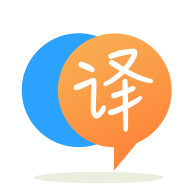
[英]Google App Engine: How to serve uploaded images in a PHP loop from google cloud storage
[英]Gae php serve images from cloud storage
我正在應用引擎應用程序中使用php開發應用程序。 這個程序是一個圖片庫。 我將圖像保存到雲存儲中,並且希望以一種動態方式檢索它們。 通常,我從我的應用程序將照片上傳到雲存儲中,但我想將其取回。 在我的本地主機中,我將我的映像名稱保存到數據庫,並與本地主機中的文件連接(典型方式)。
如何使用雲存儲執行類似的操作?如何解決此問題?
謝謝!
我用這個教程對用戶上傳到雲存儲和這一個服務於圖像。 他們工作了,照片在雲端。 現在我想創建對雲存儲的查詢以檢索它們,例如按ID,按名稱,全部,對其進行排序以及更多並將其關聯
這是我在本地主機的照片類。
class Photo extends Db_object {
protected static $db_table = "photos";
protected static $db_table_fields = array('id', 'title','caption', 'description','filename', 'alternate_text','type','size' );
public $id;
public $title;
public $caption;
public $description;
public $alternate_text;
public $filename;
public $type;
public $size;
public $tmp_path;
public $upload_directory = "images";
public $errors = array();
public $upload_errors_array = array(
UPLOAD_ERR_OK => "There is no error",
UPLOAD_ERR_INI_SIZE => "The uploaded file exceeds the upload_max_filesize directive in php.ini",
UPLOAD_ERR_FORM_SIZE => "The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the HTML form",
UPLOAD_ERR_PARTIAL => "The uploaded file was only partially uploaded.",
UPLOAD_ERR_NO_FILE => "No file was uploaded.",
UPLOAD_ERR_NO_TMP_DIR => "Missing a temporary folder.",
UPLOAD_ERR_CANT_WRITE => "Failed to write file to disk.",
UPLOAD_ERR_EXTENSION => "A PHP extension stopped the file upload."
);
// This is passing $_FILES['uploaded_file'] as an argument
public function set_file($file) {
if(empty($file) || !$file || !is_array($file)) {
$this->errors[] = "There was no file uploaded here";
return false;
}elseif($file['error'] !=0) {
$this->errors[] = $this->upload_errors_array[$file['error']];
return false;
} else {
$this->filename = basename($file['name']);
$this->tmp_path = $file['tmp_name'];
$this->type = $file['type'];
$this->size = $file['size'];
}
}
public function picture_path() {
return $this->upload_directory.DS.$this->filename;
}
public function save() {
if($this->id) {
$this->update();
} else {
if(!empty($this->errors)) {
return false;
}
if(empty($this->filename) || empty($this->tmp_path)){
$this->errors[] = "the file was not available";
return false;
}
$target_path = SITE_ROOT . DS . 'admin' . DS . $this->upload_directory . DS . $this->filename;
if(file_exists($target_path)) {
$this->errors[] = "The file {$this->filename} already exists";
return false;
}
if(move_uploaded_file($this->tmp_path, $target_path)) {
if( $this->create()) {
unset($this->tmp_path);
return true;
}
} else {
$this->errors[] = "the file directory probably does not have permission";
return false;
}
}
}
public function delete_photo() {
if($this->delete()) {
$target_path = SITE_ROOT.DS. 'admin' . DS . $this->picture_path();
return unlink($target_path) ? true : false;
} else {
return false;
}
}
public function comments() {
return Comment::find_the_comments($this->id);
}
public static function display_sidebar_data($photo_id) {
$photo = Photo::find_by_id($photo_id);
$output = "<a class='thumbnail' href='#'><img width='100' src='{$photo->picture_path()}' ></a> ";
$output .= "<p>{$photo->filename}</p>";
$output .= "<p>{$photo->type}</p>";
$output .= "<p>{$photo->size}</p>";
echo $output;
}
} // End of Class
?>
我的Db_object類在我的本地主機中,我使用它們以及正在搜索的類似內容!
<?php
class Db_object {
public $errors = array();
public $upload_errors_array = array(
UPLOAD_ERR_OK => "There is no error",
UPLOAD_ERR_INI_SIZE => "The uploaded file exceeds the upload_max_filesize directive in php.ini",
UPLOAD_ERR_FORM_SIZE => "The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the HTML form",
UPLOAD_ERR_PARTIAL => "The uploaded file was only partially uploaded.",
UPLOAD_ERR_NO_FILE => "No file was uploaded.",
UPLOAD_ERR_NO_TMP_DIR => "Missing a temporary folder.",
UPLOAD_ERR_CANT_WRITE => "Failed to write file to disk.",
UPLOAD_ERR_EXTENSION => "A PHP extension stopped the file upload."
);
public function set_file($file) {
if(empty($file) || !$file || !is_array($file)) {
$this->errors[] = "There was no file uploaded here";
return false;
}elseif($file['error'] !=0) {
$this->errors[] = $this->upload_errors_array[$file['error']];
return false;
} else {
$this->user_image = basename($file['name']);
$this->tmp_path = $file['tmp_name'];
$this->type = $file['type'];
$this->size = $file['size'];
}
}
public static function find_all() {
return static::find_by_query("SELECT * FROM " . static::$db_table . " ");
}
public static function find_by_id($id) {
global $database;
$the_result_array = static::find_by_query("SELECT * FROM " . static::$db_table . " WHERE id = $id LIMIT 1");
return !empty($the_result_array) ? array_shift($the_result_array) : false;
}
public static function find_by_query($sql) {
global $database;
$result_set = $database->query($sql);
$the_object_array = array();
while($row = mysqli_fetch_array($result_set)) {
$the_object_array[] = static::instantation($row);
}
return $the_object_array;
}
public static function instantation($the_record){
$calling_class = get_called_class();
$the_object = new $calling_class;
foreach ($the_record as $the_attribute => $value) {
if($the_object->has_the_attribute($the_attribute)) {
$the_object->$the_attribute = $value;
}
}
return $the_object;
}
private function has_the_attribute($the_attribute) {
// $object_properties = get_object_vars($this);
// return array_key_exists($the_attribute, $object_properties);
return property_exists($this, $the_attribute);
}
protected function properties() {
$properties = array();
foreach (static::$db_table_fields as $db_field) {
if(property_exists($this, $db_field)) {
$properties[$db_field] = $this->$db_field;
}
}
return $properties;
}
protected function clean_properties() {
global $database;
$clean_properties = array();
foreach ($this->properties() as $key => $value) {
$clean_properties[$key] = $database->escape_string($value);
}
return $clean_properties ;
}
public function save() {
return isset($this->id) ? $this->update() : $this->create();
}
public function create() {
global $database;
$properties = $this->clean_properties();
$sql = "INSERT INTO " . static::$db_table . "(" . implode(",", array_keys($properties)) . ")";
$sql .= "VALUES ('". implode("','", array_values($properties)) ."')";
if($database->query($sql)) {
$this->id = $database->the_insert_id();
return true;
} else {
return false;
}
} // Create Method
public function update() {
global $database;
$properties = $this->clean_properties();
$properties_pairs = array();
foreach ($properties as $key => $value) {
$properties_pairs[] = "{$key}='{$value}'";
}
$sql = "UPDATE " .static::$db_table . " SET ";
$sql .= implode(", ", $properties_pairs);
$sql .= " WHERE id= " . $database->escape_string($this->id);
$database->query($sql);
return (mysqli_affected_rows($database->connection) == 1) ? true : false;
} // end of the update method
public function delete() {
global $database;
$sql = "DELETE FROM " .static::$db_table . " ";
$sql .= "WHERE id=" . $database->escape_string($this->id);
$sql .= " LIMIT 1";
$database->query($sql);
return (mysqli_affected_rows($database->connection) == 1) ? true : false;
}
public static function count_all() {
global $database;
$sql = "SELECT COUNT(*) FROM " . static::$db_table;
$result_set = $database->query($sql);
$row = mysqli_fetch_array($result_set);
return array_shift($row);
}
public function delete_photo() {
if($this->delete()) {
$target_path = SITE_ROOT.DS. 'admin' . DS . $this->picture_path();
return unlink($target_path) ? true : false;
} else {
return false;
}
}
}
似乎您需要遵循本指南,並且需要執行SQL查詢以獲取存放在該處的數據。
希望能有所幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.