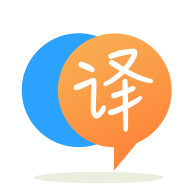
[英]Moving files from one directory to another directory in HDFS using Pyspark
[英]Moving all files from one directory to another using Python
我想使用 Python 將所有文本文件從一個文件夾移動到另一個文件夾。 我找到了這段代碼:
import os, shutil, glob
dst = '/path/to/dir/Caches/com.apple.Safari/WebKitCache/Version\ 4/Blobs '
try:
os.makedirs(/path/to/dir/Tumblr/Uploads) # create destination directory, if needed (similar to mkdir -p)
except OSError:
# The directory already existed, nothing to do
pass
for txt_file in glob.iglob('*.txt'):
shutil.copy2(txt_file, dst)
我希望它移動Blob
文件夾中的所有文件。 我沒有收到錯誤,但它也沒有移動文件。
嘗試這個:
import shutil
import os
source_dir = '/path/to/source_folder'
target_dir = '/path/to/dest_folder'
file_names = os.listdir(source_dir)
for file_name in file_names:
shutil.move(os.path.join(source_dir, file_name), target_dir)
很驚訝這沒有使用在 python 3.4
+ 中引入的 pathilib 的答案
此外,shutil 在 python 3.6
中更新以接受 pathlib 對象,此PEP-0519中的更多詳細信息
from pathlib import Path
src_path = '\tmp\files_to_move'
for each_file in Path(src_path).glob('*.*'): # grabs all files
trg_path = each_file.parent.parent # gets the parent of the folder
each_file.rename(trg_path.joinpath(each_file.name)) # moves to parent folder.
from pathlib import Path
import shutil
src_path = '\tmp\files_to_move'
trg_path = '\tmp'
for src_file in Path(src_path).glob('*.*'):
shutil.copy(src_file, trg_path)
請看一下copytree函數的實現,它:
列出目錄文件:
names = os.listdir(src)
復制文件:
for name in names:
srcname = os.path.join(src, name)
dstname = os.path.join(dst, name)
copy2(srcname, dstname)
獲取dstname不是必需的,因為如果目標參數指定一個目錄,文件將使用srcname的基本文件名復制到dst中。
將copy2替換為move 。
將“.txt”文件從一個文件夾復制到另一個文件夾非常簡單,問題包含邏輯。 只有缺失的部分用正確的信息代替,如下所示:
import os, shutil, glob
src_fldr = r"Source Folder/Directory path"; ## Edit this
dst_fldr = "Destiantion Folder/Directory path"; ## Edit this
try:
os.makedirs(dst_fldr); ## it creates the destination folder
except:
print "Folder already exist or some error";
下面的代碼行會將帶有 *.txt 擴展名的文件從 src_fldr 復制到 dst_fldr
for txt_file in glob.glob(src_fldr+"\\*.txt"):
shutil.copy2(txt_file, dst_fldr);
這應該可以解決問題。 另請閱讀 shutil 模塊的文檔以選擇適合您需要的函數(shutil.copy()、shutil.copy2()、shutil.copyfile() 或 shutil.move())。
import glob, os, shutil
source_dir = '/path/to/dir/with/files' #Path where your files are at the moment
dst = '/path/to/dir/for/new/files' #Path you want to move your files to
files = glob.iglob(os.path.join(source_dir, "*.txt"))
for file in files:
if os.path.isfile(file):
shutil.copy2(file, dst)
import shutil
import os
import logging
source = '/var/spools/asterisk/monitor'
dest1 = '/tmp/'
files = os.listdir(source)
for f in files:
shutil.move(source+f, dest1)
logging.basicConfig(filename='app.log', filemode='w', format='%(name)s
- %(levelname)s - %(message)s')
logging.info('directories moved')
帶有日志功能的一些熟代碼。 您還可以使用 crontab 將其配置為在某個時間段運行。
* */1 * * * python /home/yourprogram.py > /dev/null 2>&1
每小時運行一次! 干杯
嘗試這個:
if os.path.exists(source_dir):
try:
file_names = os.listdir(source_dir)
if not os.path.exists(target_dir):
os.makedirs(target_dir)
for file_name in file_names:
shutil.move(os.path.join(source_dir, file_name), target_dir)
except OSError as e:
print("Error: %s - %s." % (e.filename, e.strerror))
else:
log.debug(" Directory not exist {}".format(source_dir))
使用過濾器移動文件(使用 Path、os、shutil 模塊):
from pathlib import Path
import shutil
import os
src_path ='/media/shakil/New Volume/python/src'
trg_path ='/media/shakil/New Volume/python/trg'
for src_file in Path(src_path).glob('*.txt*'):
shutil.move(os.path.join(src_path,src_file),trg_path)
例如,如果我想將所有 .txt 文件從一個位置移動到另一個位置(例如在 Windows 操作系統上),我會這樣做:
import shutil
import os,glob
inpath = 'R:/demo/in'
outpath = 'R:/demo/out'
os.chdir(inpath)
for file in glob.glob("*.txt"):
shutil.move(inpath+'/'+file,outpath)
def copy_myfile_dirOne_to_dirSec(src, dest, ext):
if not os.path.exists(dest): # if dest dir is not there then we create here
os.makedirs(dest);
for item in os.listdir(src):
if item.endswith(ext):
s = os.path.join(src, item);
fd = open(s, 'r');
data = fd.read();
fd.close();
fname = str(item); #just taking file name to make this name file is destination dir
d = os.path.join(dest, fname);
fd = open(d, 'w');
fd.write(data);
fd.close();
print("Files are copyed successfully")
此腳本在目錄中查找 Docx 文件並為 Docx 文件創建文件夾並將它們移動到創建的目錄中
import os
import glob
import shutil
docfiles=glob.glob("./**/*.docx" , recursive=True)
if docfiles:
docdir = os.path.join("./DOCX")
os.makedirs(docdir , exist_ok = True)
for docfile in docfiles:
if docfile in docdir:
pass
else:
shutil.move(os.path.join(docfile),docdir)
print("Files Moved")
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.