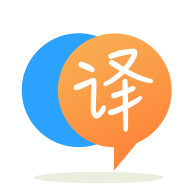
[英]Returning true or false when searching for individual characters in an array
[英]Is there a function which returns true or false when searching for an object in an array of objects?
我正在尋找一種檢查對象數組中是否存在對象的好方法。 當所有鍵/值都存在於該數組的同一對象中時,預期結果為真。
我通過瀏覽 stackoverflow 找到的答案,例如在使用jQuery.grep 的 JavaScript 對象數組中按 id 查找對象或在 Javascript 中的對象數組中查找值返回找到的對象。 我正在尋找的是一個布爾結果(不是找到的對象)。
我知道我可以循環所有數組元素,然后比較每個值......等
但我的意思是,如果有一種方法可以使用這樣的 JS 方法:
var listOfObjecs = [ {id: 1, name: "Name 1", score: 11}, {id: 2, name: "Name 2", score: 22}, {id: 3, name: "Name 3", score: 33}, {id: 4, name: "Name 4", score: 44}, {id: 5, name: "Name 5", score: 55}, ]; var isObjectExist = function(search){ return listOfObjecs.filter(function(obj){ if(obj.id===search.id && obj.name===search.name && obj.score===search.score){ return true; } return false; }); } console.log( isObjectExist({id: 3, name: "Name 3", score: 33}) ); //outputs the found object [{id: 3, name: "Name 3", score: 33}] console.log( isObjectExist({id: 9, name: "Name 3", score: 33}) ); //outputs an empty object []
這是因為:
filter() 方法創建一個新數組,其中包含通過所提供函數實現的測試的所有元素。
如果沒有原生 JavaScript 方法(返回 true 或 false),如何更新isObjectExist()
函數以返回 true 或 false?
some() 方法檢查數組中的任何元素是否通過測試(作為函數提供)。 some() 方法對數組中存在的每個元素執行一次函數:如果找到函數返回真值的數組元素, some() 返回真(並且不檢查剩余值)
我正在根據上面的評論分享一個例子,它可能會幫助其他人:
const listOfObjecs = [ { id: 1, name: "Name 1", score: 11 }, { id: 3, name: "Name 3", score: 33 }, { id: 2, name: "Name 2", score: 22 }, { id: 3, name: "Name 3", score: 33 }, { id: 4, name: "Name 4", score: 44 }, { id: 5, name: "Name 5", score: 55 }, { id: 3, name: "Name 3", score: 33 }, ]; const search1 = { id: 3, name: "Name 3", score: 33 }; const search2 = { id: 9, name: "Name 3", score: 33 }; // Using Array.prototype.some() const resSomeSearch1 = listOfObjecs.some(item => JSON.stringify(item) === JSON.stringify(search1)); console.log(`resSome(search1): ${resSomeSearch1}`); // outputs: true const resSomeSearch2 = listOfObjecs.some(item => JSON.stringify(item) === JSON.stringify(search2)); console.log(`resSome(search2): ${resSomeSearch2}`); // outputs: false // Using Array.prototype.filter() const resFilterSearch1 = !!listOfObjecs.filter(item => JSON.stringify(item) === JSON.stringify(search1)).length; console.log(`resFilter(search1): ${resFilterSearch1}`); // outputs: true const resFilterSearch2 = !!listOfObjecs.filter(item => JSON.stringify(item) === JSON.stringify(search2)).length; console.log(`resFilter(search2): ${resFilterSearch2}`); // outputs: false // Using Array.prototype.find() const resFindSearch1 = !!listOfObjecs.find(item => JSON.stringify(item) === JSON.stringify(search1)); console.log(`resFind(search1): ${resFindSearch1}`); // outputs: true const resFindSearch2 = !!listOfObjecs.find(item => JSON.stringify(item) === JSON.stringify(search2)); console.log(`resFind(search2): ${resFindSearch2}`); // outputs: false
這對我有用:
const findInArray = (myArray, item) => {
!!myArray.find((el) => el == item)
};
更新,更簡單:
['cat', 'dog', 'bat'].includes('dog');
find() 方法返回數組中滿足提供的測試函數的第一個元素的值。 否則返回 undefined。 所以沒有布爾結果。
@AlaDejbali 對他的帖子的評論。
讓我們以這個數組為例:
var arr = [{
name: "Ala",
age: 20,
},
{
name: "Jhon",
age: 22,
},
{
name: "Melissa",
age: 12,
},
];
當然, find()
返回數組中的整個對象,但這取決於您在 find 中實現的函數,例如:
function findObject(arr, value) {
if (Array.isArray(arr)) {
return arr.find((value) => {
return value.age === 20;
});
}
}
當我們像這樣調用這個函數時:
console.log(findObject(arr, 20));
結果將是:
{name: "Ala", age: 20}
據我們所知undefined
不是一個object
所以我們可以測試返回的結果:
function findObject(arr, value) {
const element = arr.find((value) => {
return value.age === 20;
});
return typeof element === "object" ? true : false;
}
或以其他方式:
function findObject(arr, value) {
const element = arr.find((value) => {
return value.age === 20;
});
return typeof element === "undefined" ? false : true;
}
如果對象存在於對象數組中,則返回布爾值。
var listOfObjecs = [ { id: 1, name: "Name 1", score: 11 }, { id: 2, name: "Name 2", score: 22 }, { id: 3, name: "Name 3", score: 33 }, { id: 4, name: "Name 4", score: 44 }, { id: 5, name: "Name 5", score: 55 }, ]; var object = { id: 3, name: "Name 3", score: 33 }; var booleanValue = listOfObjecs.filter((item) => item.id === object.id && item.name === object.name && item.score === object.score).length > 0 console.log({booleanValue})
嘗試這個:
!!arr.find(function(){...})
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.