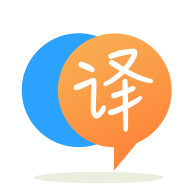
[英]React (Native): Pass a prop with the value obtained from an async function
[英]Populate React prop object from async function
我在這里的高級目標是能夠將文本文件中的數據讀取到我正在使用React創建的頁面上。
我有一個實用程序腳本文件,用於通過路徑讀取文件:
//reader.js
window.readFile = function(filePath, callback) {
var request = new XMLHttpRequest();
request.open("GET", filePath, true);
request.send(null);
request.onreadystatechange = function() {
if (request.readyState === 4 && request.status === 200) {
var type = request.getResponseHeader('Content-Type');
if (type.indexOf("text") !== 1) {
callback(null, request.responseText);
}
}
}
}
我在我的一個React組件文件中像這樣調用該函數:
//component.js
var data = {};
populateData();
function populateData() {
data.subject = "FILE TITLE";
data.title = "FILE SUBJECT";
window.readFile("../path/to/file", function(error, results){
if (!error) {
data.content = results;
}
else {
console.log(error);
}
});
}
var Content = React.createClass({
render: function() {
return (
<FileContent { ...data } />
);
}
});
我已經測試過該函數可以從此上下文正確運行,但是由於readFile函數的異步特性,看來我的數據對象的content字段永遠不會填充文件中的文本。 是否有一種同步方式返回我需要的東西,或者我該如何異步解決呢?
由於readFile
函數的異步特性,您將不得不異步解決此問題。 最簡單的方法是在您的組件中添加一個方法來調用該函數,然后在完成后設置狀態。 完成后,您可以將數據傳遞到子組件。
例如:
var Content = React.createClass({
getInitialState() {
return {
data: null
}
},
componentDidMount() {
this.populateData();
},
populateData() {
const data = {};
data.subject = "FILE TITLE";
data.title = "FILE SUBJECT";
window.readFile("../path/to/file", (error, results) => {
if (!error) {
data.content = results;
this.setState({
data
});
}
else {
console.log(error);
}
});
},
render: function() {
const {data} = this.state;
if (data === null) {
// data has not been loaded yet
// you can add a loading spinner here or some kind of loading component
return null
}
return (
<FileContent { ...data } />
);
}
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.