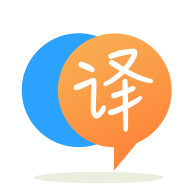
[英]Count the number of occurrences of a character in a string in Javascript
[英]count the number of occurrences of items in a list that appear in a string in javascript
我希望能夠指定一個字符/字符串列表,並找出給定字符串中列表中出現的次數。
const match = ['&', '|', '-', 'abc'];
const input = 'This & That | Or | THIS - Foo abc';
結果應該返回5
。
我可以循環匹配並使用計數器執行indexOf
,但我想知道是否有一些更簡單的方法reduce
。
您可以拆分input
字符串,過濾它,然后獲取長度,而不是遍歷match
數組。
這是一個簡單的單行:
input.split('').filter(x => match.indexOf(x) > -1).length;
片段:
const match = ['&', '|', '-']; const input = 'This & That | Or | THIS - Foo'; const count = input.split('').filter(x => match.indexOf(x) > -1).length; console.log(count); // 4
您可以使用正則表達式來查找匹配的字符:
function escape(chr) { return '\\' + chr; }
input.match(new RegExp(match.map(escape).join('|'), "g")).length
轉義是必要的,以避免出現諸如|
字符問題|
在正則表達式中具有特殊含義。
由於您要求減少:
let count = Array.prototype.reduce.call(input, (counter, chr) => (counter + (match.indexOf(chr)!==-1 ? 1: 0)), 0)
更新:
根據對其他答案的評論,OP 還希望能夠搜索除單個字符之外的“abc”等子字符串。 這是一個更優雅的解決方案,您可以將其放入本地模塊。
有兩個函數一起工作:一個轉義匹配中的特殊字符,另一個進行計數。 並再次使用減少...
// This escapes characters const escapeRegExp = (str) => ( str.replace(/[\\-\\[\\]\\/\\{\\}\\(\\)\\*\\+\\?\\.\\\\\\^\\$\\|]/g, "\\\\$&") ) // This returns the count const countMatches = (input, match, i = true) => ( match.reduce((c, pattern) => ( c + input.match(new RegExp(escapeRegExp(pattern), (i ? 'ig' : 'g'))).length ), 0) ) // OP's test case let match = ['&', '|', '-'], input = 'This & That | Or | THIS - Foo', count = countMatches(input, match) console.log('Expects 4: ', count, 4 === count) // Case Sensitive Test match = ['abc'] input = 'This ABC & That abc | Or | THIS - Foo' count = countMatches(input, match, false) console.log('Expects 1: ', count, 1 === count) // Case Insensitive Test count = countMatches(input, match) console.log('Expects 2: ', count, 2 === count)
您可以使用.map()
、 .filter()
和.filter()
.reduce()
鏈接來添加結果數組的元素
const match = ['&', '|', '-']; const input = 'This, & That | Or | THIS - Foo'; let res = match .map(v => [...input].filter(c => c === v).length) .reduce((a, b) => a + b) console.log(res);
您還可以使用for..of
循環迭代每個字符, String.prototype.contains()
,如果支持,或.some()
,以檢查字符是否在連接的字符串match
。
let n = 0;
let m = match.join("");
for (let str of input) m.contains(str) && (n += 1);
for (let str of input) match.some(s => s === str) && (n += 1);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.