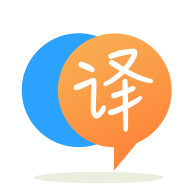
[英]convert a long string of characters to uint32_t or uint64_t in c++
[英]C++ convert string to uint64_t
我正在嘗試從字符串轉換為 uint64_t 整數。 stoi
返回一個 32 位整數,所以它在我的情況下不起作用。 還有其他解決方案嗎?
如果您使用 C++11 或更高版本,請嘗試std::stoull
。
這篇文章也可能有幫助。 我沒有將其標記為重復,因為另一個問題是關於 C。
你試過了嗎
uint64_t value;
std::istringstream iss("18446744073709551610");
iss >> value;
?
觀看現場演示
這也可能適用於過時的標准。
如果您使用的是 boost,則可以使用boost::lexical_cast
#include <iostream>
#include <string>
#include <boost-1_61/boost/lexical_cast.hpp> //I've multiple versions of boost installed, so this path may be different for you
int main()
{
using boost::lexical_cast;
using namespace std;
const string s("2424242");
uint64_t num = lexical_cast<uint64_t>(s);
cout << num << endl;
return 0;
}
現場示例: http : //coliru.stacked-crooked.com/a/c593cee68dba0d72
如果您使用的是 C++11 或更新版本,則可以使用 < cstdlib > 中的 strtoull()。 否則,如果您也需要使用 c99,您可以從 C 的stdlib.h 中使用 strtoull() 函數。
看下面的例子
#include <iostream>
#include <string>
#include <cstdlib>
int main()
{
std::string value= "14443434343434343434";
uint64_t a;
char* end;
a= strtoull( value.c_str(), &end,10 );
std::cout << "UInt64: " << a << "\n";
}
所有這些解決方案都不符合我的需要。
所以我只使用 for 循環:
uint64_t val = 0;
for (auto ch: new_str) {
if (not isdigit(ch)) return 0;
val = 10 * val + (ch - '0');
}
注意:這是 c 的解決方案,而不是 C++。 所以在 C++ 中可能更難。 這里我們將 String HEX 轉換為 uint64_t 十六進制值。 字符串的所有單個字符都被轉換為一個十六進制整數。 例如在基數 10 -> String = "123" 中:
所以就像這個邏輯用於將 HEX 字符的 String 轉換為 uint_64hex 值。
uint64_t stringToUint_64(String value) {
int stringLenght = value.length();
uint64_t uint64Value = 0x0;
for(int i = 0; i<=stringLenght-1; i++) {
char charValue = value.charAt(i);
uint64Value = 0x10 * uint64Value;
uint64Value += stringToHexInt(charValue);
}
return uint64Value;
}
int stringToHexInt(char value) {
switch(value) {
case '0':
return 0;
break;
case '1':
return 0x1;
break;
case '2':
return 0x2;
break;
case '3':
return 0x3;
break;
case '4':
return 0x4;
break;
case '5':
return 0x5;
break;
case '6':
return 0x6;
break;
case '7':
return 0x7;
break;
case '8':
return 0x8;
break;
case '9':
return 0x9;
break;
case 'A':
case 'a':
return 0xA;
break;
case 'B':
case 'b':
return 0xB;
break;
case 'C':
case 'c':
return 0xC;
break;
case 'D':
case 'd':
return 0xD;
break;
case 'E':
case 'e':
return 0xE;
break;
case 'F':
case 'f':
return 0xF;
break;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.