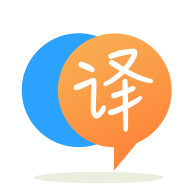
[英]What BLE characteristic should I use in a BLE IOS device for OBDII
[英]How to use BLE OBDII Peripheral
我正在嘗試創建一個可以從OBDII藍牙4(LE)設備讀取的iOS應用程序。 我購買了Vgate icar3藍牙(4.0)elm327 OBDII。 我將它插入我的汽車並找到宣傳某些服務的IOS-VLink外圍設備。 然后我可以獲得這些服務的特征。 這些是我找到的服務:
<CBService: 0x1780729c0, isPrimary = YES, UUID = Battery>
<CBService: 0x178072a80, isPrimary = YES, UUID = 1803>
<CBService: 0x178072ac0, isPrimary = YES, UUID = 1802>
<CBService: 0x178072b00, isPrimary = YES, UUID = 1811>
<CBService: 0x178072b40, isPrimary = YES, UUID = 1804>
<CBService: 0x178072b80, isPrimary = YES, UUID = 18F0>
<CBService: 0x178072bc0, isPrimary = YES, UUID = Device Information>
<CBService: 0x178072c00, isPrimary = YES, UUID = E7810A71-73AE-499D-8C15-FAA9AEF0C3F2>
但我不知道1803,1802,1811,1804和18F0服務是什么或如何使用它們。 這是我的簡單程序,可以找出所宣傳的內容。
class ViewController: UIViewController, CBCentralManagerDelegate, CBPeripheralDelegate {
var centralManager = CBCentralManager()
var peripherals = [CBPeripheral]()
@IBOutlet weak var outputTextView: UITextView!
override func viewDidLoad() {
super.viewDidLoad()
centralManager.delegate = self
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
func centralManager(_ central: CBCentralManager, didConnect peripheral: CBPeripheral) {
print("connected to \(peripheral.name ?? "unnamed")")
peripheral.delegate = self
peripheral.discoverServices(nil)
}
func centralManagerDidUpdateState(_ central: CBCentralManager) {
if central.state == .poweredOn {
central.scanForPeripherals(withServices: nil, options: nil)
}
}
func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String : Any], rssi RSSI: NSNumber) {
if peripheral.name == "IOS-Vlink" {
peripherals.append(peripheral)
print(peripheral.name ?? "peripheral has no name")
print(peripheral.description)
central.connect(peripheral, options: nil)
central.stopScan()
}
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) {
guard let services = peripheral.services else {
return
}
for service in services {
print(service.description)
peripheral.discoverCharacteristics(nil, for: service)
}
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverCharacteristicsFor service: CBService, error: Error?) {
guard let chars = service.characteristics else {
return
}
for char in chars {
print(char.description)
}
}
}
我想到了。 “E7810A71-73AE-499D-8C15-FAA9AEF0C3F2”服務具有“BEF8D6C9-9C21-4C9E-B632-BD58C1009F9F”的特征。 如果你寫一個AT命令到那個特性,那么它調用peripheral(_ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error?)
然后在value屬性中得到結果。 這是發送簡單ATZ命令的代碼。 此時它只是使用正確的OBDII ELM327命令。
class ViewController: UIViewController, CBCentralManagerDelegate, CBPeripheralDelegate {
var centralManager = CBCentralManager()
var peripherals = [CBPeripheral]()
@IBOutlet weak var outputTextView: UITextView!
override func viewDidLoad() {
super.viewDidLoad()
centralManager.delegate = self
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
func centralManager(_ central: CBCentralManager, didConnect peripheral: CBPeripheral) {
print("connected to \(peripheral.name ?? "unnamed")")
peripheral.delegate = self
peripheral.discoverServices([CBUUID(string:"E7810A71-73AE-499D-8C15-FAA9AEF0C3F2")])
}
func centralManagerDidUpdateState(_ central: CBCentralManager) {
if central.state == .poweredOn {
central.scanForPeripherals(withServices: nil, options: nil)
}
}
func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String : Any], rssi RSSI: NSNumber) {
if peripheral.name == "IOS-Vlink" {
peripherals.append(peripheral)
print(peripheral.name ?? "peripheral has no name")
print(peripheral.description)
central.connect(peripheral, options: nil)
central.stopScan()
}
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) {
guard let services = peripheral.services else {
return
}
for service in services {
peripheral.discoverCharacteristics([CBUUID(string:"BEF8D6C9-9C21-4C9E-B632-BD58C1009F9F")], for: service)
}
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverCharacteristicsFor service: CBService, error: Error?) {
guard let chars = service.characteristics else {
return
}
guard chars.count > 0 else {
return
}
let char = chars[0]
peripheral.setNotifyValue(true, for: char)
peripheral.discoverDescriptors(for: char)
print (char.properties)
peripheral.writeValue("ATZ\r\n".data(using: .utf8)!, for: char, type: CBCharacteristicWriteType.withResponse)
peripheral.readValue(for: char)
if let value = char.value {
print(String(data:value, encoding:.utf8) ?? "bad utf8 data")
}
}
func peripheral(_ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error?) {
if let value = characteristic.value {
print(String(data:value, encoding:.utf8)!)
}
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverDescriptorsFor characteristic: CBCharacteristic, error: Error?) {
print(characteristic.descriptors ?? "bad didDiscoverDescriptorsFor")
}
func peripheral(_ peripheral: CBPeripheral, didWriteValueFor characteristic: CBCharacteristic, error: Error?) {
if let error = error {
print(error)
}
print("wrote to \(characteristic)")
if let value = characteristic.value {
print(String(data:value, encoding:.utf8)!)
}
}
}
如果您在https://www.bluetooth.com/specifications/gatt/services上找到已分配的服務,則可以按照服務鏈接獲取有關特征的信息。 從服務描述中,可以遵循特征鏈接以獲取更多信息。
例如:0x1804服務用於發射功率電平。 它是一個提供org.bluetooth.characteristic.tx_power_level特性的只讀服務,如果鏈接到該特性,可以看到它提供-100到20之間的帶符號8位db級別。
另一個例子:服務0x1811用於警報通知服務。 它提供了5個獨立的特征,org.bluetooth.characteristic.supported_new_alert_category,org.bluetooth.characteristic.new_alert,org.bluetooth.characteristic.supported_unread_alert_category,org.bluetooth.characteristic.unread_alert_status和org.bluetooth.characteristic.alert_notification_control_point。 服務描述為每個特征提供簡短說明,並鏈接到該特征值的更多細節。 如果您的設備無法通過語音,短信,即時消息或電子郵件進行消息傳遞,則這些特性可能沒有意義,並且可能不受支持。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.