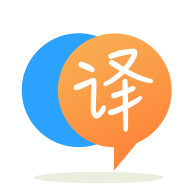
[英]Java — StringBuilder using String & not StringBuilder
[英]How to create a random phrase using java stringbuilder?
我已經編寫了簡單的隨機短語生成器。 但是我不明白如何使用stringbuilder重寫這個程序。 我試着用“追加”。 但它只是在整個字符串中添加了單詞。
我的代碼:
public static void main(String[] args){
String[] firstWord = {"one", "two","three"};
String[] secondWord = {"four", "five", "six"};
String[] thirdWord = {"seven", "eight", "nine"};
String[] fourthWord = {"ten", "eleven", "twelve"};
int oneLength = firstWord.length;
int secondLength = secondWord.length;
int thirdLength = thirdWord.length;
int fourthLength = fourthWord.length;
int rand1 = (int) (Math.random() * oneLength);
int rand2 = (int) (Math.random() * secondLength);
int rand3 = (int) (Math.random() * thirdLength);
int rand4 = (int) (Math.random() * fourthLength);
String phrase = firstWord[rand1] + " " + secondWord[rand2] + " "
+ thirdWord[rand3] + fourthWord[rand4];
System.out.println(phrase);
}
像這樣:
String phrase = new StringBuilder(firstWord[rand1]).append(" ")
.append(secondWord[rand2]).append(" ")
.append(thirdWord[rand3]).append(" ")
.append(fourthWord[rand4]).toString();
您的示例已修改為使用字符串構建器 您可以在https://www.tutorialspoint.com/compile_java8_online.php上進行測試
import java.lang.StringBuilder;
public class HelloWorld{
public static void main(String []args){
String[] firstWord = {"one", "two","three"};
String[] secondWord = {"four", "five", "six"};
String[] thirdWord = {"seven", "eight", "nine"};
int oneLength = firstWord.length;
int secondLength = secondWord.length;
int thirdLength = thirdWord.length;
int rand1 = (int) (Math.random() * oneLength);
int rand2 = (int) (Math.random() * secondLength);
int rand3 = (int) (Math.random() * thirdLength);
String phrase = firstWord[rand1] + " " + secondWord[rand2] + " "
+ thirdWord[rand3];
StringBuilder sb = new StringBuilder();
sb.append(firstWord[rand1]);
sb.append(" ");
sb.append(secondWord[rand2]);
sb.append(" ");
sb.append(thirdWord[rand3]);
String phraseSb = sb.toString();
System.out.println("Plus Operator: " + phrase);
System.out.println("String Builder: " + phraseSb);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.