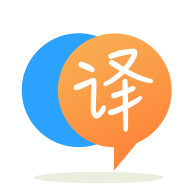
[英]How to sort and get max score for each name from 3 array of objects in JavaScript and assign it and the missing keys and values to another array?
[英]Get max values from each array of objects
所以我有這樣的數據:
data = [
[{a: "b", value: 12}, {a: "bb", value: 39}, {a: "bb", value: 150}],
[{a: "c", value: 15}, {a: "cc", value: 83}, {a: "ccc", value: 12}],
[{a: "d", value: 55}, {a: "dd", value: 9}, {a: "dd", value: 1}]
]
我想在每個對象數組中獲取最大值。 所以結果應該是這樣的:
maxValues = [150, 83, 55]
現在我的代碼是:
let maxValues = []
let tmp;
let highest = Number.NEGATIVE_INFINITY;
data.map((eachArr, index) => {
for(let i = eachArr.length -1; i >= 0; i--){
tmp = eachArr[i].value;
if(tmp > highest) highest = tmp;
}
maxValues.push(highest)
})
結果是
maxValues = [150, 150, 150]
我怎樣才能做到這一點?
您可以使用spread 語法並取映射value
的最大值。
展開語法允許在需要多個參數(用於函數調用)或多個元素(用於數組文字)或多個變量(用於解構賦值)的地方擴展表達式。
var data = [[{ a: "b", value: 12}, {a: "bb", value: 39 }, {a: "bb", value: 150 }], [{ a: "c", value: 15}, {a: "cc", value: 83 }, {a: "ccc", value: 12 }], [{ a: "d", value: 55}, {a: "dd", value: 9 }, {a: "dd", value: 1 }]], result = data.map(a => Math.max(...a.map(b => b.value))); console.log(result);
你必須包括let highest = Number.NEGATIVE_INFINITY;
在forEach
函數內部,因為您必須從array
每個集合中設置highest
。
data = [ [{a: "b", value: 12}, {a: "bb", value: 39}, {a: "bb", value: 150}], [{a: "c", value: 15}, {a: "cc", value: 83}, {a: "ccc", value: 12}], [{a: "d", value: 55}, {a: "dd", value: 9}, {a: "dd", value: 1}] ] let maxValues = [] let tmp; data.forEach((eachArr, index) => { let highest = Number.NEGATIVE_INFINITY; for(let i = eachArr.length -1; i >= 0; i--){ tmp = eachArr[i].value; if(tmp > highest) highest = tmp; } maxValues.push(highest) }) console.log(maxValues);
帶有Math.max.apply()
和Array.prototype.map()
函數的 Ecmascript 5 解決方案:
var data = [ [{a: "b", value: 12}, {a: "bb", value: 39}, {a: "bb", value: 150}], [{a: "c", value: 15}, {a: "cc", value: 83}, {a: "ccc", value: 12}], [{a: "d", value: 55}, {a: "dd", value: 9}, {a: "dd", value: 1}] ]; var maxValues = data.map(function (arr) { return Math.max.apply(null, arr.map(function(o){ return o.value; })); }); console.log(maxValues);
Math.max.apply()函數將返回給定數字中的最大值。
數字(每個嵌套對象的value
屬性)通過Array.prototype.map()函數累積。
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/max
使用Array#map
遍歷數組,生成數組元素,使用Array#reduce
找出包含最大值的對象,然后檢索其 value 屬性。
data.map(arr => arr.reduce((a, b) => a.value < b.value ? b : a).value)
data = [ [{ a: "b", value: 12 }, { a: "bb", value: 39 }, { a: "bb", value: 150 }], [{ a: "c", value: 15 }, { a: "cc", value: 83 }, { a: "ccc", value: 12 }], [{ a: "d", value: 55 }, { a: "dd", value: 9 }, { a: "dd", value: 1 }] ] console.log( data.map((arr) => arr.reduce((a, b) => a.value < b.value ? b : a).value) )
沒有ES6 箭頭函數:
data.map(function(arr){
return arr.reduce(function(a, b){
return a.value < b.value ? b : a
}).value;
});
使用下划線最大函數
沒有 ES6
_(data).map( function (item){
var max = _(item).max( function( hash ){ return hash.value })
return max.value
});
使用 ES6
_(data).map((item) => _(item).max(( hash ) => hash.value).value);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.