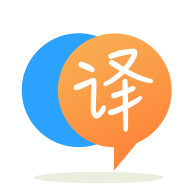
[英]How to take a specific line from a text file and read it into an array? (Java)
[英]How to take array size and elements' inputs from a text file in java?
這是給在線法官(Codeforces)的。 輸入文件是這樣的
input.txt
6
4 3 2 1 5 6
第一行是數組大小,第二行是數組元素。
我已經嘗試過使用它
public static int readFiles(String file){
try{
File f = new File(file);
Scanner Sc = new Scanner(f);
int n = Sc.nextInt();
return n;
}
catch(Exception e){
return 0;
}
}
public static int[] readFiles(String file,int n){
try{
File f = new File(file);
Scanner Sc = new Scanner(f);
Sc.nextInt();
int arr [] = new int [n];
for(int count = 0;count < n; count++){
arr[count] = Sc.nextInt();
}
return arr;
}
catch(Exception e){
return null;
}
}
public static void main (String args [] ){
int n = readFiles("input.txt");
int arr [] = readFiles("input.txt",n);
您可以調用不提供n
構建數組的方法:
public static void main (String args [] ){
int arr [] = readFiles("input.txt");
System.out.println(Arrays.toString(arr));
int n = arr.length;
System.out.println("n is: " + n);
}
public static int[] readFiles(String file){
try{
File f = new File(file);
Scanner Sc = new Scanner(f);
int n= Sc.nextInt();
int arr [] = new int [n];
for(int count = 0;count < n; count++){
arr[count] = Sc.nextInt();
}
return arr;
}
catch(Exception e){
System.out.println("The exception is: " + e);
e.printStackTrace();
return null;
}
}
如果需要n
,則可以通過arr.length
這行arr.length
。
您的代碼將永遠無法工作,因為甚至無法編譯
如果此方法readFiles(String file)
返回一個int,則這樣做沒有任何意義
int arr [] = readFiles("input.txt",n);
您為什么不在for循環上嘗試在數字之間將空格分開並將其存儲在數組中:
int temp[] = Scanner.readLine().split(" ");
您可以添加一個bufferedreader來一次讀取一行,就像這樣。
public static void main(String[] args) {
int[] numberArray = readFile();
}
public static int[] readFile(){
try(BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
int arraySize = Integer.parseInt(br.readLine());
String[] arrayContent = br.readLine().split(" ");
int[] newArray = new int[arraySize];
for(int i = 0; i<arraySize; i++){
newArray[i] = Integer.parseInt(arrayContent[i]);
}
return newArray;
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.