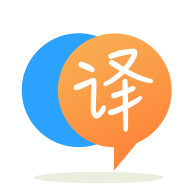
[英]Adding post fields to post data in custom WordPress REST API endpoint
[英]Enabling Custom Fields in Wordpress REST API Custom Endpoint
我為我們正在制作的應用程序的首頁制作了一個自定義REST API端點,以便它將返回基於帖子類型的3個自定義查詢,而不是為每個帖子類型發出3個不同的HTTP請求,但無法弄清楚如何獲取要顯示的每個帖子的自定義字段。 不知道下一步該去哪里:
class Home_Custom_Route extends WP_REST_Controller {
/**
* Register the routes for the objects of the controller.
*/
public function my_register_routes() {
$version = 'v2';
$namespace = 'wp/' . $version;
$base = 'home';
register_rest_route( $namespace, '/' . $base, array(
array(
'methods' => WP_REST_Server::READABLE,
'callback' => array( $this, 'get_items' ),
'permission_callback' => array( $this, 'get_items_permissions_check' ),
'args' => array(
),
),
) );
register_rest_route( $namespace, '/' . $base . '/schema', array(
'methods' => WP_REST_Server::READABLE,
'callback' => array( $this, 'get_public_item_schema' ),
) );
}
/**
* Get a collection of items
*
* @param WP_REST_Request $request Full data about the request.
* @return WP_Error|WP_REST_Response
*/
public function get_items( $request ) {
$eventargs = array(
'post_type' => 'event',
'posts_per_page' => 3,
'meta_key' => 'wpcf-event-start',
'meta_value' => current_time( 'timestamp', 1 ),
'meta_compare' => '<=',
);
$main_events = new WP_Query( $eventargs );
$listingargs = array(
'post_type' => 'listings',
'posts_per_page' => 3,
'orderby' => 'date',
'order' => 'DESC',
);
$main_listings = new WP_Query( $listingargs );
$ticketsargs = array(
'post_type' => 'product',
'posts_per_page' => 3,
'orderby' => 'date',
'order' => 'DESC',
'tax_query' => array(
array(
'taxonomy' => 'product_cat',
'field' => 'slug',
'terms' => 'tickets',
)
),
);
$main_tickets = new WP_Query( $ticketsargs );
$data = array(
'events' => $main_events->posts,
'listings' => $main_listings->posts,
'tickets' => $main_tickets->posts,
);
return new WP_REST_Response( $data, 200 );
}
/**
* Get one item from the collection
*
* @param WP_REST_Request $request Full data about the request.
* @return WP_Error|WP_REST_Response
*/
public function get_item( $request ) {
//get parameters from request
$params = $request->get_params();
$item = array();//do a query, call another class, etc
$data = $this->prepare_item_for_response( $item, $request );
//return a response or error based on some conditional
if ( 1 == 1 ) {
return new WP_REST_Response( $data, 200 );
}else{
return new WP_Error( 'code', __( 'Couldnt find it', 'xxx' ) );
}
}
/**
* Check if a given request has access to get items
*
* @param WP_REST_Request $request Full data about the request.
* @return WP_Error|bool
*/
public function get_items_permissions_check( $request ) {
return true; //<--use to make readable by all
}
/**
* Check if a given request has access to get a specific item
*
* @param WP_REST_Request $request Full data about the request.
* @return WP_Error|bool
*/
public function get_item_permissions_check( $request ) {
return $this->get_items_permissions_check( $request );
}
/**
* Prepare the item for the REST response
*
* @param mixed $item WordPress representation of the item.
* @param WP_REST_Request $request Request object.
* @return mixed
*/
public function prepare_item_for_response( $item, $request ) {
/*pretty sure this is where custom fields are enabled, but not sure how to do that*/
}
}
我發現如何通過使用get_post_meta來解決這個問題,但我的答案在每個查詢中執行多次調用,因此可能優化以首先拉取所有post meta,然后只獲取我需要的字段。
public function get_items( $request ) {
$eventargs = array(
'post_type' => 'event',
'posts_per_page' => 3,
'meta_key' => 'wpcf-event-start',
'meta_value' => current_time( 'timestamp', 1 ),
'meta_compare' => '<=',
);
$main_events = new WP_Query( $eventargs );
$events = $main_events->posts;
foreach($events as $event) {
foreach( array( 'wpcf-event-start', 'wpcf-event-end', 'wpcf-event-website' ) as $field ){
$event->$field = get_post_meta( $event->ID, $field, true );
}
}
$listingargs = array(
'post_type' => 'listings',
'posts_per_page' => 3,
'orderby' => 'date',
'order' => 'DESC',
);
$main_listings = new WP_Query( $listingargs );
$listings = $main_listings->posts;
foreach($listings as $listing) {
foreach( array( 'wpcf-listing-hours', 'wpcf-correct-address', 'wpcf-total-ratings', 'wpcf-average-rating' ) as $field ){
$listing->$field = get_post_meta( $listing->ID, $field, true );
}
}
$ticketsargs = array(
'post_type' => 'product',
'posts_per_page' => 3,
'orderby' => 'date',
'order' => 'DESC',
'tax_query' => array(
array(
'taxonomy' => 'product_cat',
'field' => 'slug',
'terms' => 'tickets',
)
),
);
$main_tickets = new WP_Query( $ticketsargs );
$tickets = $main_tickets->posts;
foreach($tickets as $ticket) {
foreach( array( '_price', '_stock', '_stock_status' ) as $field ){
$ticket->$field = get_post_meta( $ticket->ID, $field, true );
}
}
$data = array(
'events' => $events,
'listings' => $listings,
'tickets' => $tickets,
);
return new WP_REST_Response( $data, 200 );
}
帖子查詢數據不會提取自定義字段,只會提取原始帖子數據。 你必須使用get_post_meta()
但是,因為您正在創建REST API端點,所以最好執行自定義$ wpdb查詢。
<?php
$querystr = "
SELECT $wpdb->posts.*, $wpdb->postmeta.*
FROM $wpdb->posts, $wpdb->postmeta
WHERE $wpdb->posts.ID = $wpdb->postmeta.post_id
AND $wpdb->posts.post_status = 'publish'
AND $wpdb->posts.post_type = 'event'
ORDER BY $wpdb->posts.post_date DESC
";
$events = $wpdb->get_results($querystr, OBJECT);
?>
您只需將'event'替換為您要使用的任何post_type。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.