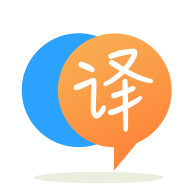
[英]invalid conversion from 'void* (*)(int*)' to 'void* (*)(void*)'
[英]Invalid conversion from `void *` to `void (*)(void*)`
因此,我目前正在使用或至少試圖編寫一個使用此C pthread線程池庫的程序。
值得注意的是thpool.h
的以下功能:
int thpool_add_work(threadpool, void (*function_p)(void*), void* arg_p);
我要添加工作的代碼如下:
int testpool(string (&input)[3]){
// Pass three strings to it. Which we will end up displaying.
cout << input[0].c_str() << endl;
cout << input[1].c_str() << endl;
cout << input[2].c_str() << endl;
return 1;
}
string input[3];
input[1] = "Hello";
input[2] = "Test";
input[3] = "Happy.";
thpool_add_work(thpool, (void*)testpool, (void*)input);
這給了我以下錯誤:
main.cpp: In function 'int main(int, char**)': main.cpp:167:55: error: invalid conversion from 'void*' to 'void (*)(void*)' [-fpermissive] thpool_add_work(thpool, (void*)testpool, (void*)input); ^ In file included from main.cpp:29:0: thpool.h:67:5: note: initializing argument 2 of 'int thpool_add_work(threadpool, void (*)(void*), void*)' int thpool_add_work(threadpool, void (*function_p)(void*), void* arg_p);
我敢肯定,我只是在調用該函數錯誤之類的東西,但無法弄清楚如何正確地正確執行該函數。 那么我該如何解決呢?
編輯/更新:
我將功能更改為執行以下操作:
void testpool(void*){
// Pass three strings to it. Which we will end up displaying.
cout << "Hellooooo." << endl;
}
這很好。 現在的問題是,如何傳遞此字符串數組,以便可以將數據作為參數訪問?
void (*function_p)(void*)
意味着您的函數必須具有返回類型void並接受單個void指針作為參數。 您的功能並非如此。
thpool_add_work
需要一個指向返回void
並接受單個void*
參數的函數的指針。 您的testpool
不是這樣的功能。 指向testpool
的指針的類型為
int (*)(string (&)[3])
這與預期的有很大的不同
void (*)(void*)
如果要將該函數與該庫一起使用,則需要對其稍作更改:
void testpool(void* vinput){
string* input = static_cast<string*>(vinput);
// Pass three strings to it. Which we will end up displaying.
cout << input[0].c_str() << endl;
cout << input[1].c_str() << endl;
cout << input[2].c_str() << endl;
}
請注意,我已經更改了參數類型,對string*
添加了強制類型轉換,並刪除了return
語句。 現在,您可以像這樣調用thpool_add_work
:
thpool_add_work(thpool, testpool, input);
如果您確實需要該返回值,則需要進一步走一步並將一個指針傳遞給某個struct:
struct TestpoolArgs
{
string input[3];
int ret;
};
void testpool(void* vargs){
TestpoolArgs* args = static_cast<TestpoolArgs*>(vargs);
// Pass three strings to it. Which we will end up displaying.
cout << args->input[0].c_str() << endl;
cout << args->input[1].c_str() << endl;
cout << args->input[2].c_str() << endl;
args->ret = 1;
}
使用此版本,您的呼叫站點將如下所示:
TestpoolArgs args;
args.input[0] = "Hello";
args.input[1] = "Test";
args.input[2] = "Happy.";
thpool_add_work(thpool, testpool, &args);
// Wait until testpool runs somehow
int testpool_return_value = args.ret;
最后一點是,在異步調用完成之前保持參數對象處於活動狀態可能是一個挑戰。 正如我在這里所做的那樣,將它們聲明為自動變量意味着您必須等待異步調用完成才能退出聲明它們的范圍,並且您實際上不能使用std::unique_ptr
或std::shared_ptr
帶C庫的std::shared_ptr
。 由於正在編寫C ++,因此最好使用std::async
類的東西。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.