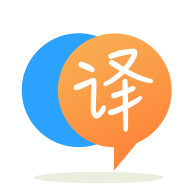
[英]Java Thread Start-Stop-Start on same button click in Eclipse
[英]Java Thread Start-Stop-Start on same button click
我正在創建一個簡單的Java程序,它具有在窗口生成器的幫助下構建的GUI。 GUI僅包含一個按鈕。
單擊按鈕后,啟動一個線程,該線程將無限次打印為隨機數,直到再次單擊同一按鈕將其停止為止。
這是我的代碼
LoopTest.java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class LoopTest extends JFrame implements ActionListener {//******
private JButton startB, stopB;
private JTextArea oa;
Start sta;
public LoopTest(){
super("Final Exam: Question ");
Container c = getContentPane();
c.setLayout(new FlowLayout());
startB = new JButton("START"); c.add(startB);
stopB = new JButton("STOP"); c.add(stopB);
oa = new JTextArea(5,20); c.add(oa);
c.add(new JScrollPane(oa));
registerEvents();
sta = new Start("Loop", oa);
}
public void registerEvents(){
startB.addActionListener(
new ActionListener(){
public void actionPerformed(ActionEvent ae){
if(startB.isEnabled() == true )
sta.setLoopFlag(true);
if(!sta.isAlive())
sta.start();
startB.setEnabled(false);
}
}
);
stopB.addActionListener(
new ActionListener(){
public void actionPerformed(ActionEvent ae){
if(stopB.isEnabled()==true){
sta.setLoopFlag(false);
}
}
}
);
}
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
}
public static void main(String[] args){
LoopTest app = new LoopTest();
app.setSize(300,300);
app.setLocationRelativeTo(null);
app.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
app.setVisible(true);
}
}
啟動.java
public class Start extends Thread {
private JTextArea ta;
private boolean loopFlag;
public Start(String name, JTextArea ta){
super(name);
this.ta = ta;
ta.setText("");
loopFlag = true;
}
public void run(){
int num=0;
while(true)
while(loopFlag){
num = 1+ (int)(Math.random()*100);
ta.append(num + "\n");
}
}
public void setLoopFlag(boolean value){
loopFlag = value;
}
}
Stop.java
public class Stop extends Thread {
public Stop( String name ){
super(name);
}
public void run(){
}
}
提前致謝。
在對Swing事件線程進行Swing組件的突變更改時,您的代碼違反了Swing線程規則。 意見建議:
repaint()
之外的Swing調用。 while (true)
是一個“緊密”循環-它里面沒有Thread.sleep
,這意味着它有可能在緊密循環中鍵入CPU,這可能會妨礙程序和計算機。 start()
和stop()
方法輕松啟動和停止此Timer。 例如:
import java.awt.BorderLayout;
import java.awt.event.*;
import javax.swing.*;
@SuppressWarnings("serial")
public class StartStop extends JPanel {
private static final int TIMER_DELAY = 300;
private StartAction startAction = new StartAction();
private StopAction stopAction = new StopAction();
private JButton button = new JButton(startAction);
private DefaultListModel<Integer> model = new DefaultListModel<>();
private JList<Integer> jList = new JList<>(model);
private Timer timer = new Timer(TIMER_DELAY, new TimerListener());
public StartStop() {
JPanel btnPanel = new JPanel();
btnPanel.add(button);
jList.setFocusable(false);
jList.setVisibleRowCount(10);
jList.setPrototypeCellValue(100000);
JScrollPane scrollPane = new JScrollPane(jList);
setLayout(new BorderLayout());
add(scrollPane, BorderLayout.CENTER);
add(btnPanel, BorderLayout.PAGE_END);
}
private class TimerListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
int num = 1 + (int) (Math.random() * 100);
model.addElement(num);
}
}
private class StartAction extends AbstractAction {
public StartAction() {
super("Start");
putValue(MNEMONIC_KEY, KeyEvent.VK_S);
}
@Override
public void actionPerformed(ActionEvent e) {
timer.start();
button.setAction(stopAction);
}
}
private class StopAction extends AbstractAction {
public StopAction() {
super("Stop");
putValue(MNEMONIC_KEY, KeyEvent.VK_S);
}
@Override
public void actionPerformed(ActionEvent e) {
timer.stop();
button.setAction(startAction);
}
}
private static void createAndShowGui() {
JFrame frame = new JFrame("Start Stop");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new StartStop());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> createAndShowGui());
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.