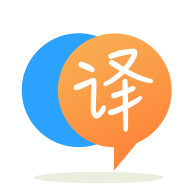
[英]How can I set another state to pass data to from the initial state in a react class component
[英]How can I set initial React state from API data?
我有一個休息 API 后端設置。 我想在getInitialState
函數中為組件設置值,但我不知道如何填充我需要返回的對象,因為我使用的是異步 http 請求。 正如預期的那樣,我返回的對象具有未定義的值。 我該如何解決這個問題?
到目前為止,我正在使用 fetch(老實說,可以切換到任何其他庫)。 我不知道如何在異步調用返回某個值之后而不是在它發生之前調用getInitialState
。
import React from 'react';
import 'whatwg-fetch';
export default class IndexPage extends React.Component {
render() {
// I basically need to call getInitialState after the last promise has been resolved
fetch('https://localhost:3000/api/aye')
.then(function(response) {
return response.json();
})
.then(function(json) {
// Need to return some values from this.
});
return (
<div>
<h1>{this.state.jsonReturnedValue}</h1>
</div>
);
}
}
提前致謝!
您應該調用this.setState
以更改state
值
export default class IndexPage extends React.Component { constructor() { super(); this.state = { jsonReturnedValue: null } } componentDidMount() { fetch('https://localhost:3000/api/aye') .then(response => response.json()) .then(json => { this.setState({ jsonReturnedValue: json }); }); } render() { return ( <div> <h1>{ this.state.jsonReturnedValue }</h1> </div> ); } }
您應該按照以下內容做一些事情。 首先,在constructor
方法中創建state
。 這將允許您通過引用{this.state.jsonReturnedValue}
在render
方法中使用此值。 如果您不知道這一點, constructor()
方法是getInitialState()
的 ES6 版本。 這是您應該設置組件state
的地方。
然后在將在render
方法之后運行的componentDidMount
,您可以使 API 調用 api 並使用 React 的setState
方法更新this.state.jsonReturnedValue
的值。
當this.setState()
運行並設置一個新值時,它將再次運行render
方法並相應地更新視圖。
export default class IndexPage extends React.Component {
constructor(){
super();
this.state = {
jsonReturnedValue: null
}
}
componentDidMount() {
this.jsonList();
}
jsonList() {
fetch('https://localhost:3000/api/aye')
.then(function(response) {
this.setState({
jsonReturnedValue: response.json()
})
})
}
render() {
return (
<div>
<h1>{this.state.jsonReturnedValue}</h1>
</div>
);
}
}
在你的情況下——
最好是第一次用空狀態數據完成渲染讓我們說
constructor(props){
super(props);
this.state = {
data : []
};
}
並在componentDidMount
進行 ajax 調用,這是您可以執行 dom 操作和發送ajax
請求以通過REST
獲取數據的地方。
從服務器獲取新數據后,使用新數據設置狀態
this.setState({data:newDataFromServer});
例如在componentDidMount
componentDidMount() {
sendAjaxRequest()
.then(
(newDataFromServer) => {
this.setState({data : newDataFromServer });
});
}
這將導致使用從服務器獲取的最新數據進行重新渲染,並且將反映新的狀態更改。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.