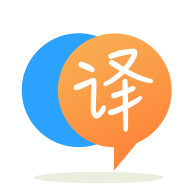
[英]How to check for digits that are the same in an integer without using arrays?
[英]How to compare the digits of 2 integer numbers in c (without arrays and strings)
我正在做一個關於猜數字的小游戲的簡單例子。
我想構建一個檢查數字並按如下所示創建兩個值的函數:
1)命中數-包含在兩個數字和兩個數字的相同位置的位數。
2)遺漏-包含在兩個數字中但不在同一位置的數字位數。
例如:
int systemNumber=1653;
int userGuess=5243;
在此示例中,兩個數字中都有數字5和3。在兩個數字中,同一位置都有數字3。 但是,在數字5 systemNumber
不一樣的地方userNumber
。 因此,我們這里有1個命中和1個未命中。
我已經用數組編寫了代碼,我想知道是否有一種方法可以在沒有數組和字符串的情況下執行此操作。
這是我的代碼。 請,如果您對我的代碼有任何改進,我想知道:)
#include <stdio.h>
#include <stdlib.h>
void checkUserCode(int num1[4], int num2[4]); // declare the function which check the guess
int hits=0, misses=0; // hits and misses in the guess
int main(void)
{
int userCode=0;
int userCodeArray[4];
int systemCodeArray[4]={1, 4, 6, 3};
int i=0;
// printing description
printf("welcome to the guessing game!\n");
printf("your goal is to guess what is the number of the system!\n");
printf("the number have 4 digits. Each digit can be between 1 to 6\nGood Luck!\n");
// input of user guess
printf("enter number: ");
scanf("%d", &userCode);
for (i=3; i>=0; i--)
{
userCodeArray[i]=userCode%10;
userCode=userCode/10;
}
checkUserCode(systemCodeArray, userCodeArray);
printf("there are %d hits and %d misess", hits, misses); // output
return 0;
}
/*
this function gets two arrays and check its elements
input (parameters): the two arrays (codes) to check
output (returning): number of hits and misses
if the element in one array also contains in the other array but not the same index: add a miss
if the element in one array also contains in the other array and they have the same index: add a hits
*/
void checkUserCode(int num1[4], int num2[4])
{
int i=0, j=0;
for (i=0; i<4; i++)
{
for (j=0; j<4; j++)
{
if(num1[i]==num2[j])
{
if (j==i)
hits++;
else
misses++;
}
}
}
}
這是我之前寫的一個示例,針對您的問題進行了調整:
我基本上使用兩個for
循環,外部循環遍歷第一個數字1653
,內部循環遍歷第二個數字5243
。 它基本上將第一個數字中的每個單獨的數字與第二個數字中的所有數字進行比較。
根據計數器的不同,它使用%10
模比較每個數字,以評估是否在相同位置匹配了相同的數字。
這是代碼:
#include <stdio.h>
#include <stdlib.h>
int
main(void) {
int num1 = 1653;
int num2 = 5243;
int pos1, pos2, hit = 0, miss = 0, i, j;
pos1 = 0;
for (i = num1; i > 0; i /= 10) {
pos2 = 0;
for (j = num2; j > 0; j /= 10) {
if (i % 10 == j % 10) {
if (pos1 == pos2) {
hit++;
} else {
miss++;
}
}
pos2++;
}
pos1++;
}
printf("hits = %d\n", hit);
printf("misses = %d\n", miss);
return 0;
}
在這種靶心游戲中,常見的方法是列表。 但是,您只能使用數字。 這是一段簡單但並非最佳的代碼,可以幫助您入門(考慮數字兩次具有相同的數字倍數!我處理得不好!或第一個數字為0?):
int n1 =...;
int n2 =...;
int count = 1;
while(n1) {
d1 = n1%10;
tmp = n2;
int count2 = 1;
while(tmp):
d2 = n2 %10;
if(d1 == d2) {
if(count == count2) hit++;
else miss++;
break;
}
count2++;
tmp/=10;
}
count++;
n1/=10;
}
我再次強調,這不是功能完整的代碼! 這是入門。 嘗試查看失敗的地方。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.