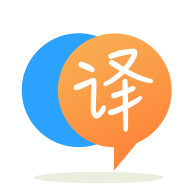
[英]How to pass data from a selected item in a listview to another activity?
[英]How to pass selected listview item to another listview in the next activity
如何將Listview選定的項目傳遞到ListView中的下一個活動中? 我通過從我的數據庫中獲取的id傳遞它,當我將所選列表傳遞到textview時它正在工作。 但我不知道如何將其傳遞給listview。 有人幫我。
這是我將ID傳遞到下一個活動的代碼
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent (this, Sample.class);
HashMap<String,String> map =(HashMap)parent.getItemAtPosition(position);
String s_id = map.get(Config.TAG_s_id).toString();
String s_name = map.get(Config.TAG_s_name).toString();
String s_gender = map.get(Config.TAG_s_gender).toString();
String teamone = map.get(Config.TAG_teamone).toString();
String teamonepts = map.get(Config.TAG_teamonepts).toString();
String teamtwo = map.get(Config.TAG_teamtwo).toString();
String teamtwopts = map.get(Config.TAG_teamtwopts).toString();
intent.putExtra(Config.S_id,s_id);
intent.putExtra(Config.S_name,s_name);
intent.putExtra(Config.S_gender,s_gender);
intent.putExtra(Config.Teamone,teamone);
intent.putExtra(Config.Teamonepts,teamonepts);
intent.putExtra(Config.Teamtwo,teamtwo);
intent.putExtra(Config.Teamtwopts,teamtwopts);
startActivity(intent);
}
}
這是我的吸氣劑下一個活動
editTextId = (EditText) findViewById(R.id.editTextId);
title1ID = (TextView) findViewById(R.id.s_genderID);
contentID = (TextView) findViewById(R.id.s_nameID);
dateID = (TextView) findViewById(R.id.teamone);
teamoneptsID = (TextView) findViewById(R.id.teamonepts);
teamtwoID = (TextView) findViewById(R.id.teamtwo);
teamtwoptsID = (TextView) findViewById(R.id.teamtwopts);
listview = (ListView) findViewById(R.id.listView);
Typeface font = Typeface.createFromAsset(getAssets(), "arial.ttf");
title1ID.setTypeface(font);
contentID.setTypeface(font);
dateID.setTypeface(font);
editTextId.setText(id);
title1ID.setText(titl);
contentID.setText(cont);
dateID.setText(date);
teamoneptsID.setText(teamonepts);
teamtwoID.setText(teamtwo);
teamtwoptsID.setText(teamtwopts);
getResult();
private void getResult() {
class GetResult extends AsyncTask<Void, Void, String> {
@Override
protected void onPreExecute() {
super.onPreExecute();
}
@Override
protected void onPostExecute(String s) {
super.onPostExecute(s);
showResult(s);
}
@Override
protected String doInBackground(Void... params) {
RequestHandler rh = new RequestHandler();
String s = rh.sendGetRequestParam(Config.URL_Sport1, id);
return s;
}
}
GetResult ge = new GetResult();
ge.execute();
}
private void showResult(String json) {
try {
JSONObject jsonObject = new JSONObject(json);
JSONArray result = jsonObject.getJSONArray(Config.TAG_JSON_ARRAY1);
JSONObject c = result.getJSONObject(0);
} catch (JSONException e) {
e.printStackTrace();
}
}
private void showResult(){
JSONObject jsonObject = null;
ArrayList<HashMap<String,String>> list = new ArrayList<>();
try {
jsonObject = new JSONObject(JSON_STRING);
JSONArray result = jsonObject.getJSONArray(Config.TAG_JSON_ARRAY2);
for(int i = 0; i<result.length(); i++){
JSONObject jo = result.getJSONObject(i);
String teamone = jo.getString(Config.TAG_teamone);
String teamonepts = jo.getString(Config.TAG_teamonepts);
String teamtwo = jo.getString(Config.TAG_teamtwo);
String teamtwopts = jo.getString(Config.TAG_teamtwopts);
String s_name = jo.getString(Config.TAG_s_name);
String s_gender = jo.getString(Config.TAG_s_gender);
HashMap<String,String> match = new HashMap<>();
match.put(Config.TAG_teamone, teamone);
match.put(Config.TAG_teamonepts,teamonepts);
match.put(Config.TAG_teamtwo,teamtwo);
match.put(Config.TAG_teamtwopts,teamtwopts);
match.put(Config.TAG_s_name,s_name);
match.put(Config.TAG_s_gender,s_gender);
list.add(match);
}
} catch (JSONException e) {
e.printStackTrace();
}
ListAdapter adapter = new SimpleAdapter(
Sample.this, list, R.layout.gamesadapterlayout,
new String[]{Config.TAG_teamone,Config.TAG_teamonepts, Config.TAG_teamtwo, Config.TAG_teamtwopts, Config.TAG_s_name, Config.TAG_s_gender},
new int[]{ R.id.team1, R.id.score1, R.id.team2, R.id.score2, R.id.Type, R.id.s_gender});
listview.setAdapter(adapter);
}
使用這樣的模態類可以有所幫助
public class Version implements Serializable{
public Version(String name, String version, String released) {
this.name = name;
this.version = version;
this.released = released;
}
private String name;
private String version;
private String released;
private String api;
private String image;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getReleased() {
return released;
}
public void setReleased(String released) {
this.released = released;
}
public String getApi() {
return api;
}
public void setApi(String api) {
this.api = api;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
}
並使用構造函數獲取並設置所有值
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
HashMap<String, String> selected = androidversions.get(position);
Version verison = new Version(selected.getString("name"),selected.getString("version"),selected.getString("released"))
Intent intent = new Intent(MainActivity.this, DetailActivity.class);
intent.putExtra("verisonDetails", verison);
startActivity(intent);
}
});
在詳細信息活動中,您可以獲得
version = (Version) getIntent().getExtras().getSerializable("verisonDetails");
在onCreate()中,並將每個值獲取為version.get ..
您可以在其他列表視圖中使用這些詳細信息,如下所示:
private void showResult(){
JSONObject jsonObject = null;
ArrayList<HashMap<String,String>> list = new ArrayList<>();
try {
jsonObject = new JSONObject(JSON_STRING);
JSONArray result = jsonObject.getJSONArray(Config.TAG_JSON_ARRAY2);
//Get details from intent that is passed from previous listview and set it to new list.
HashMap<String,String> map = new HashMap<>();
map.put(Config.TAG_teamone, teamone);
map.put(Config.TAG_teamonepts,teamonepts);
map.put(Config.TAG_teamtwo,teamtwo);
map.put(Config.TAG_teamtwopts,teamonepts);
map.put(Config.TAG_s_name,s_name);
map.put(Config.TAG_s_gender,s_gender);
list.add(map);
for(int i = 0; i<result.length(); i++){
JSONObject jo = result.getJSONObject(i);
String teamone = jo.getString(Config.TAG_teamone);
String teamonepts = jo.getString(Config.TAG_teamonepts);
String teamtwo = jo.getString(Config.TAG_teamtwo);
String teamtwopts = jo.getString(Config.TAG_teamtwopts);
String s_name = jo.getString(Config.TAG_s_name);
String s_gender = jo.getString(Config.TAG_s_gender);
HashMap<String,String> match = new HashMap<>();
match.put(Config.TAG_teamone, teamone);
match.put(Config.TAG_teamonepts,teamonepts);
match.put(Config.TAG_teamtwo,teamtwo);
match.put(Config.TAG_teamtwopts,teamtwopts);
match.put(Config.TAG_s_name,s_name);
match.put(Config.TAG_s_gender,s_gender);
list.add(match);
}
} catch (JSONException e) {
e.printStackTrace();
}
ListAdapter adapter = new SimpleAdapter(
Sample.this, list, R.layout.gamesadapterlayout,
new String[]{Config.TAG_teamone,Config.TAG_teamonepts, Config.TAG_teamtwo, Config.TAG_teamtwopts, Config.TAG_s_name, Config.TAG_s_gender},
new int[]{ R.id.team1, R.id.score1, R.id.team2, R.id.score2, R.id.Type, R.id.s_gender});
listview.setAdapter(adapter);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.