next", Toast.LENGTH_SHORT).show();
Log.i(LOG_TAG, "Clicked Next");
} else if (intent.getAction().equals(
Constants.ACTION.STOPFOREGROUND_ACTION)) {
if(mediaPlayer.isPlaying()) {
mediaPlayer.stop();
}
stopForeground(true);
stopSelf();
}
return START_STICKY;
}
@Override
public void onCompletion(MediaPlayer mp) {
showNotificationClose();
Toast.makeText(this, "OVER", Toast.LENGTH_SHORT).show();
}
/** A client is binding to the service with bindService() */
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
/** Called when all clients have unbound with unbindService() */
@Override
public boolean onUnbind(Intent intent) {
return mAllowRebind;
}
/** Called when a client is binding to the service with bindService()*/
@Override
public void onRebind(Intent intent) {
}
/** Called when The service is no longer used and is being destroyed */
@Override
public void onDestroy() {
}
private void showNotification() {
// Using RemoteViews to bind custom layouts into Notification
RemoteViews views = new RemoteViews(getPackageName(),
R.layout.status_bar);
RemoteViews bigViews = new RemoteViews(getPackageName(),
R.layout.status_bar_expanded);
// showing default album image
views.setViewVisibility(R.id.status_bar_icon, View.VISIBLE);
views.setViewVisibility(R.id.status_bar_album_art, View.GONE);
bigViews.setImageViewBitmap(R.id.status_bar_album_art,
Constants.getDefaultAlbumArt(this));
Intent notificationIntent = new Intent(this, myHome.class);
notificationIntent.setAction(Constants.ACTION.MAIN_ACTION);
notificationIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK
| Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0,
notificationIntent, 0);
Intent previousIntent = new Intent(this, MediaPlayerService.class);
previousIntent.setAction(Constants.ACTION.PREV_ACTION);
PendingIntent ppreviousIntent = PendingIntent.getService(this, 0,
previousIntent, 0);
Intent playIntent = new Intent(this, MediaPlayerService.class);
playIntent.setAction(Constants.ACTION.PLAY_ACTION);
PendingIntent pplayIntent = PendingIntent.getService(this, 0,
playIntent, 0);
Intent nextIntent = new Intent(this, MediaPlayerService.class);
nextIntent.setAction(Constants.ACTION.NEXT_ACTION);
PendingIntent pnextIntent = PendingIntent.getService(this, 0,
nextIntent, 0);
Intent closeIntent = new Intent(this, MediaPlayerService.class);
closeIntent.setAction(Constants.ACTION.STOPFOREGROUND_ACTION);
PendingIntent pcloseIntent = PendingIntent.getService(this, 0,
closeIntent, 0);
views.setOnClickPendingIntent(R.id.status_bar_play, pplayIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_play, pplayIntent);
views.setOnClickPendingIntent(R.id.status_bar_next, pnextIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_next, pnextIntent);
views.setOnClickPendingIntent(R.id.status_bar_prev, ppreviousIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_prev, ppreviousIntent);
views.setOnClickPendingIntent(R.id.status_bar_collapse, pcloseIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_collapse, pcloseIntent);
views.setImageViewResource(R.id.status_bar_play,
R.drawable.apollo_holo_dark_pause);
bigViews.setImageViewResource(R.id.status_bar_play,
R.drawable.apollo_holo_dark_pause);
views.setTextViewText(R.id.status_bar_track_name, "Cheap Thrills");
bigViews.setTextViewText(R.id.status_bar_track_name, "Cheap Thrills");
views.setTextViewText(R.id.status_bar_artist_name, "Sia");
bigViews.setTextViewText(R.id.status_bar_artist_name, "Sia");
bigViews.setTextViewText(R.id.status_bar_album_name, "Cheap Thrills");
status = new Notification.Builder(this).build();
status.contentView = views;
status.bigContentView = bigViews;
status.flags = Notification.FLAG_ONGOING_EVENT;
status.icon = R.drawable.icon;
status.contentIntent = pendingIntent;
startForeground(Constants.NOTIFICATION_ID.FOREGROUND_SERVICE, status);
}
private void showNotificationClose() {
// Using RemoteViews to bind custom layouts into Notification
RemoteViews views = new RemoteViews(getPackageName(),
R.layout.status_bar);
RemoteViews bigViews = new RemoteViews(getPackageName(),
R.layout.status_bar_expanded);
// showing default album image
views.setViewVisibility(R.id.status_bar_icon, View.VISIBLE);
views.setViewVisibility(R.id.status_bar_album_art, View.GONE);
bigViews.setImageViewBitmap(R.id.status_bar_album_art,
Constants.getDefaultAlbumArt(this));
Intent notificationIntent = new Intent(this, myHome.class);
notificationIntent.setAction(Constants.ACTION.MAIN_ACTION);
notificationIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK
| Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0,
notificationIntent, 0);
Intent previousIntent = new Intent(this, MediaPlayerService.class);
previousIntent.setAction(Constants.ACTION.PREV_ACTION);
PendingIntent ppreviousIntent = PendingIntent.getService(this, 0,
previousIntent, 0);
Intent playIntent = new Intent(this, MediaPlayerService.class);
playIntent.setAction(Constants.ACTION.PLAY_ACTION);
PendingIntent pplayIntent = PendingIntent.getService(this, 0,
playIntent, 0);
Intent nextIntent = new Intent(this, MediaPlayerService.class);
nextIntent.setAction(Constants.ACTION.NEXT_ACTION);
PendingIntent pnextIntent = PendingIntent.getService(this, 0,
nextIntent, 0);
Intent closeIntent = new Intent(this, MediaPlayerService.class);
closeIntent.setAction(Constants.ACTION.STOPFOREGROUND_ACTION);
PendingIntent pcloseIntent = PendingIntent.getService(this, 0,
closeIntent, 0);
views.setOnClickPendingIntent(R.id.status_bar_play, pplayIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_play, pplayIntent);
views.setOnClickPendingIntent(R.id.status_bar_next, pnextIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_next, pnextIntent);
views.setOnClickPendingIntent(R.id.status_bar_prev, ppreviousIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_prev, ppreviousIntent);
views.setOnClickPendingIntent(R.id.status_bar_collapse, pcloseIntent);
bigViews.setOnClickPendingIntent(R.id.status_bar_collapse, pcloseIntent);
views.setImageViewResource(R.id.status_bar_play,
R.drawable.apollo_holo_dark_play);
bigViews.setImageViewResource(R.id.status_bar_play,
R.drawable.apollo_holo_dark_play);
views.setTextViewText(R.id.status_bar_track_name, "Cheap Thrills");
bigViews.setTextViewText(R.id.status_bar_track_name, "Cheap Thrills");
views.setTextViewText(R.id.status_bar_artist_name, "Sia");
bigViews.setTextViewText(R.id.status_bar_artist_name, "Sia");
bigViews.setTextViewText(R.id.status_bar_album_name, "Cheap Thrills");
status = new Notification.Builder(this).build();
status.contentView = views;
status.bigContentView = bigViews;
status.flags = Notification.FLAG_ONGOING_EVENT;
status.icon = R.drawable.icon;
status.contentIntent = pendingIntent;
startForeground(Constants.NOTIFICATION_ID.FOREGROUND_SERVICE, status);
}
}
在通知中創建Intent時,將創建一個新的服務實例,並因此創建一個新的mediaplayer實例,依此類推。
如果您希望與該服務建立連接,則應綁定該服務。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.