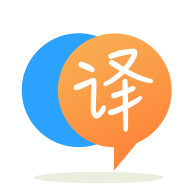
[英]Getting 'BadPaddingException: pad block corrupted' in AES/CBC/PKCS5Padding
[英]AES/ECB/PKCS5Padding how to properly pad final block?
我收到此異常:
javax.crypto.BadPaddingException: Given final block not properly padded
奇怪的是,這只會以某種不可預測的方式發生在某些字符串上,所以我不知道如何解決它。
這是完整的例外:
javax.crypto.BadPaddingException: Given final block not properly padded
at com.sun.crypto.provider.CipherCore.doFinal(CipherCore.java:966)
at com.sun.crypto.provider.CipherCore.doFinal(CipherCore.java:824)
at com.sun.crypto.provider.AESCipher.engineDoFinal(AESCipher.java:436)
at javax.crypto.Cipher.doFinal(Cipher.java:2165)
這是我的代碼:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Arrays;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class Crypt {
private Crypt() {
}
public static String encrypt(String key, String toEncrypt) {
byte[] toEncryptBytes = toEncrypt.getBytes();
try {
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, getKey(key));
byte[] encrypted = cipher.doFinal(toEncryptBytes);
return new String(encrypted);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
public static String decrypt(String key, String toDecrypt) {
try {
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, getKey(key));
byte[] decrypted = cipher.doFinal(toDecrypt.getBytes());
return new String(decrypted);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
private static SecretKeySpec getKey(String str) {
byte[] bytes = str.getBytes();
try {
MessageDigest sha = MessageDigest.getInstance("SHA-256");
bytes = sha.digest(bytes);
bytes = Arrays.copyOf(bytes, 16);
return new SecretKeySpec(bytes, "AES");
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
return null;
}
public static final void main(String[] args) {
String key = "KEY123";
String toEncrypt = "0";
boolean[] b = new boolean[128];
for (int i = 0; i < 128; i++) {
if (test(key, toEncrypt) == false) {
System.err.println("ERROR with size " + toEncrypt.length());
} else {
b[i] = true;
}
toEncrypt += "0";
}
System.out.println("Following sizes don't work:");
for (int i = 0; i < b.length; i++) {
if (!b[i]) {
System.out.println(i + 1);
}
}
}
// true = success
public static boolean test(String key, String toEncrypt) {
try {
System.out.print(toEncrypt.length() + ": ");
String encrypted = encrypt(key, toEncrypt);
System.out.print(encrypted + "; ");
String decrypted = decrypt(key, encrypted);
System.out.println(decrypted);
if (decrypted == null) {
return false;
}
if (toEncrypt.equals(decrypted)) {
return true;
}
return false;
} catch (Throwable t) {
return false;
}
}
}
運行此代碼后,我得到以下輸出:
Following sizes don't work:
3
6
10
11
15
18
22
23
27
30
34
35
39
42
46
47
51
54
58
59
63
66
70
71
75
78
82
83
87
90
94
95
99
102
106
107
111
114
118
119
123
126
但是,當我更改要加密的字符串時,這些數字也會更改。例如,當我使用“ 1”而不是“ 0”時。
我該如何工作? 如何正確填充最后一塊?
提前致謝。
您沒有將解密函數的確切輸出提供給解密函數。
加密產生一個字節數組。 但是,您可以將其轉換為String,這將以某種方式解釋字節數組(取決於平台字符集)。
保留字節數組本身,或者將字節數組轉換為十六進制String或Base64 String。 例如:
byte[] encrypted = cipher.doFinal(toEncryptBytes);
return Base64.getEncoder().encode(encrypted); // Uses Java 8 java.util.Base64
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.