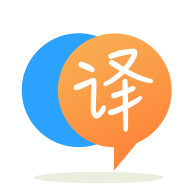
[英]Write a program in C to display the n terms of even and odd natural number and their sum using functions
[英]Write a program in C that outputs the smallest natural number greater or equal to n whose all digits are even
用C編寫一個程序,該程序將采用自然數n並輸出大於或等於n
的最小自然數,其所有數字均為十進制偶數。 例如,如果n = 16
,則應輸出20
;如果n = 41
,則應輸出42
。 我嘗試過的
#include <stdio.h>
int main(void) {
int n, i, condition = 0;
scanf("%d", &n);
for (i = n; i >= n; i++) {
if (i % 2 == 0) {
i = i / 10;
while (i > 0) {
if (i % 2 == 0) {
i = i / 10;
condition = 1;
} else {
break;
}
}
} else {
condition = 0;
}
if (condition == 1) {
printf("%d", i);
break;
}
}
return 0;
}
為什么不起作用?
您要在將i設為0(即除以10直到出現第一位數字)之后再打印i。 因此,在每種情況下都將輸出0。
相反,您應該嘗試將i的值分配給另一個變量,這樣就不必更改i。 同樣,在第一次迭代變為0后,由於for循環中要求i> = n,因此不會進行其他迭代。
#include <stdio.h>
int main(void) {
int n;
scanf("%d", &n);
for (int i = n; i >= n; i++){
int num = i;
int cond = 0;
while(num>0){
int dig = num%10;
if(dig%2 != 0){
cond = 1;
break;
}
num/=10;
}
if (cond ==0){
printf("%d",i);
break;
}
}
return 0;
}
除了@ Sanjay-sopho答案,您可以嘗試兩種方法:
這是迭代版本的樣子:
#include <stdio.h>
#include <stdlib.h>
int check_even(int n);
int
main(void) {
int i, n;
printf("Enter n: ");
if (scanf("%d", &n) != 1 || n < 1) {
printf("Invalid entry, must be a natural number!\n");
exit(EXIT_FAILURE);
}
for (i = n; i >= n; i++) {
if (check_even(i)) {
printf("%d\n", i);
break;
}
}
return 0;
}
int
check_even(int n) {
int i;
for (i = n; i > 0; i /= 10) {
if (i % 2 != 0) {
return 0;
}
}
return 1;
}
或者您可以嘗試以下遞歸函數:
int
check_even_rec(int n) {
if (n == 0) {
return 1;
} else if (n % 2 != 0) {
return 0;
}
return check_even_rec(n /= 10);
}
到目前為止,已發布的解決方案將搜索所有大於或等於n的數字,直到找到具有全偶數位的數字為止。 這是非常低效的。
更好的方法是從右到左掃描一次數字,並對其進行調整以直接獲得所需的結果:
// assume n >= 0
int next (int n)
{
int r = 0; // result
int c = 1; // coefficient (power of ten) of the current digit
// Scan digits from right to left.
while (n) {
// current digit
int d = n % 10;
if (d % 2 == 0) {
r += c * d;
}
else {
// Make sure we handle the case d == 9 correctly.
n++;
d = n % 10;
r += c * d;
// Since we are incrementing the current digit (or a more
// significant one), we must set every less significant
// digits to zero.
r -= r % c;
}
c *= 10;
n /= 10;
}
return r;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.