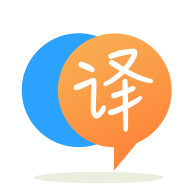
[英]How can I get angular.js checkboxes with select/unselect all functionality and indeterminate values?
[英]how can I construct .post query based on checkboxes clicked on page with Angular.js?
我有以下HTML表單:
<form name="queryForm" class="form-inline text-center">
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkOne" value="one" ng-model="formData.one" ng-true-value="'one'" ng-init="formData.one='one'">
One
</p>
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkTwo" value="two" ng-model="formData.two" ng-true-value="'two'" ng-init="formData.two='two'">
Two
</p>
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkThree" value="three" ng-model="formData.three" ng-true-value="'three'" ng-init="formData.three='three'">
Three
</p>
<button type="submit" class="btn btn-fill btn-info" style="margin-top:24px;margin-left:8px" ng-click="queryNumbers()">Refresh</button>
</form>
它在我的頁面上顯示3個復選框和一個按鈕。 我想基於用戶選擇的值構建對我的Web服務的.post
查詢。 我為此寫了一個函數:
$scope.queryUsers = function(){
var one = $scope.formData.one;
var two = $scope.formData.two;
var three = $scope.formData.three;
var params = [];
if(one){
}
if(two){
}
if(three){
}
//here I want to create a .post query
};
到目前為止,我已經准備好了我的Web服務,它采用以下格式的json:
"one":"true" //can be false
"two":"true" //can be false
"three":"true" //can be false
我想創建一個params
數組並創建后查詢-如何根據復選框的值用每個數字的true
或false
值填充此數組?
官方文檔https://docs.angularjs.org/api/ng/service/ $ http
工作的plunkr: http ://plnkr.co/edit/D7AV2DavhvNny9rppy4r?p=preview
您必須在控制器依賴區中注入“ $ http”:
myApp.controller('Ctrl1', ['$scope','$http', function($scope,$http) {
然后:JS
$scope.queryUsers = function() {
console.clear();
var one = $scope.formData.one;
var two = $scope.formData.two;
var three = $scope.formData.three;
//here I want to create a .post query
$http({
method: 'POST',
url: 'path/to/service', // put your url her
data: {
"one": one,
"two": two,
"three": three
}
}).then(function successCallback(response) {
// success
}, function errorCallback(response) {
// called asynchronously if an error occurs
// or server returns response with an error status.
});
};
html:
<form name="queryForm" class="form-inline text-center">
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkOne" value="one" ng-model="formData.one" ng-init="formData.one=false">
One
</p>
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkTwo" value="two" ng-model="formData.two" ng-init="formData.two=false">
Two
</p>
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkThree" value="three" ng-model="formData.three" ng-init="formData.three=false">
Three
</p>
<button type="submit" class="btn btn-fill btn-info" style="margin-top:24px;margin-left:8px" ng-click="queryUsers()">Refresh</button>
</form>
1)您需要創建一個關聯數組,如下所示:
var app=angular.module('myApp', []); app.controller('myCtrl', function($scope) { $scope.queryNumbers = function(){ var one = $scope.formData.one; var two = $scope.formData.two; var three = $scope.formData.three; var params = {}; params["one"]=one!='one'; params["two"]=two!='two'; params["three"]=three!='three'; console.log(params); }; })
.done-true { text-decoration: line-through; color: grey; }
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.1/angular.min.js"></script> <div ng-app="myApp"> <div ng-controller="myCtrl"> <form name="queryForm" class="form-inline text-center"> <p class="checkbox-inline onlinecheckbox"> <input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkOne" value="one" ng-model="formData.one" ng-true-value="'one'" ng-init="formData.one='one'"> One </p> <p class="checkbox-inline onlinecheckbox"> <input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkTwo" value="two" ng-model="formData.two" ng-true-value="'two'" ng-init="formData.two='two'"> Two </p> <p class="checkbox-inline onlinecheckbox"> <input type="checkbox" class="onlinecheckbox" name="optionsRadios" id="checkThree" value="three" ng-model="formData.three" ng-true-value="'three'" ng-init="formData.three='three'"> Three </p> <button type="submit" class="btn btn-fill btn-info" style="margin-top:24px;margin-left:8px" ng-click="queryNumbers()">Refresh</button> </form> </div> </div>
2)不要忘記使用JSON.stringify(object)
將數據發送到服務器;
JSON.stringify函數將Javascript對象轉換為JSON文本並將其存儲在字符串中。
在您的情況下,您需要使用以下內容:
$http({
method: 'POST',
url: 'you_method_url',
data:JSON.stringify(params)
}).then(..);
我會嘗試使事情更加通用。
首先,對於多個輸入元素使用相同的name
attr完全是不好的。
<form name="queryForm" class="form-inline text-center">
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="one" id="checkOne" value="one" ng-model="formData.one" ng-true-value="'one'" ng-init="formData.one='one'">One
</p>
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="two" id="checkTwo" value="two" ng-model="formData.two" ng-true-value="'two'" ng-init="formData.two='two'">Two
</p>
<p class="checkbox-inline onlinecheckbox">
<input type="checkbox" class="onlinecheckbox" name="three" id="checkThree" value="three" ng-model="formData.three" ng-true-value="'three'" ng-init="formData.three='three'">Three
</p>
<button type="submit" class="btn btn-fill btn-info" style="margin-top:24px;margin-left:8px" ng-click="queryNumbers()">Refresh</button>
</form>
然后,您可以在角度范圍內按他的name
訪問表單,並在不實際知道名字的情況下使用內部輸入!
var form = $scope.queryForm;
var params = [];
for (let name in form) {
if (form.hasOwnProperty(name) && typeof form[name] === 'object' && form[name].hasOwnProperty('$modelValue')) {
// build the array as you want here
params.push($scope.formData[name]);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.